qt使用hpsocket的udp
时间: 2023-09-24 08:09:45 浏览: 49
使用 hpsocket 库实现 UDP 协议的代码如下:
```cpp
#include <QtCore/QCoreApplication>
#include <QHostAddress>
#include <QDebug>
#include "IUdpListener.h"
#include "Udp.h"
class MyUdpListener : public IUdpListener
{
public:
virtual EnHandleResult OnSend(IUdp* pSender, const BYTE* pData, int iLength, EnSendPolicy enPolicy)
{
qDebug() << "send data: " << QString::fromUtf8((const char*)pData, iLength);
return HR_OK;
}
virtual EnHandleResult OnReceive(IUdp* pSender, const BYTE* pData, int iLength, const HP_SOCKADDR& addr)
{
qDebug() << "receive data from " << QHostAddress(addr.Addr()).toString() << ": " << QString::fromUtf8((const char*)pData, iLength);
return HR_OK;
}
virtual EnHandleResult OnError(IUdp* pSender, EnSocketOperation enOperation, int iErrorCode)
{
qDebug() << "error: " << pSender->GetLastErrorDesc();
return HR_OK;
}
};
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
MyUdpListener listener;
CUdp udp(&listener);
if(!udp.Start("0.0.0.0", 8001))
{
qDebug() << "start error: " << udp.GetLastErrorDesc();
return -1;
}
if(!udp.Send("127.0.0.1", 8001, "hello udp", strlen("hello udp")))
{
qDebug() << "send error: " << udp.GetLastErrorDesc();
return -1;
}
return a.exec();
}
```
其中,`MyUdpListener` 是继承了 `IUdpListener` 接口的自定义类,用于处理 UDP 监听事件。在 `main` 函数中,我们创建了 `CUdp` 对象,并指定了 `MyUdpListener` 对象作为参数,然后调用 `Start` 方法启动 UDP 监听服务。最后,我们使用 `Send` 方法发送数据到指定的目标地址和端口。
需要注意的是,`CUdp` 类的实现依赖于 HP-Socket 库,你需要下载并安装该库,并将其包含路径和链接库路径添加到 Qt 项目中,以便编译通过。
相关推荐
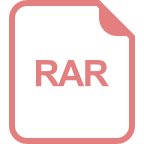













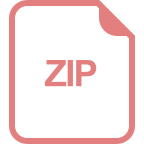
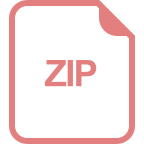