首页std::string encoded = base64_encode(reinterpret_cast<const unsigned char*>(s.c_str()), s.length());
std::string encoded = base64_encode(reinterpret_cast<const unsigned char*>(s.c_str()), s.length());
时间: 2024-06-04 13:10:04 浏览: 15
这段代码是使用Base64编码对std::string类型的字符串s进行编码,并将结果存储在std::string类型的变量encoded中。
具体来说,首先使用c_str()函数将std::string类型的字符串s转换为const char*类型的指针,再通过reinterpret_cast将其转换为const unsigned char*类型的指针。这是因为Base64编码是基于字节的,因此需要将字符串转换为字节流进行处理。
然后调用base64_encode函数对字节流进行编码,并将编码结果存储在std::string类型的变量encoded中。
需要注意的是,这段代码中使用了reinterpret_cast进行指针类型的转换,这是一种比较底层的操作,如果使用不当可能会导致程序出错。因此在实际开发中需要谨慎使用。
CSDN会员
开通CSDN年卡参与万元壕礼抽奖
最新推荐
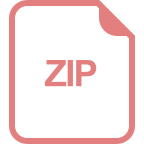
基于微信小程序的宠物小程序(免费提供全套java开源毕业设计源码+数据库+使用说明)
基于微信小程序的宠物小程序是一款专为宠物爱好者设计的综合性平台,旨在提供便捷的宠物服务和信息交流。该小程序充分利用微信生态,用户无需下载安装即可使用,具有轻量化、易操作的特点。
主要功能模块包括宠物信息管理、在线咨询、社区交流、宠物商城和服务预订。宠物信息管理模块允许用户添加和管理宠物的详细信息,包括品种、年龄、健康记录等。在线咨询模块提供与宠物医生或专家的即时沟通渠道,帮助用户解决宠物健康和养护问题。社区交流模块是一个互动平台,用户可以分享养宠心得、发布宠物照片、参与讨论,促进宠物爱好者之间的交流和互助。宠物商城模块提供各类宠物用品的在线购物服务,用户可以方便地购买到优质的宠物食品、玩具、护理用品等。服务预订模块则涵盖了宠物美容、医疗、寄养等服务,用户可以在线预订,享受便捷的宠物服务。
此外,小程序还具备推送提醒功能,定期提醒用户宠物的疫苗接种、健康检查等事项。整体而言,基于微信小程序的宠物小程序通过集成多种实用功能,为宠物主人提供了全面的宠物服务解决方案,提升了养宠体验,促进了宠物与主人之间的互动与关爱。
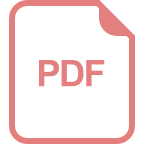
恒温水杯商业计划书.pdf
恒温水杯商业计划书.pdf
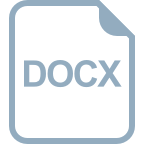
BSC关键绩效财务与客户指标详解
BSC(Balanced Scorecard,平衡计分卡)是一种战略绩效管理系统,它将企业的绩效评估从传统的财务维度扩展到非财务领域,以提供更全面、深入的业绩衡量。在提供的文档中,BSC绩效考核指标主要分为两大类:财务类和客户类。
1. 财务类指标:
- 部门费用的实际与预算比较:如项目研究开发费用、课题费用、招聘费用、培训费用和新产品研发费用,均通过实际支出与计划预算的百分比来衡量,这反映了部门在成本控制上的效率。
- 经营利润指标:如承保利润、赔付率和理赔统计,这些涉及保险公司的核心盈利能力和风险管理水平。
- 人力成本和保费收益:如人力成本与计划的比例,以及标准保费、附加佣金、续期推动费用等与预算的对比,评估业务运营和盈利能力。
