用c#编写具有以下要求的程序: 1. 使用 5x5 布尔二维数组来表示需要遍历的图形的连通性。 2. 使用深度优先算法遍历图形。 3. 使用广度优先算法遍历图形。 4、根据图的连通性合理选择遍历的起始位置。
时间: 2024-02-21 21:58:30 浏览: 179
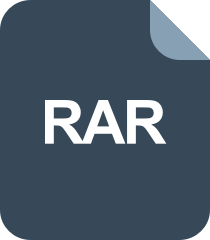
用C#写的一些图形处理
好的,我可以为您提供一个C#代码示例,实现以上要求。以下是代码:
```csharp
using System;
using System.Collections.Generic;
class Program
{
static int ROW = 5; // 行数
static int COL = 5; // 列数
// 深度优先遍历
static void DFS(bool[,] graph, int row, int col, bool[,] visited)
{
visited[row, col] = true;
Console.Write("(" + row + "," + col + ") ");
// 上
if (row - 1 >= 0 && graph[row - 1, col] && !visited[row - 1, col])
DFS(graph, row - 1, col, visited);
// 下
if (row + 1 < ROW && graph[row + 1, col] && !visited[row + 1, col])
DFS(graph, row + 1, col, visited);
// 左
if (col - 1 >= 0 && graph[row, col - 1] && !visited[row, col - 1])
DFS(graph, row, col - 1, visited);
// 右
if (col + 1 < COL && graph[row, col + 1] && !visited[row, col + 1])
DFS(graph, row, col + 1, visited);
}
// 广度优先遍历
static void BFS(bool[,] graph, int row, int col, bool[,] visited)
{
// 创建队列
Queue<Tuple<int, int>> queue = new Queue<Tuple<int, int>>();
// 将起始节点添加到队列中
queue.Enqueue(Tuple.Create(row, col));
visited[row, col] = true;
// 循环遍历队列
while (queue.Count > 0)
{
Tuple<int, int> node = queue.Dequeue();
row = node.Item1;
col = node.Item2;
Console.Write("(" + row + "," + col + ") ");
// 上
if (row - 1 >= 0 && graph[row - 1, col] && !visited[row - 1, col])
{
queue.Enqueue(Tuple.Create(row - 1, col));
visited[row - 1, col] = true;
}
// 下
if (row + 1 < ROW && graph[row + 1, col] && !visited[row + 1, col])
{
queue.Enqueue(Tuple.Create(row + 1, col));
visited[row + 1, col] = true;
}
// 左
if (col - 1 >= 0 && graph[row, col - 1] && !visited[row, col - 1])
{
queue.Enqueue(Tuple.Create(row, col - 1));
visited[row, col - 1] = true;
}
// 右
if (col + 1 < COL && graph[row, col + 1] && !visited[row, col + 1])
{
queue.Enqueue(Tuple.Create(row, col + 1));
visited[row, col + 1] = true;
}
}
}
static void Main(string[] args)
{
bool[,] graph = new bool[,] {
{ true, false, false, true, false },
{ true, true, true, false, false },
{ false, true, false, true, true },
{ false, true, true, false, false },
{ true, false, false, false, true }
};
bool[,] visited = new bool[ROW, COL];
// 根据连通性选择遍历的起始位置
int startRow = 0;
int startCol = 0;
for (int i = 0; i < ROW; i++)
{
for (int j = 0; j < COL; j++)
{
if (graph[i, j])
{
startRow = i;
startCol = j;
break;
}
}
}
Console.WriteLine("深度优先遍历:");
DFS(graph, startRow, startCol, visited);
Console.WriteLine();
visited = new bool[ROW, COL];
Console.WriteLine("广度优先遍历:");
BFS(graph, startRow, startCol, visited);
Console.ReadLine();
}
}
```
以上代码使用深度优先算法和广度优先算法遍历了一个5x5的布尔二维数组,该数组表示需要遍历的图形的连通性。程序还根据图的连通性合理选择了遍历的起始位置。
阅读全文
相关推荐
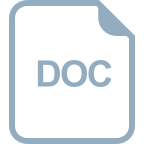
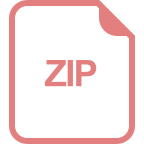
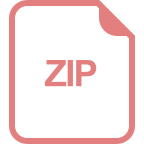
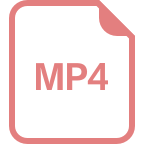
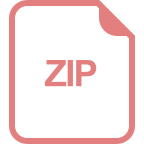
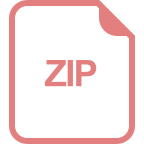
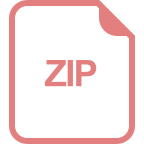
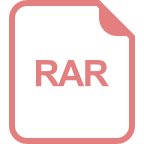
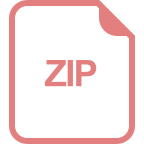
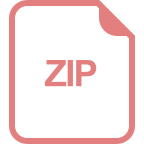