java + mysql + jdbc实现图片读取并显示代码
时间: 2023-01-28 19:59:24 浏览: 120
好的,下面是使用 Java、MySQL 和 JDBC 来读取并显示图片的代码示例:
首先,需要在 MySQL 中创建一张表来存储图片,例如:
```
CREATE TABLE images (
id INT PRIMARY KEY AUTO_INCREMENT,
name VARCHAR(255) NOT NULL,
data LONGBLOB NOT NULL
);
```
然后,使用 JDBC 将图片插入到 MySQL 数据库中:
```
Connection conn = null;
PreparedStatement pstmt = null;
FileInputStream fis = null;
try {
// 建立数据库连接
conn = DriverManager.getConnection(DB_URL, USERNAME, PASSWORD);
// 创建 PreparedStatement
pstmt = conn.prepareStatement("INSERT INTO images (name, data) VALUES (?, ?)");
// 设置参数
pstmt.setString(1, "image.jpg");
fis = new FileInputStream("/path/to/image.jpg");
pstmt.setBinaryStream(2, fis, fis.available());
// 执行插入操作
pstmt.executeUpdate();
} catch (SQLException | IOException e) {
e.printStackTrace();
} finally {
// 关闭连接
if (pstmt != null) pstmt.close();
if (conn != null) conn.close();
if (fis != null) fis.close();
}
```
最后,使用 JDBC 从 MySQL 中读取图片并显示:
```
Connection conn = null;
PreparedStatement pstmt = null;
ResultSet rs = null;
try {
// 建立数据库连接
conn = DriverManager.getConnection(DB_URL, USERNAME, PASSWORD);
// 创建 Prepared Statement
pstmt = conn.prepareStatement("SELECT data FROM images WHERE name=?");
// 设置参数
pstmt.setString(1, "image.jpg");
// 执行查询操作
rs = pstmt.executeQuery();
if (rs.next()) {
// 获取图片数据
byte[] data = rs.getBytes("data");
// 创建图片对象
BufferedImage image = ImageIO.read(new
阅读全文
相关推荐


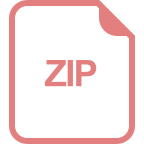
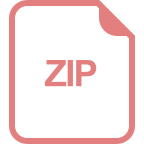
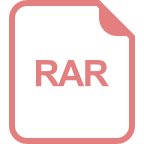
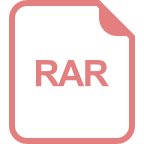
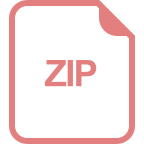
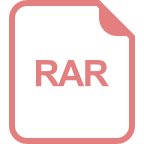
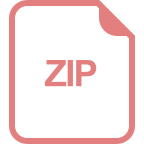
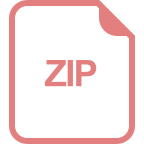
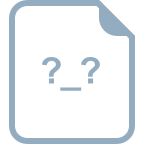
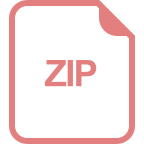
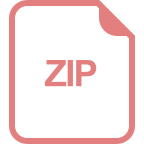
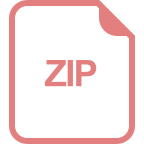
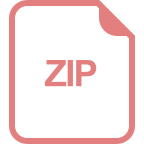
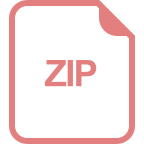