用Android Studio实现消息提示Toast的代码,目标如下:1.通过点击三个按钮分别实现Toast的默认方式、自定义方式、带图标方式3种显示方式。2.用图片作为消息提示的图标
时间: 2024-05-03 10:22:01 浏览: 158
以下是用Android Studio实现消息提示Toast的代码,实现了点击三个按钮分别实现Toast的默认方式、自定义方式、带图标方式3种显示方式,并使用了图片作为消息提示的图标。
XML布局文件:
```
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:gravity="center">
<Button
android:id="@+id/default_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Default Toast"/>
<Button
android:id="@+id/custom_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Custom Toast"/>
<Button
android:id="@+id/icon_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Icon Toast"/>
</LinearLayout>
```
Java代码:
```
public class MainActivity extends AppCompatActivity {
private Button defaultButton, customButton, iconButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
defaultButton = findViewById(R.id.default_button);
customButton = findViewById(R.id.custom_button);
iconButton = findViewById(R.id.icon_button);
defaultButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Toast.makeText(MainActivity.this, "This is a default toast message.", Toast.LENGTH_SHORT).show();
}
});
customButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
LayoutInflater inflater = getLayoutInflater();
View layout = inflater.inflate(R.layout.custom_toast_layout,
(ViewGroup) findViewById(R.id.custom_toast_container));
TextView text = layout.findViewById(R.id.text);
text.setText("This is a custom toast message.");
Toast toast = new Toast(getApplicationContext());
toast.setGravity(Gravity.CENTER_VERTICAL, 0, 0);
toast.setDuration(Toast.LENGTH_SHORT);
toast.setView(layout);
toast.show();
}
});
iconButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
LayoutInflater inflater = getLayoutInflater();
View layout = inflater.inflate(R.layout.icon_toast_layout,
(ViewGroup) findViewById(R.id.icon_toast_container));
ImageView image = layout.findViewById(R.id.image);
image.setImageResource(R.drawable.icon);
TextView text = layout.findViewById(R.id.text);
text.setText("This is an icon toast message.");
Toast toast = new Toast(getApplicationContext());
toast.setGravity(Gravity.CENTER_VERTICAL, 0, 0);
toast.setDuration(Toast.LENGTH_SHORT);
toast.setView(layout);
toast.show();
}
});
}
}
```
custom_toast_layout.xml布局文件:
```
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/custom_toast_container"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="@drawable/custom_toast_background"
android:orientation="horizontal"
android:padding="16dp">
<TextView
android:id="@+id/text"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textColor="#FFFFFF"
android:textSize="16sp" />
</LinearLayout>
```
icon_toast_layout.xml布局文件:
```
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/icon_toast_container"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="@drawable/icon_toast_background"
android:orientation="horizontal"
android:padding="16dp">
<ImageView
android:id="@+id/image"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginRight="16dp" />
<TextView
android:id="@+id/text"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textColor="#FFFFFF"
android:textSize="16sp" />
</LinearLayout>
```
custom_toast_background.xml和icon_toast_background.xml文件分别为自定义Toast的背景,可以根据需要自行设置。
阅读全文
相关推荐
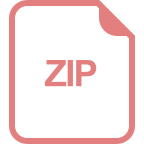
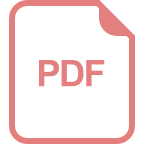
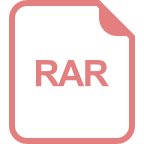















