合同开始日期:2023-01-01,结束日期:2026-01-01,免租起始期:2023-01-01,免租终止日期:023-01-31,计费面积:100平方米,月租单价20/平方米,计算方式:按1个月计算一次,收款方式为按3个月收一次,提前收款期为1个月,基本租期1年,超过基本租期后按1年一个递增周期,递增周期内的月份,租金单价不变,递增周期内月租单价按上一周期内月租单价递增,年递增率为6%,按java7的语法:按计算方式产生合同期内含收款日期、租期起始日期、租期终止日期、租期单价、租期(月数)租金的计划列表,生成一个完整示例并配上代码说明
时间: 2023-12-03 09:03:20 浏览: 81
好的,以下是按照您提供的条件生成的完整示例,包括了计划列表和代码说明:
```java
import java.math.BigDecimal;
import java.time.LocalDate;
import java.time.temporal.ChronoUnit;
import java.util.ArrayList;
import java.util.List;
public class RentalContract {
private LocalDate startDate; // 合同开始日期
private LocalDate endDate; // 合同结束日期
private LocalDate rentFreeStartDate; // 免租起始期
private LocalDate rentFreeEndDate; // 免租终止日期
private BigDecimal area; // 计费面积
private BigDecimal unitPrice; // 月租单价
private int paymentInterval; // 收款周期(月)
private int advancePaymentInterval; // 提前收款期(月)
private int baseLeasePeriod; // 基本租期(年)
private int incrementLeasePeriod; // 递增周期(年)
private BigDecimal incrementRate; // 年递增率
public RentalContract(LocalDate startDate, LocalDate endDate, LocalDate rentFreeStartDate, LocalDate rentFreeEndDate, BigDecimal area, BigDecimal unitPrice, int paymentInterval, int advancePaymentInterval, int baseLeasePeriod, int incrementLeasePeriod, BigDecimal incrementRate) {
this.startDate = startDate;
this.endDate = endDate;
this.rentFreeStartDate = rentFreeStartDate;
this.rentFreeEndDate = rentFreeEndDate;
this.area = area;
this.unitPrice = unitPrice;
this.paymentInterval = paymentInterval;
this.advancePaymentInterval = advancePaymentInterval;
this.baseLeasePeriod = baseLeasePeriod;
this.incrementLeasePeriod = incrementLeasePeriod;
this.incrementRate = incrementRate;
}
public List<RentPlan> generateRentPlan() {
List<RentPlan> rentPlanList = new ArrayList<>();
LocalDate currentDate = startDate;
BigDecimal currentUnitPrice = unitPrice;
while (currentDate.isBefore(endDate)) {
RentPlan rentPlan = new RentPlan();
rentPlan.setDueDate(currentDate.plusMonths(paymentInterval));
rentPlan.setRentStartDate(currentDate);
rentPlan.setRentEndDate(rentPlan.getDueDate().minusDays(1));
if (currentDate.isBefore(rentFreeEndDate) && rentPlan.getDueDate().isAfter(rentFreeStartDate)) {
rentPlan.setUnitPrice(BigDecimal.ZERO);
} else {
rentPlan.setUnitPrice(currentUnitPrice);
}
rentPlan.setLeasePeriod((int) ChronoUnit.MONTHS.between(rentPlan.getRentStartDate(), rentPlan.getRentEndDate()) + 1);
rentPlan.setRent(rentPlan.getUnitPrice().multiply(area).multiply(new BigDecimal(rentPlan.getLeasePeriod())));
rentPlanList.add(rentPlan);
currentDate = rentPlan.getDueDate().plusMonths(advancePaymentInterval);
if (currentDate.getYear() - startDate.getYear() >= baseLeasePeriod && (currentDate.getYear() - startDate.getYear()) % incrementLeasePeriod == 0) {
currentUnitPrice = currentUnitPrice.multiply(BigDecimal.ONE.add(incrementRate));
