如何使用C++写一个进度条模拟加载过程
时间: 2023-06-26 14:10:33 浏览: 124
可以使用C++的标准输出流来实现进度条模拟加载过程。
以下是一个简单的示例代码:
```cpp
#include <iostream>
#include <chrono>
#include <thread>
int main()
{
const int total = 100; // 加载总量
const int bar_width = 20; // 进度条宽度
for (int i = 0; i <= total; i++)
{
// 计算进度条和百分比
float progress = (float)i / total;
int bar_count = progress * bar_width;
int percent = progress * 100;
// 输出进度条
std::cout << "[";
for (int j = 0; j < bar_width; j++)
{
if (j < bar_count)
std::cout << "=";
else
std::cout << " ";
}
std::cout << "] " << percent << "%\r";
std::cout.flush();
// 模拟加载延迟
std::this_thread::sleep_for(std::chrono::milliseconds(50));
}
std::cout << std::endl;
return 0;
}
```
在此示例中,我们使用了C++11中的std::chrono和std::thread库来实现了一个简单的进度条模拟加载过程。
首先,我们定义了加载总量total和进度条宽度bar_width。然后,我们使用for循环遍历total的范围,计算出当前进度条和百分比,并输出进度条。
在每次输出进度条后,我们使用std::this_thread::sleep_for函数来模拟加载延迟。最后,我们输出一个换行符,结束程序的运行。
相关推荐
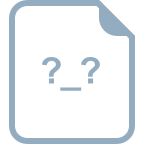
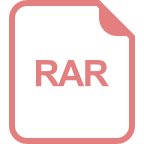
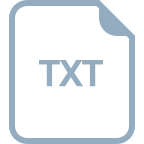














