修改这段代码更加简洁 private async Task<string> get() { string a = textBox2.Text; var requestBody = ""; var requestContent = new StringContent(requestBody, Encoding.UTF8, "application/json"); var httpClient = new HttpClient(); var response = await httpClient.PostAsync("http://localhost:63300/api/Pms/GetLocationProperty?WareHouseCode=上料", requestContent); var responseContent = await response.Content.ReadAsStringAsync(); //Console.WriteLine(responseContent); return responseContent; }
时间: 2024-02-14 09:09:42 浏览: 27
好的,下面是一种更加简洁的实现方式:
```
private async Task<string> GetLocationProperty(string wareHouseCode = "")
{
using (var httpClient = new HttpClient())
{
var response = await httpClient.GetAsync($"http://localhost:63300/api/Pms/GetLocationProperty?WareHouseCode={wareHouseCode}");
return await response.Content.ReadAsStringAsync();
}
}
```
这段代码定义了一个名为 GetLocationProperty 的异步方法,该方法接受一个可选的参数 wareHouseCode 表示仓库编码。该方法使用 using 语句创建一个 HttpClient 对象,并使用 GetAsync 方法发送一个 HTTP GET 请求到指定的接口地址,同时将 WareHouseCode 参数作为查询字符串传递给接口。最后,该方法使用 ReadAsStringAsync 方法读取响应内容,并将其作为异步任务的返回值。
相关问题
private async Task<string> get() { string a = textBox2.Text; var requestBody = ""; var requestContent = new StringContent(requestBody, Encoding.UTF8, "application/json"); var httpClient = new HttpClient(); var response = await httpClient.PostAsync("http://localhost:63300/api/Pms/GetLocationProperty?WareHouseCode=上料", requestContent); var responseContent = await response.Content.ReadAsStringAsync(); //Console.WriteLine(responseContent); return responseContent; }
这是一个使用 C# 编写的异步方法,用于发送 HTTP POST 请求到 http://localhost:63300/api/Pms/GetLocationProperty 接口,并且指定 WareHouseCode 参数为“上料”。该方法的返回值是一个字符串类型的异步任务,表示接口返回的数据。
具体来说,该方法首先获取了一个名为“a”的字符串变量,然后使用空的请求体和 UTF-8 编码的请求内容创建了一个名为“requestContent”的 StringContent 对象。接下来,该方法使用 HttpClient 类创建了一个名为“httpClient”的 HTTP 客户端,并且使用 PostAsync 方法发送了一个 HTTP POST 请求到指定的接口地址。最后,该方法使用 ReadAsStringAsync 方法读取了响应内容,并将其作为异步任务的返回值。
用按钮调用这个方法 private async Task<string> Task() { }
如果你想用按钮调用这个方法,可以在按钮点击事件的处理函数中调用该方法。例如,你可以在 Windows 窗体中创建一个按钮,然后在其 Click 事件处理函数中调用该方法,如下所示:
```csharp
private async void btnTask_Click(object sender, EventArgs e)
{
var result = await Task(); // 调用 Task 方法并等待返回结果
// 处理返回结果
}
```
需要注意的是,因为 Task 方法是异步方法,所以在调用它时需要使用 `await` 关键字来等待其执行完成。在等待的过程中,程序会继续执行其他任务,不会被阻塞。当 Task 方法执行完成后,会返回一个字符串类型的结果,此时可以对返回结果进行处理。
相关推荐
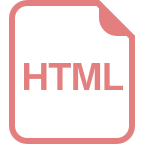
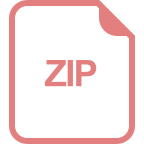













