springboot 整合spring-boot-starter-data-redis 操作redis6 geo
时间: 2023-10-09 12:05:32 浏览: 78
在Spring Boot中使用Spring Data Redis进行Redis Geo操作,需要在pom.xml文件中添加以下依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
<dependency>
<groupId>redis.clients</groupId>
<artifactId>jedis</artifactId>
<version>3.6.0</version>
</dependency>
```
然后在application.properties文件中配置Redis相关信息:
```properties
# Redis
spring.redis.host=localhost
spring.redis.port=6379
spring.redis.password=
```
接下来,我们需要创建一个RedisGeoService来进行Geo操作:
```java
@Service
public class RedisGeoService {
private final RedisTemplate<String, String> redisTemplate;
public RedisGeoService(RedisTemplate<String, String> redisTemplate) {
this.redisTemplate = redisTemplate;
}
/**
* 添加地理位置信息
*
* @param key 键
* @param longitude 经度
* @param latitude 纬度
* @param member 成员
* @return Long
*/
public Long add(String key, double longitude, double latitude, String member) {
Point point = new Point(longitude, latitude);
return redisTemplate.opsForGeo().add(key, point, member);
}
/**
* 获取两个地理位置的距离
*
* @param key 键
* @param member1 成员1
* @param member2 成员2
* @param unit 距离单位
* @return Distance
*/
public Distance distance(String key, String member1, String member2, Metric unit) {
return redisTemplate.opsForGeo().distance(key, member1, member2, unit);
}
/**
* 获取指定成员的地理位置
*
* @param key 键
* @param members 成员
* @return List<Point>
*/
public List<Point> position(String key, String... members) {
return redisTemplate.opsForGeo().position(key, members);
}
/**
* 获取指定地理位置附近的成员
*
* @param key 键
* @param longitude 经度
* @param latitude 纬度
* @param radius 半径
* @param unit 距离单位
* @return GeoResults<GeoLocation<String>>
*/
public GeoResults<GeoLocation<String>> nearBy(String key, double longitude, double latitude, double radius, Metric unit) {
Circle circle = new Circle(longitude, latitude, new Distance(radius, unit));
GeoRadiusCommandArgs args = GeoRadiusCommandArgs.newGeoRadiusArgs().sortAscending();
return redisTemplate.opsForGeo().radius(key, circle, args);
}
}
```
上述代码中,我们使用了RedisTemplate来进行Redis操作。RedisTemplate是Spring Data Redis提供的核心组件,用于执行Redis命令。
在RedisGeoService中,我们定义了四个方法来进行Geo操作:
- add方法:添加地理位置信息。
- distance方法:获取两个地理位置的距离。
- position方法:获取指定成员的地理位置。
- nearBy方法:获取指定地理位置附近的成员。
使用Spring Boot和Spring Data Redis进行Geo操作非常方便,只需要定义一个RedisGeoService,并注入RedisTemplate即可。
相关推荐
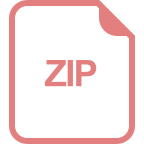
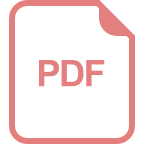
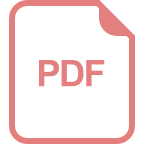
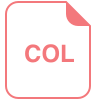