- 财务效率:包括管理费用、销售费用和投资回报率,如净投资收益率、销售目标达成率等,反映公司的财务健康状况和经营效率。
2. 客户类指标:
- 客户满意度:通过包装水平客户满意度调研,了解产品和服务的质量和客户体验。
- 市场表现:通过市场销售月报和市场份额,衡量公司在市场中的竞争地位和销售业绩。
- 服务指标:如新契约标保完成度、续保率和出租率,体现客户服务质量和客户忠诚度。
- 品牌和市场知名度:通过问卷调查、公众媒体反馈和总公司级评价来评估品牌影响力和市场认知度。
BSC绩效考核指标旨在确保企业的战略目标与财务和非财务目标的平衡,通过量化这些关键指标,帮助管理层做出决策,优化资源配置,并驱动组织的整体业绩提升。同时,这份指标汇总文档强调了财务稳健性和客户满意度的重要性,体现了现代企业对多维度绩效管理的重视。

管理建模和仿真的文件
管理Boualem Benatallah引用此版本:布阿利姆·贝纳塔拉。管理建模和仿真。约瑟夫-傅立叶大学-格勒诺布尔第一大学,1996年。法语。NNT:电话:00345357HAL ID:电话:00345357https://theses.hal.science/tel-003453572008年12月9日提交HAL是一个多学科的开放存取档案馆,用于存放和传播科学研究论文,无论它们是否被公开。论文可以来自法国或国外的教学和研究机构,也可以来自公共或私人研究中心。L’archive ouverte pluridisciplinaire
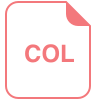
【实战演练】俄罗斯方块:实现经典的俄罗斯方块游戏,学习方块生成和行消除逻辑。
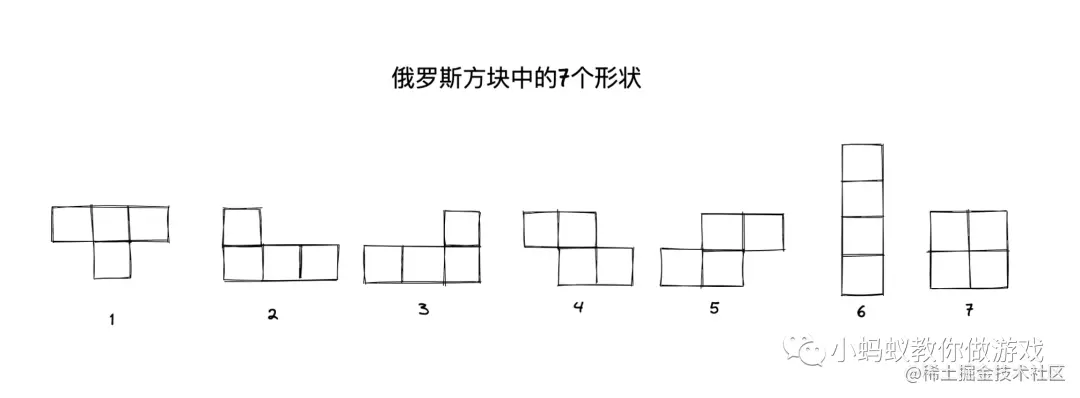
# 1. 俄罗斯方块游戏概述**
俄罗斯方块是一款经典的益智游戏,由阿列克谢·帕基特诺夫于1984年发明。游戏目标是通过控制不断下落的方块,排列成水平线,消除它们并获得分数。俄罗斯方块风靡全球,成为有史以来最受欢迎的视频游戏之一。
# 2.

卷积神经网络实现手势识别程序
卷积神经网络(Convolutional Neural Network, CNN)在手势识别中是一种非常有效的机器学习模型。CNN特别适用于处理图像数据,因为它能够自动提取和学习局部特征,这对于像手势这样的空间模式识别非常重要。以下是使用CNN实现手势识别的基本步骤:
1. **输入数据准备**:首先,你需要收集或获取一组带有标签的手势图像,作为训练和测试数据集。
2. **数据预处理**:对图像进行标准化、裁剪、大小调整等操作,以便于网络输入。
3. **卷积层(Convolutional Layer)**:这是CNN的核心部分,通过一系列可学习的滤波器(卷积核)对输入图像进行卷积,以
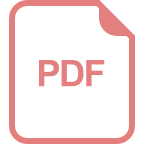
绘制企业战略地图:从财务到客户价值的六步法
"BSC资料.pdf"
战略地图是一种战略管理工具,它帮助企业将战略目标可视化,确保所有部门和员工的工作都与公司的整体战略方向保持一致。战略地图的核心内容包括四个相互关联的视角:财务、客户、内部流程和学习与成长。
1. **财务视角**:这是战略地图的最终目标,通常表现为股东价值的提升。例如,股东期望五年后的销售收入达到五亿元,而目前只有一亿元,那么四亿元的差距就是企业的总体目标。
2. **客户视角**:为了实现财务目标,需要明确客户价值主张。企业可以通过提供最低总成本、产品创新、全面解决方案或系统锁定等方式吸引和保留客户,以实现销售额的增长。
3. **内部流程视角**:确定关键流程以支持客户价值主张和财务目标的实现。主要流程可能包括运营管理、客户管理、创新和社会责任等,每个流程都需要有明确的短期、中期和长期目标。
