请用才c++写一个图书管理系统
时间: 2023-04-08 08:02:02 浏览: 85
好的,我可以回答这个问题。一个基本的图书管理系统需要以下功能:
1. 添加图书:管理员可以添加新的图书到系统中,包括书名、作者、出版社、ISBN 码、分类等信息。
2. 删除图书:管理员可以删除系统中已有的图书。
3. 修改图书信息:管理员可以修改系统中已有的图书的信息。
4. 查询图书:用户可以通过关键字、作者、分类等信息查询系统中的图书。
5. 借阅图书:用户可以借阅系统中的图书,管理员可以查看借阅情况。
6. 归还图书:用户可以归还已借阅的图书,管理员可以更新借阅情况。
以下是一个简单的图书管理系统的代码示例:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_BOOKS 100
struct Book {
char title[100];
char author[50];
char publisher[50];
char isbn[20];
char category[20];
int available;
};
struct Book books[MAX_BOOKS];
int num_books = 0;
void add_book() {
if (num_books >= MAX_BOOKS) {
printf("Error: too many books\n");
return;
}
struct Book book;
printf("Enter book title: ");
fgets(book.title, 100, stdin);
printf("Enter author name: ");
fgets(book.author, 50, stdin);
printf("Enter publisher name: ");
fgets(book.publisher, 50, stdin);
printf("Enter ISBN: ");
fgets(book.isbn, 20, stdin);
printf("Enter category: ");
fgets(book.category, 20, stdin);
book.available = 1;
books[num_books++] = book;
printf("Book added successfully\n");
}
void delete_book() {
char isbn[20];
printf("Enter ISBN of book to delete: ");
fgets(isbn, 20, stdin);
for (int i = 0; i < num_books; i++) {
if (strcmp(books[i].isbn, isbn) == 0) {
for (int j = i; j < num_books - 1; j++) {
books[j] = books[j+1];
}
num_books--;
printf("Book deleted successfully\n");
return;
}
}
printf("Error: book not found\n");
}
void modify_book() {
char isbn[20];
printf("Enter ISBN of book to modify: ");
fgets(isbn, 20, stdin);
for (int i = 0; i < num_books; i++) {
if (strcmp(books[i].isbn, isbn) == 0) {
printf("Enter new title (or press enter to keep current title): ");
fgets(books[i].title, 100, stdin);
printf("Enter new author (or press enter to keep current author): ");
fgets(books[i].author, 50, stdin);
printf("Enter new publisher (or press enter to keep current publisher): ");
fgets(books[i].publisher, 50, stdin);
printf("Enter new category (or press enter to keep current category): ");
fgets(books[i].category, 20, stdin);
printf("Book modified successfully\n");
return;
}
}
printf("Error: book not found\n");
}
void search_books() {
char keyword[100];
printf("Enter search keyword: ");
fgets(keyword, 100, stdin);
for (int i = 0; i < num_books; i++) {
if (strstr(books[i].title, keyword) != NULL ||
strstr(books[i].author, keyword) != NULL ||
strstr(books[i].publisher, keyword) != NULL ||
strstr(books[i].category, keyword) != NULL) {
printf("%s by %s, published by %s, ISBN: %s, category: %s, available: %s\n",
books[i].title, books[i].author, books[i].publisher, books[i].isbn,
books[i].category, books[i].available ? "yes" : "no");
}
}
}
void borrow_book() {
char isbn[20];
printf("Enter ISBN of book to borrow: ");
fgets(isbn, 20, stdin);
for (int i = 0; i < num_books; i++) {
if (strcmp(books[i].isbn, isbn) == 0) {
if (books[i].available) {
books[i].available = 0;
printf("Book borrowed successfully\n");
} else {
printf("Error: book not available\n");
}
return;
}
}
printf("Error: book not found\n");
}
void return_book() {
char isbn[20];
printf("Enter ISBN of book to return: ");
fgets(isbn, 20, stdin);
for (int i = 0; i < num_books; i++) {
if (strcmp(books[i].isbn, isbn) == 0) {
if (!books[i].available) {
books[i].available = 1;
printf("Book returned successfully\n");
} else {
printf("Error: book not borrowed\n");
}
return;
}
}
printf("Error: book not found\n");
}
int main() {
while (1) {
printf("1. Add book\n");
printf("2. Delete book\n");
printf("3. Modify book\n");
printf("4. Search books\n");
printf("5. Borrow book\n");
printf("6. Return book\n");
printf("7. Exit\n");
printf("Enter your choice: ");
int choice;
scanf("%d", &choice);
getchar();
switch (choice) {
case 1:
add_book();
break;
case 2:
delete_book();
break;
case 3:
modify_book();
break;
case 4:
search_books();
break;
case 5:
borrow_book();
break;
case 6:
return_book();
break;
case 7:
exit(0);
default:
printf("Invalid choice\n");
}
}
return 0;
}
相关推荐
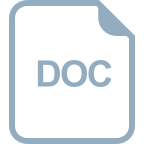










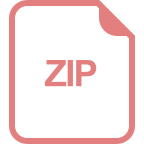
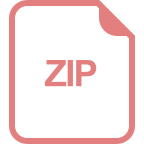
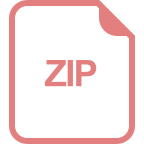
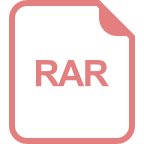
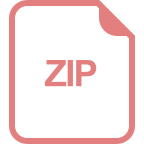