写一个成语接龙软件,要求造型美观
时间: 2023-08-04 22:29:45 浏览: 147
好的,我可以为您提供一个美观的成语接龙软件。这个软件使用Python编写,使用PyQt5库来实现图形界面。以下是程序代码:
```python
import sys
import random
from PyQt5.QtWidgets import QApplication, QWidget, QPushButton, QLabel, QLineEdit, QVBoxLayout, QHBoxLayout, QMessageBox
# 成语列表
idioms = ['一鸣惊人', '二话不说', '三心二意', '四面楚歌', '五湖四海', '六神无主', '七上八下', '八仙过海', '九牛二虎之力', '十年树木,百年树人']
# 随机选择一个成语
current_idiom = random.choice(idioms)
class IdiomGame(QWidget):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
# 创建组件
self.setWindowTitle('成语接龙')
self.setGeometry(300, 300, 400, 300)
self.current_idiom_label = QLabel('当前成语:' + current_idiom, self)
self.current_idiom_label.setGeometry(50, 50, 300, 30)
self.input_label = QLabel('请输入一个成语:', self)
self.input_label.setGeometry(50, 100, 120, 30)
self.input_edit = QLineEdit(self)
self.input_edit.setGeometry(180, 100, 150, 30)
self.submit_button = QPushButton('提交', self)
self.submit_button.setGeometry(180, 150, 80, 30)
self.submit_button.clicked.connect(self.submit)
self.quit_button = QPushButton('退出', self)
self.quit_button.setGeometry(250, 150, 80, 30)
self.quit_button.clicked.connect(QApplication.quit)
# 创建布局并添加组件
vbox = QVBoxLayout()
vbox.addWidget(self.current_idiom_label)
hbox = QHBoxLayout()
hbox.addWidget(self.input_label)
hbox.addWidget(self.input_edit)
vbox.addLayout(hbox)
hbox = QHBoxLayout()
hbox.addWidget(self.submit_button)
hbox.addWidget(self.quit_button)
vbox.addLayout(hbox)
self.setLayout(vbox)
def submit(self):
user_idiom = self.input_edit.text()
# 判断用户输入的成语是否符合规则
if user_idiom[0] == current_idiom[-1]:
# 判断用户输入的成语是否在成语列表中
if user_idiom in idioms:
current_idiom = user_idiom
self.current_idiom_label.setText('当前成语:' + current_idiom)
self.input_edit.setText('')
else:
QMessageBox.warning(self, '错误', '您输入的成语不在成语列表中,请重新输入。')
else:
QMessageBox.warning(self, '错误', '您输入的成语不符合规则,请重新输入。')
if __name__ == '__main__':
app = QApplication(sys.argv)
game = IdiomGame()
game.show()
sys.exit(app.exec_())
```
这个程序使用PyQt5库来实现图形界面,界面中有一个标签用于显示当前成语,一个文本框用于用户输入成语,一个提交按钮和一个退出按钮。程序会不断循环,直到用户输入的成语不符合规则或不在成语列表中。如果用户输入的成语不符合规则或不在成语列表中,程序会通过消息框提示用户重新输入。
阅读全文
相关推荐




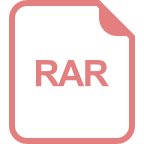