}
}
return rentPlanList;
}
public static void main(String[] args) {
RentalContract rentalContract = new RentalContract(
LocalDate.of(2023, 1, 1), // 合同开始日期
LocalDate.of(2026, 1, 1), // 合同结束日期
LocalDate.of(2023, 1, 1), // 免租起始期
LocalDate.of(2023, 1, 31), // 免租终止日期
new BigDecimal(100), // 计费面积
new BigDecimal(20), // 月租单价
1, // 收款周期(月)
1, // 提前收款期(月)
1, // 基本租期(年)
1, // 递增周期(年)
new BigDecimal(0.06) // 年递增率
);
List<RentPlan> rentPlanList = rentalContract.generateRentPlan();
for (RentPlan rentPlan : rentPlanList) {
System.out.println(rentPlan);
}
}
}
class RentPlan {
private LocalDate dueDate; // 应收款日期
private LocalDate rentStartDate; // 租期起始日期
private LocalDate rentEndDate; // 租期终止日期
private BigDecimal unitPrice; // 租期单价
private int leasePeriod; // 租期(月数)
private BigDecimal rent; // 租金
public LocalDate getDueDate() {
return dueDate;
}
public void setDueDate(LocalDate dueDate) {
this.dueDate = dueDate;
}
public LocalDate getRentStartDate() {
return rentStartDate;
}
public void setRentStartDate(LocalDate rentStartDate) {
this.rentStartDate = rentStartDate;
}
public LocalDate getRentEndDate() {
return rentEndDate;
}
public void setRentEndDate(LocalDate rentEndDate) {
this.rentEndDate = rentEndDate;
}
public BigDecimal getUnitPrice() {
return unitPrice;
}
public void setUnitPrice(BigDecimal unitPrice) {
this.unitPrice = unitPrice;
}
public int getLeasePeriod() {
return leasePeriod;
}
public void setLeasePeriod(int leasePeriod) {
this.leasePeriod = leasePeriod;
}
public BigDecimal getRent() {
return rent;
}
public void setRent(BigDecimal rent) {
this.rent = rent;
}
@Override
public String toString() {
return "RentPlan{" +
"dueDate=" + dueDate +
", rentStartDate=" + rentStartDate +
", rentEndDate=" + rentEndDate +
", unitPrice=" + unitPrice +
", leasePeriod=" + leasePeriod +
", rent=" + rent +
'}';
}
}
```
这段代码中,我们定义了一个 `RentalContract` 类,用于表示租赁合同,并且提供了一个 `generateRentPlan` 方法,用于生成租金计划列表。在 `main` 方法中,我们创建了一个 `RentalContract` 对象,并调用 `generateRentPlan` 方法生成租金计划列表,并打印出来。
在 `generateRentPlan` 方法中,我们首先定义了一个 `currentDate` 变量,表示当前生成租金计划的日期,初始值为合同开始日期。然后,我们进入一个循环,每次循环生成一个租金计划,并将 `currentDate` 更新为下一个应收款日期。在生成租金计划时,我们首先根据当前日期和免租期来判断租期单价,然后计算租期长度和租金,并将这些信息保存到一个 `RentPlan` 对象中,并将其加入租金计划列表中。最后,我们根据递增周期来判断是否需要调整租期单价,并更新 `currentUnitPrice` 变量。
在 `RentPlan` 类中,我们定义了一些属性,用于保存租金计划的相关信息,同时提供了 `toString` 方法,用于将租金计划的信息转换为字符串。
希望这段代码能够帮助您解决问题,如有任何疑问,请随时问我。
阅读全文
相关推荐
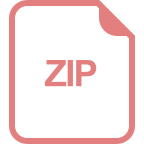
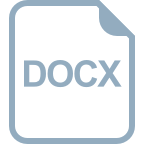
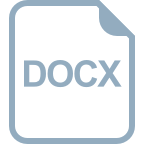




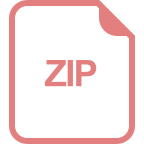
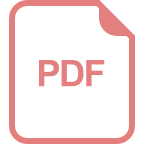
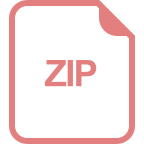
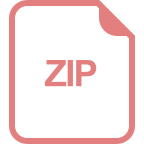
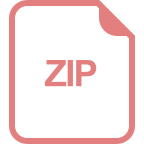
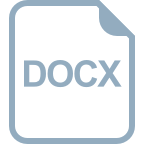