nodejs+Vue+MySQL实现邮箱系统,登录、注册并可以查看自己发送的邮件;具体过程+代码
时间: 2023-12-24 07:05:29 浏览: 328
实现一个完整的邮箱系统需要涉及很多技术点和模块,下面是一个简单的实现过程和代码示例。
1. 准备工作
我们需要先安装 Node.js 和 MySQL 数据库,并创建一个名为 `email_system` 的数据库。在数据库中创建两张表,分别为 `users` 和 `emails`,分别存储用户信息和邮件信息。
`users` 表结构:
| Field | Type | Null | Key | Default | Extra |
| ------- | ------------ | ---- | --- | ------- | -------------- |
| id | int | NO | PRI | NULL | auto_increment |
| name | varchar(255) | NO | | NULL | |
| email | varchar(255) | NO | | NULL | |
| password| varchar(255) | NO | | NULL | |
`emails` 表结构:
| Field | Type | Null | Key | Default | Extra |
| --------- | ------------| ---- | --- | ------- | -------------- |
| id | int | NO | PRI | NULL | auto_increment |
| from_user | varchar(255) | NO | | NULL | |
| to_user | varchar(255) | NO | | NULL | |
| subject | varchar(255) | NO | | NULL | |
| content | text | NO | | NULL | |
| created_at| datetime | NO | | NULL | |
2. 后端实现
我们使用 Node.js 的 Express 框架来实现后端接口,使用 Sequelize 来操作数据库。
首先,我们需要安装依赖:
```
npm install express sequelize mysql2 body-parser cors
```
在 `index.js` 中实现后端接口:
```javascript
const express = require('express');
const bodyParser = require('body-parser');
const cors = require('cors');
const { Sequelize, DataTypes } = require('sequelize');
const app = express();
app.use(bodyParser.json());
app.use(cors());
// 创建 Sequelize 实例
const sequelize = new Sequelize('email_system', 'root', '', {
dialect: 'mysql',
host: 'localhost',
});
// 定义 User 模型
const User = sequelize.define('User', {
name: {
type: DataTypes.STRING,
allowNull: false,
},
email: {
type: DataTypes.STRING,
allowNull: false,
},
password: {
type: DataTypes.STRING,
allowNull: false,
},
}, {
tableName: 'users',
});
// 定义 Email 模型
const Email = sequelize.define('Email', {
from_user: {
type: DataTypes.STRING,
allowNull: false,
},
to_user: {
type: DataTypes.STRING,
allowNull: false,
},
subject: {
type: DataTypes.STRING,
allowNull: false,
},
content: {
type: DataTypes.TEXT,
allowNull: false,
},
created_at: {
type: DataTypes.DATE,
allowNull: false,
defaultValue: Sequelize.NOW,
},
}, {
tableName: 'emails',
});
// 创建用户
app.post('/api/users', async (req, res) => {
const { name, email, password } = req.body;
try {
const user = await User.create({ name, email, password });
res.json(user);
} catch (error) {
console.error(error);
res.status(500).json({ error: 'Failed to create user' });
}
});
// 获取用户信息
app.get('/api/users/:id', async (req, res) => {
const id = req.params.id;
try {
const user = await User.findByPk(id);
res.json(user);
} catch (error) {
console.error(error);
res.status(500).json({ error: 'Failed to get user' });
}
});
// 创建邮件
app.post('/api/emails', async (req, res) => {
const { from_user, to_user, subject, content } = req.body;
try {
const email = await Email.create({ from_user, to_user, subject, content });
res.json(email);
} catch (error) {
console.error(error);
res.status(500).json({ error: 'Failed to create email' });
}
});
// 获取用户发送的邮件
app.get('/api/emails', async (req, res) => {
const { email } = req.query;
try {
const emails = await Email.findAll({ where: { from_user: email } });
res.json(emails);
} catch (error) {
console.error(error);
res.status(500).json({ error: 'Failed to get emails' });
}
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
```
3. 前端实现
我们使用 Vue.js 来实现前端界面,使用 Axios 来发送请求。
首先,我们需要安装依赖:
```
npm install vue axios
```
在 `main.js` 中实现前端界面:
```javascript
import Vue from 'vue';
import App from './App.vue';
import axios from 'axios';
Vue.config.productionTip = false;
new Vue({
render: h => h(App),
mounted() {
// 创建用户
axios.post('http://localhost:3000/api/users', {
name: 'Alice',
email: 'alice@example.com',
password: '123456',
}).then(response => {
console.log(response.data);
}).catch(error => {
console.error(error);
});
// 获取用户信息
axios.get('http://localhost:3000/api/users/1').then(response => {
console.log(response.data);
}).catch(error => {
console.error(error);
});
// 创建邮件
axios.post('http://localhost:3000/api/emails', {
from_user: 'alice@example.com',
to_user: 'bob@example.com',
subject: 'Hello Bob',
content: 'How are you?',
}).then(response => {
console.log(response.data);
}).catch(error => {
console.error(error);
});
// 获取用户发送的邮件
axios.get('http://localhost:3000/api/emails?email=alice@example.com').then(response => {
console.log(response.data);
}).catch(error => {
console.error(error);
});
},
}).$mount('#app');
```
在 `App.vue` 中展示邮件列表:
```vue
<template>
<div>
<h1>My Emails</h1>
<ul>
<li v-for="email in emails" :key="email.id">
<strong>From:</strong> {{ email.from_user }}<br>
<strong>To:</strong> {{ email.to_user }}<br>
<strong>Subject:</strong> {{ email.subject }}<br>
<strong>Content:</strong> {{ email.content }}<br>
<strong>Created At:</strong> {{ email.created_at }}
</li>
</ul>
</div>
</template>
<script>
import axios from 'axios';
export default {
data() {
return {
emails: [],
};
},
mounted() {
const email = 'alice@example.com';
axios.get(`http://localhost:3000/api/emails?email=${email}`).then(response => {
this.emails = response.data;
}).catch(error => {
console.error(error);
});
},
};
</script>
```
以上代码仅为示例,真正的邮箱系统需要考虑更多的功能和安全性。
阅读全文
相关推荐
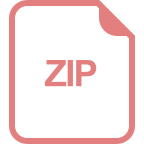
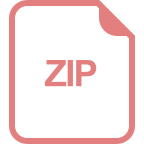
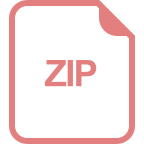




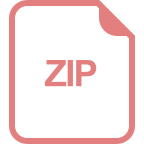
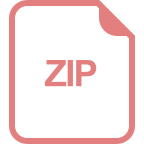
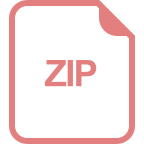
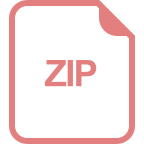
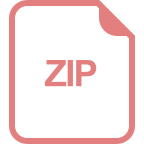
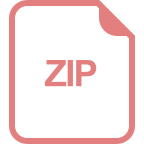
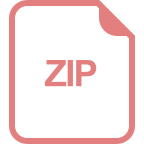
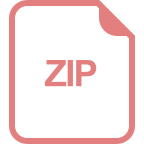
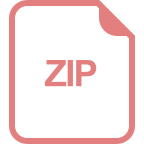
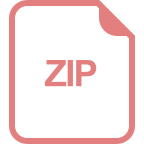
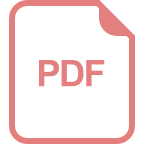

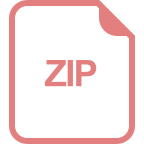