import { Icon } from '@ali/deep'; import React, { FC, useState } from 'react'; import styles from './style.module.css'; interface IDayParams { minAdvanceTime: number; maxAdvanceTime: number; bookDate: number; } const DateSelector: FC<IDayParams> = ({ bookDate, minAdvanceTime, maxAdvanceTime }) => { console.log('maxAdvanceTime',maxAdvanceTime) const [date, setDate] = useState(new Date(bookDate)); const handlePrevClick = () => { const newDate = new Date(date.getTime() - 15 * 24 * 60 * 60 * 1000); const today = new Date(); if (newDate < today) { setDate(today); } else { setDate(newDate); } }; const handleNextClick = () => { const newDate = new Date(date.getTime() + 15 * 24 * 60 * 60 * 1000); setDate(newDate); }; const formatDate = (date) => { const month = (date.getMonth() + 1).toString().padStart(2, '0'); const day = date.getDate().toString().padStart(2, '0'); return `${month}/${day}日`; }; const renderDateRange = () => { const start = new Date(date.getTime() - 0 * 24 * 60 * 60 * 1000); const end = new Date(date.getTime() + 14 * 24 * 60 * 60 * 1000); const firstDay = new Date(start); const lastDay = new Date(end); return ( <div className={styles.dates}> <div key={firstDay.getTime()} className={styles.date}> {formatDate(firstDay)} </div> <span>-</span> <div key={lastDay.getTime()} className={styles.date}> {formatDate(lastDay)} </div> </div> ); }; return ( <div className={styles.choose}> <div className={styles.content}> <Icon type="arrow-double-left" onClick={handlePrevClick} className={styles.icon} /> <div>{renderDateRange()}</div> <Icon type="arrow-double-right" onClick={handleNextClick} className={styles.icon} /> </div> </div> ); }; export default DateSelector;不如最大时间是15天,就不能往下点了,应该值写
时间: 2023-12-30 12:02:33 浏览: 25
可以在 `handleNextClick` 函数中加入判断逻辑,如果 `newDate` 超过了 `maxAdvanceTime`,则不更新日期。例如:
```
const handleNextClick = () => {
const newDate = new Date(date.getTime() + 15 * 24 * 60 * 60 * 1000);
if (newDate > new Date(maxAdvanceTime)) {
return;
}
setDate(newDate);
};
```
这样就可以实现最大时间为15天的限制了。
相关问题
import classes from './Header.module.css'
`import classes from './Header.module.css'` 这段代码是在 React 组件中使用 CSS modules 的语法,用于导入 `Header.module.css` 文件,并把其中定义的 CSS 类名映射到一个 JavaScript 对象中,以便在组件中使用。
在使用 CSS modules 时,通常会将样式文件的后缀名改为 `.module.css`,例如 `Header.module.css`。这样做可以使样式表在被导入时,自动启用局部作用域,避免全局 CSS 类名的冲突,并提供更好的样式隔离性。
当执行 `import classes from './Header.module.css'` 后,`classes` 对象将包含 `Header.module.css` 中定义的所有 CSS 类名。例如,如果 `Header.module.css` 中定义了一个名为 `header` 的 CSS 类,那么可以通过 `classes.header` 来访问这个类名,并将其应用到组件的 DOM 元素上,例如:
```jsx
import React from 'react';
import classes from './Header.module.css';
function Header() {
return (
<header className={classes.header}>
<h1>Welcome to my website</h1>
</header>
);
}
export default Header;
```
这样,当 `Header` 组件被渲染时,它的 `header` 元素会自动添加一个本地化的 CSS 类名,例如 `_Header_module_css__header__3g0L6`,从而保证样式的唯一性和隔离性。
import styles from './App.less'报错Cannot read properties of undefined
### 回答1:
这个错误通常是因为在使用 `import` 导入样式时,没有正确配置 webpack 或者 babel 的相关插件,导致样式文件无法被正确解析。可以尝试以下几种方法来解决:
1. 确保项目中已经安装了 `less` 和 `less-loader`,并且在 webpack 配置中正确配置了 less-loader。
2. 确保项目中已经安装了 `babel-plugin-import`,并且在 `.babelrc` 中进行了配置。例如:
```
{
"plugins": [
["import", { "libraryName": "antd", "style": true }]
]
}
```
3. 如果你使用的是 create-react-app 创建的项目,可以尝试使用 `react-app-rewired` 来覆盖默认的 webpack 配置,然后在 `config-overrides.js` 中进行相关的配置。例如:
```
const lessRegex = /\.less$/;
const lessModuleRegex = /\.module\.less$/;
module.exports = function override(config, env) {
const lessLoader = {
loader: require.resolve('less-loader'),
options: {
lessOptions: {
javascriptEnabled: true
}
}
}
config.module.rules.push({
test: lessRegex,
exclude: lessModuleRegex,
use: [
'style-loader',
'css-loader',
lessLoader
]
});
config.module.rules.push({
test: lessModuleRegex,
use: [
'style-loader',
{
loader: 'css-loader',
options: {
modules: true
}
},
lessLoader
]
});
return config;
}
```
希望以上方法能帮助到你解决问题。
### 回答2:
报错"Cannot read properties of undefined"通常是因为在代码中使用了一个未定义的属性或方法。
在这种情况下,"import styles from './App.less'"导入的styles对象可能是未定义的。
有几个可能的原因导致这个错误。
首先,确保你的App.less文件存在,并且路径正确。如果路径错误,导入的对象将是undefined。
其次,确保你的App.less文件中导出了一个有效的样式对象。你可以检查一下App.less文件中是否有正确的样式导出。
另外,你需要确认你的项目是否正确地配置了less的解析器。确保你的项目中安装了less解析器,并且在webpack配置中正确设置了less-loader。如果配置不正确,less文件就无法正确解析,导致无法正确导入样式对象。
最后,尽量检查一下是否有其他报错或警告出现。有时候其他的报错或警告信息可能与该错误有关联。
总之,要解决这个问题,你需要逐个排查以上可能的原因,并确保路径、样式对象以及less解析器的配置正确无误。
### 回答3:
报错信息“Cannot read properties of undefined”表示在读取undefined的属性时出错。而在这个问题中,错误发生在`import styles from './App.less'`这行代码上。
这个报错通常发生在以下几种情况下:
1. 要引入的文件(./App.less)不存在。请检查路径是否输入正确,确保要引入的文件确实存在。
2. 引入的文件(./App.less)为空。在文件中没有定义任何对象或变量,导致读取属性时出错。请检查要引入的文件是否为空。
3. 引入的文件(./App.less)中没有导出需要的样式。在引入文件的代码中使用了styles对象的属性,但是在文件中未定义或未导出该样式。请检查要引入的文件中是否定义了需要的样式,并通过export关键字将其导出。
4. 使用的文件类型不支持。如果"./App.less"是一个不支持的文件类型,比如.png或.html,那么导入时会出现错误。请确保要导入的文件是支持的文件类型(如.less)。
总结:在使用`import styles from './App.less'`进行导入时,需要确保所引入的文件存在、非空,并且导出了需要的样式。
相关推荐
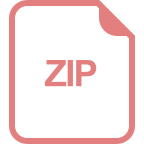
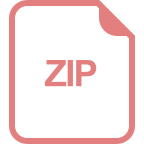
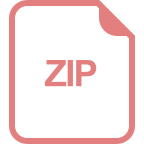













