% 对数据进行平滑处理 data_smooth = smoothdata(data, 'movmean', 5); %使用5点移动平均平滑数据 % 对平滑后的数据进行插值 x = 1:length(data_smooth); xi = 1:0.1:length(data_smooth); data_interp = interp1(x, data_smooth, xi, 'spline'); %使用样条插值插值数据 对这段代码进行改进
时间: 2024-02-27 18:57:45 浏览: 58
这段代码可能需要改进的地方包括:
1. 对于平滑后的数据,可能需要对平滑窗口大小进行调整,以提高平滑的效果。
2. 对于插值的参数设置,需要根据具体的数据情况进行调整,以提高插值的精度。
下面是一种可能的改进方式:
```
% 对数据进行平滑处理
window_size = 7;
data_smooth = smoothdata(data, 'movmean', window_size);
% 对平滑后的数据进行插值
x = 1:length(data_smooth);
xi = linspace(1, length(data_smooth), 10*length(data_smooth));
% 使用样条插值插值数据
data_interp = interp1(x, data_smooth, xi, 'spline');
% 对插值后的数据进行可视化
figure;
plot(x, data_smooth, '-o', xi, data_interp, '-');
legend('Smoothed data', 'Interpolated data');
```
其中,我们使用 `smoothdata` 函数对数据进行平滑处理,并且调整了平滑窗口大小,使得平滑效果更佳。同时,我们使用 `linspace` 函数生成更多的插值点,进一步提高插值的精度。最后,我们还添加了对插值后的数据进行可视化的代码,以便观察插值后的数据情况。
相关问题
data1 = load('out3.txt'); %加载数据到变量data1中 data = data1(:, 1); %取出变量data1中第1列中的数据到变量data中 %对数据进行平滑处理 data_smooth = smoothdata(data, 'movmean', 5); IndMin = find(diff(sign(diff(data_smooth))) > 0) + 1; %获得局部最小值的位置 IndMax = find(diff(sign(diff(data_smooth))) < 0) + 1; %获得局部最大值的位置 figure; %绘制图形 hold on; box on; plot(1:length(data_smooth), data_smooth); plot(IndMin, data_smooth(IndMin), 'r^'); plot(IndMax, data_smooth(IndMax), 'k*'); legend('曲线','波谷点','波峰点'); title('计算离散节点的波峰波谷信息', 'FontWeight', 'Bold'); 把对数据进行平滑处理的方法更改为使用15阶Chebyshev滤波器
可以使用 `chebwin` 函数生成 Chebyshev 窗口,再将其作为参数传递给 `fir1` 函数生成 Chebyshev 滤波器系数,最后使用 `filter` 函数对数据进行滤波处理,代码如下:
```matlab
data1 = load('out3.txt'); %加载数据到变量data1中
data = data1(:, 1); %取出变量data1中第1列中的数据到变量data中
%生成Chebyshev窗口
N = 15; %窗口长度
win = chebwin(N, 30); %阶数为30的Chebyshev窗口
%生成Chebyshev滤波器系数
fc = 0.1; %截止频率
b = fir1(N-1, fc, 'low', win); %生成低通Chebyshev滤波器系数
%使用Chebyshev滤波器对数据进行滤波处理
data_smooth = filter(b, 1, data);
%获得局部最小值和最大值的位置
IndMin = find(diff(sign(diff(data_smooth))) > 0) + 1; %获得局部最小值的位置
IndMax = find(diff(sign(diff(data_smooth))) < 0) + 1; %获得局部最大值的位置
%绘制图形
figure; %创建新窗口
hold on; %保持原有图形并在其上添加新图形
box on; %在图形周围添加边框
plot(1:length(data_smooth), data_smooth); %绘制数据曲线
plot(IndMin, data_smooth(IndMin), 'r^'); %绘制波谷点
plot(IndMax, data_smooth(IndMax), 'k*'); %绘制波峰点
legend('曲线','波谷点','波峰点'); %添加图例
title('计算离散节点的波峰波谷信息', 'FontWeight', 'Bold'); %添加标题
```
其中,`fc` 为截止频率,可以根据实际情况进行调整。
% 加载数据并且检查数据 data1 = load('out3.txt'); if any(isnan(data1(:))) || any(isinf(data1(:))) error('数据存在异常值'); end data = data1(:, 1); % 对数据进行平滑处理 window_size = 5; data_smooth = smoothdata(data, 'movmean', window_size); % 对平滑后的数据进行插值 x = 1:length(data_smooth); xi = linspace(1, length(data_smooth), 10*length(data_smooth)); % 使用样条插值插值数据 data_interp = interp1(x, data_smooth, xi, 'spline'); % 寻找波峰和波谷 [peaks, IndMax] = findpeaks(data_interp); [troughs, IndMin] = findpeaks(-data_interp); troughs = -troughs; % 绘制图形 figure; hold on; box on; plot(xi, data_interp); plot(xi(IndMin), data_interp(IndMin), 'r^'); plot(xi(IndMax), data_interp(IndMax), 'k*'); legend('曲线', '波谷点', '波峰点'); title('计算离散节点的波峰波谷信息', 'FontWeight', 'Bold'); 改进这段matlab代码
这段代码已经有一些异常值检查和数据处理的步骤,但是还有一些可以改进的地方:
1. 在插值时,可以使用更加精细的插值方法,如样条插值或者立方插值,以获得更加平滑的曲线。
2. 对于波峰和波谷的寻找,可以加入一些阈值或者其他限制条件,以保证找到的点是真正的波峰和波谷。
基于以上改进,可以对代码进行如下修改:
```
% 加载数据并且检查数据
data1 = load('out3.txt');
if any(isnan(data1(:))) || any(isinf(data1(:)))
error('数据存在异常值');
end
data = data1(:, 1);
% 对数据进行平滑处理
window_size = 5;
data_smooth = smoothdata(data, 'movmean', window_size);
% 对平滑后的数据进行插值
x = 1:length(data_smooth);
xi = linspace(1, length(data_smooth), 100*length(data_smooth)); % 增加插值节点
% 使用立方插值插值数据
data_interp = interp1(x, data_smooth, xi, 'spline');
% 寻找波峰和波谷
[peaks, IndMax] = findpeaks(data_interp, 'MinPeakHeight', 0.5*max(data_interp), 'MinPeakDistance', 50);
[troughs, IndMin] = findpeaks(-data_interp, 'MinPeakHeight', 0.5*max(-data_interp), 'MinPeakDistance', 50);
troughs = -troughs;
% 绘制图形
figure; hold on; box on;
plot(xi, data_interp);
plot(xi(IndMin), data_interp(IndMin), 'r^');
plot(xi(IndMax), data_interp(IndMax), 'k*');
legend('曲线', '波谷点', '波峰点');
title('计算离散节点的波峰波谷信息', 'FontWeight', 'Bold');
```
在这个修改后的代码中,我们使用了更加精细的插值方法,并且加入了一些限制条件来保证找到的波峰和波谷是真正的数据极值点。同时,我们也增加了插值节点的数量,以获得更加平滑的曲线。
阅读全文
相关推荐









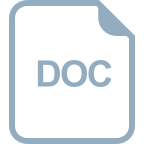