4. **学习与成长视角**:评估和提升企业的人力资本、信息资本和组织资本,确保这些无形资产能够支持内部流程的优化和战略目标的达成。
绘制战略地图的六个步骤:
1. **确定股东价值差距**:识别与股东期望之间的差距。
2. **调整客户价值主张**:分析客户并调整策略以满足他们的需求。
3. **设定价值提升时间表**:规划各阶段的目标以逐步缩小差距。
4. **确定战略主题**:识别关键内部流程并设定目标。
5. **提升战略准备度**:评估并提升无形资产的战略准备度。
6. **制定行动方案**:根据战略地图制定具体行动计划,分配资源和预算。
战略地图的有效性主要取决于两个要素:
1. **KPI的数量及分布比例**:一个有效的战略地图通常包含20个左右的指标,且在四个视角之间有均衡的分布,如财务20%,客户20%,内部流程40%。
2. **KPI的性质比例**:指标应涵盖财务、客户、内部流程和学习与成长等各个方面,以全面反映组织的绩效。
战略地图不仅帮助管理层清晰传达战略意图,也使员工能更好地理解自己的工作如何对公司整体目标产生贡献,从而提高执行力和组织协同性。

"互动学习:行动中的多样性与论文攻读经历"
多样性她- 事实上SCI NCES你的时间表ECOLEDO C Tora SC和NCESPOUR l’Ingén学习互动,互动学习以行动为中心的强化学习学会互动,互动学习,以行动为中心的强化学习计算机科学博士论文于2021年9月28日在Villeneuve d'Asq公开支持马修·瑟林评审团主席法布里斯·勒菲弗尔阿维尼翁大学教授论文指导奥利维尔·皮耶昆谷歌研究教授:智囊团论文联合主任菲利普·普雷教授,大学。里尔/CRISTAL/因里亚报告员奥利维耶·西格德索邦大学报告员卢多维奇·德诺耶教授,Facebook /索邦大学审查员越南圣迈IMT Atlantic高级讲师邀请弗洛里安·斯特鲁布博士,Deepmind对于那些及时看到自己错误的人...3谢谢你首先,我要感谢我的两位博士生导师Olivier和Philippe。奥利维尔,"站在巨人的肩膀上"这句话对你来说完全有意义了。从科学上讲,你知道在这篇论文的(许多)错误中,你是我可以依
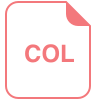
【实战演练】井字棋游戏:开发井字棋游戏,重点在于AI对手的实现。
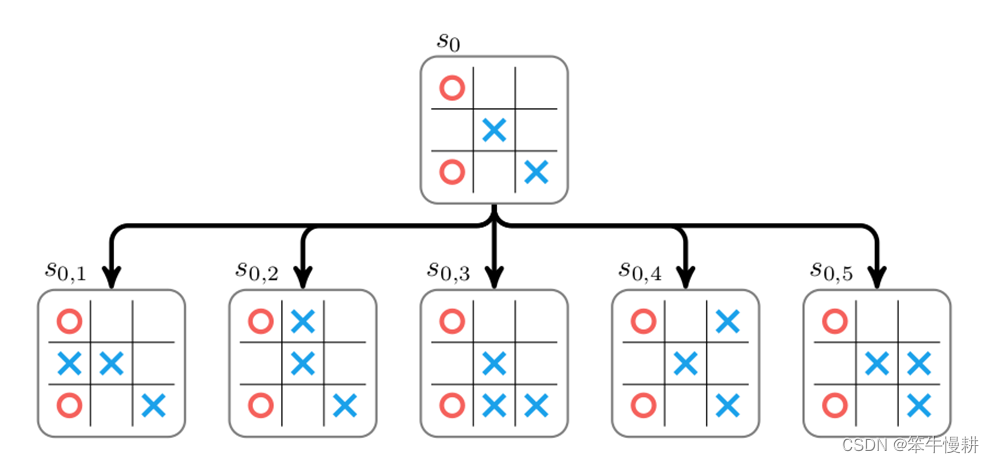
# 2.1 井字棋游戏规则
井字棋游戏是一个两人对弈的游戏,在3x3的棋盘上进行。玩家轮流在空位上放置自己的棋子(通常为“X”或“O”),目标是让自己的棋子连成一条直线(水平、垂直或对角线)。如果某位玩家率先完成这一目标,则该玩家获胜。
游戏开始时,棋盘上所有位置都为空。玩家轮流放置自己的棋子,直到出现以下情况之一:
* 有玩家连成一条直线,获胜。
* 棋盘上所有位置都被占满,平局。

transformer模型对话
Transformer模型是一种基于自注意力机制的深度学习架构,最初由Google团队在2017年的论文《Attention is All You Need》中提出,主要用于自然语言处理任务,如机器翻译和文本生成。Transformer完全摒弃了传统的循环神经网络(RNN)和卷积神经网络(CNN),转而采用全连接的方式处理序列数据,这使得它能够并行计算,极大地提高了训练速度。
在对话系统中,Transformer模型通过编码器-解码器结构工作。编码器将输入序列转化为固定长度的上下文向量,而解码器则根据这些向量逐步生成响应,每一步都通过自注意力机制关注到输入序列的所有部分,这使得模型能够捕捉到