用java设计一个学生student类,包含学号、姓名、出生日期、性别等字段 从input.txt(从附件下载)中读取其中的学生信息,并存入学生集合list中 对学生集合list进行排序,按照出生日期从早到晚排序(即年龄由大到小) 将排序后的学生集合list输出到output.txt文件中,输出格式同input.txt:一行一个学生,依次输出学号、姓名、出生日期、性别并用空格隔开
时间: 2024-02-03 13:04:00 浏览: 70
好的,我可以帮你设计一个Java版的学生类并实现排序和输出。首先,让我们来看看学生类的实现。
```java
public class Student {
private String studentId;
private String name;
private LocalDate birthDate;
private String gender;
public Student(String studentId, String name, LocalDate birthDate, String gender) {
this.studentId = studentId;
this.name = name;
this.birthDate = birthDate;
this.gender = gender;
}
// getters and setters
}
```
以上是一个简单的Java版学生类实现,包含了学号、姓名、出生日期、性别等字段。注意出生日期使用了Java 8中的`LocalDate`类。
接下来,让我们来读取input.txt文件中的学生信息,并存入学生集合list中。
```java
List<Student> students = new ArrayList<>();
try (Scanner scanner = new Scanner(new File("input.txt"))) {
while (scanner.hasNextLine()) {
String[] data = scanner.nextLine().split(" ");
LocalDate birthDate = LocalDate.parse(data[2], DateTimeFormatter.ofPattern("yyyy-MM-dd"));
Student student = new Student(data[0], data[1], birthDate, data[3]);
students.add(student);
}
} catch (FileNotFoundException e) {
e.printStackTrace();
}
```
以上代码将会从input.txt文件中读取每一行学生信息,并将其存入一个学生对象中,最后添加到学生集合list中。注意出生日期的处理。
接下来,我们需要对学生集合list进行排序,按照出生日期从早到晚排序(即年龄由大到小)。
```java
students.sort(Comparator.comparing(Student::getBirthDate));
```
以上代码将会对学生集合list进行排序,按照出生日期从早到晚排序(即年龄由大到小)。
最后,我们需要将排序后的学生集合list输出到output.txt文件中,输出格式同input.txt:一行一个学生,依次输出学号、姓名、出生日期、性别并用空格隔开。
```java
try (PrintWriter writer = new PrintWriter(new File("output.txt"))) {
for (Student student : students) {
writer.printf("%s %s %s %s%n", student.getStudentId(), student.getName(),
student.getBirthDate().format(DateTimeFormatter.ofPattern("yyyy-MM-dd")), student.getGender());
}
} catch (FileNotFoundException e) {
e.printStackTrace();
}
```
以上代码将会将排序后的学生集合list输出到output.txt文件中,输出格式同input.txt:一行一个学生,依次输出学号、姓名、出生日期、性别并用空格隔开。
完整代码如下:
```java
import java.io.File;
import java.io.FileNotFoundException;
import java.io.PrintWriter;
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
import java.util.ArrayList;
import java.util.Comparator;
import java.util.List;
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
List<Student> students = new ArrayList<>();
try (Scanner scanner = new Scanner(new File("input.txt"))) {
while (scanner.hasNextLine()) {
String[] data = scanner.nextLine().split(" ");
LocalDate birthDate = LocalDate.parse(data[2], DateTimeFormatter.ofPattern("yyyy-MM-dd"));
Student student = new Student(data[0], data[1], birthDate, data[3]);
students.add(student);
}
} catch (FileNotFoundException e) {
e.printStackTrace();
}
students.sort(Comparator.comparing(Student::getBirthDate));
try (PrintWriter writer = new PrintWriter(new File("output.txt"))) {
for (Student student : students) {
writer.printf("%s %s %s %s%n", student.getStudentId(), student.getName(),
student.getBirthDate().format(DateTimeFormatter.ofPattern("yyyy-MM-dd")), student.getGender());
}
} catch (FileNotFoundException e) {
e.printStackTrace();
}
}
}
```
希望能够帮助到你!
阅读全文
相关推荐
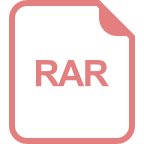
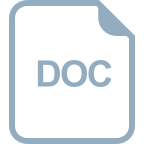
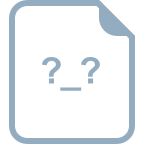







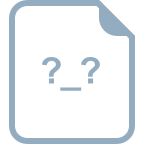
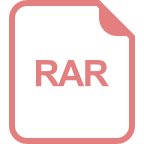
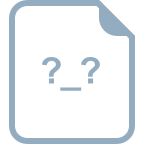
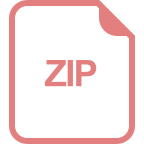
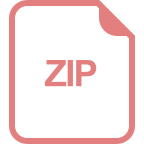