用C语言编码实现bmp文件的压缩
时间: 2024-02-16 07:59:53 浏览: 31
好的,下面是一个简单的使用C语言实现bmp文件压缩的示例代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define BMP_HEADER_SIZE 54
typedef struct {
unsigned char r, g, b;
} RGB;
int main(int argc, char **argv) {
if (argc != 3) {
printf("Usage: %s input.bmp output.bmp\n", argv[0]);
exit(1);
}
char *input_filename = argv[1];
char *output_filename = argv[2];
FILE *input_fp = fopen(input_filename, "rb");
if (input_fp == NULL) {
printf("Failed to open input file: %s\n", input_filename);
exit(1);
}
FILE *output_fp = fopen(output_filename, "wb");
if (output_fp == NULL) {
printf("Failed to open output file: %s\n", output_filename);
fclose(input_fp);
exit(1);
}
// 读取bmp文件头
unsigned char bmp_header[BMP_HEADER_SIZE];
fread(bmp_header, 1, BMP_HEADER_SIZE, input_fp);
// 获取图像宽度和高度
int width = *(int *)(bmp_header + 18);
int height = *(int *)(bmp_header + 22);
// 计算图像数据大小
int row_size = width * 3;
int padding_size = 0;
while ((row_size + padding_size) % 4 != 0) {
padding_size++;
}
int data_size = (row_size + padding_size) * height;
// 读取图像数据
unsigned char *data = (unsigned char *)malloc(data_size);
fread(data, 1, data_size, input_fp);
// 压缩图像数据
int compressed_size = data_size / 2;
unsigned char *compressed_data = (unsigned char *)malloc(compressed_size);
int i, j;
for (i = 0, j = 0; i < data_size; i += 6, j += 3) {
RGB p1 = { data[i], data[i+1], data[i+2] };
RGB p2 = { data[i+3], data[i+4], data[i+5] };
RGB avg = { (p1.r + p2.r) / 2, (p1.g + p2.g) / 2, (p1.b + p2.b) / 2 };
compressed_data[j] = avg.r;
compressed_data[j+1] = avg.g;
compressed_data[j+2] = avg.b;
}
// 写入压缩后的bmp文件头
unsigned char compressed_bmp_header[BMP_HEADER_SIZE];
memcpy(compressed_bmp_header, bmp_header, BMP_HEADER_SIZE);
*(int *)(compressed_bmp_header + 2) = BMP_HEADER_SIZE + compressed_size;
*(int *)(compressed_bmp_header + 18) = width / 2;
*(int *)(compressed_bmp_header + 22) = height;
*(int *)(compressed_bmp_header + 34) = compressed_size;
fwrite(compressed_bmp_header, 1, BMP_HEADER_SIZE, output_fp);
// 写入压缩后的图像数据
fwrite(compressed_data, 1, compressed_size, output_fp);
fclose(input_fp);
fclose(output_fp);
free(data);
free(compressed_data);
printf("Compression completed.\n");
return 0;
}
```
这段代码实现了读取bmp文件、压缩图像数据、写入压缩后的bmp文件。具体来说,它首先读取bmp文件头,获取图像的宽度和高度,然后计算图像数据大小,读取图像数据,对图像数据进行压缩,写入压缩后的bmp文件头和压缩后的图像数据,最后释放内存并结束程序。
相关推荐
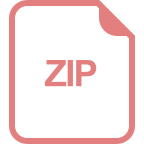














