编写一个程序,读取输入,直至读到EOF,统计读入的单词数、大写字母数、小写字母数、标点符号数和数字字符数。使用ctype.h头文件中的函数
时间: 2024-02-18 20:59:38 浏览: 74
以下是一个读取输入并统计各种字符数量的程序示例:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
int c;
int word_count = 0;
int upper_count = 0;
int lower_count = 0;
int punct_count = 0;
int digit_count = 0;
int in_word = 0;
while ((c = getchar()) != EOF) {
if (isalpha(c)) {
if (!in_word) {
in_word = 1;
++word_count;
}
if (isupper(c)) {
++upper_count;
} else {
++lower_count;
}
} else if (ispunct(c)) {
++punct_count;
in_word = 0;
} else if (isdigit(c)) {
++digit_count;
in_word = 0;
} else {
in_word = 0;
}
}
printf("Word count: %d\n", word_count);
printf("Uppercase count: %d\n", upper_count);
printf("Lowercase count: %d\n", lower_count);
printf("Punctuation count: %d\n", punct_count);
printf("Digit count: %d\n", digit_count);
return 0;
}
```
程序使用getchar函数逐个读取输入字符,使用isalpha、ispunct和isdigit函数判断字符类型,并统计数量。in_word变量表示当前是否在单词中。如果读入的字符是字母,则判断是否在单词中,如果不在,则单词数加1;如果在,则统计大写字母和小写字母数量。如果读入的字符是标点符号或数字,则单词结束,in_word变量设置为0。最后,输出各种字符的数量统计结果。
阅读全文
相关推荐
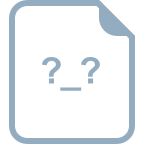

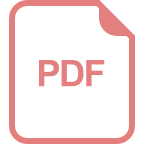
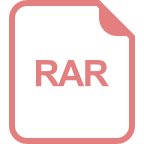
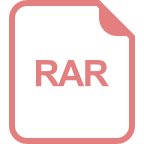
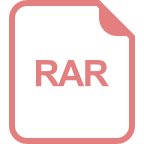
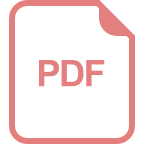
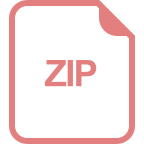
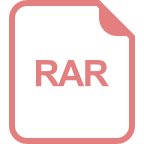
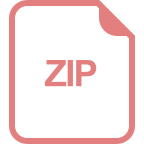
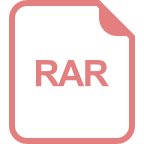
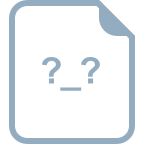
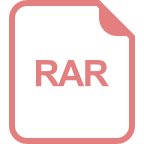