基本要求 (1)以栈模拟停车场,以队列模拟车场外的便道,按照从终端读入的输入数据序列进行模拟管理。 (2) 每一组输入数据包括三个数据项:汽车“到达”或“离去”信息、汽车牌照号码以及到达或离去的时刻。 (3)对每一组输入数据进行操作后的输出信息为:若是车辆到达,则输出汽车在停车场内或便道上的停车位置;若是车辆离去,则输出汽车在停车场内停留的时间和应交纳的费用(在便道上停留的时间不收费)。 (4)栈以顺序结构实现,队列以循环队列实现。
时间: 2024-04-02 09:35:09 浏览: 12
以下是基于C++的停车场管理系统的实现,其中栈使用了顺序结构,队列使用了循环队列。
```cpp
#include <iostream>
#include <cstring>
#include <ctime>
using namespace std;
const int MAX_SIZE = 10; // 停车场最大容量
const int MAX_TIME = 24; // 一天最大时间
enum ActionType { ARRIVE, DEPART }; // 汽车到达或离去的枚举类型
struct Car {
char license[8]; // 车牌号
int arrive_time; // 到达时间
int park_time; // 停留时间
int fee; // 停车费用
};
class Stack {
public:
Stack() {
top = -1;
}
bool is_empty() {
return top == -1;
}
bool is_full() {
return top == MAX_SIZE - 1;
}
void push(Car car) {
if (is_full()) {
cerr << "The parking lot is full!" << endl;
return;
}
cars[++top] = car;
cout << car.license << " arrives at parking lot, position: " << top + 1 << endl;
}
Car pop() {
if (is_empty()) {
cerr << "The parking lot is empty!" << endl;
return {};
}
Car car = cars[top--];
return car;
}
int find_car(char* license) {
for (int i = top; i >= 0; i--) {
if (strcmp(cars[i].license, license) == 0) {
return i;
}
}
return -1;
}
Car get_car(int pos) {
return cars[pos];
}
void show_cars() {
cout << "Cars in parking lot: " << endl;
for (int i = top; i >= 0; i--) {
cout << "Position " << i + 1 << ": " << cars[i].license << endl;
}
}
private:
Car cars[MAX_SIZE];
int top;
};
class Queue {
public:
Queue() {
front = rear = 0;
}
bool is_empty() {
return front == rear;
}
bool is_full() {
return (rear + 1) % MAX_SIZE == front;
}
void push(Car car) {
if (is_full()) {
cerr << "The waiting queue is full!" << endl;
return;
}
cars[rear] = car;
rear = (rear + 1) % MAX_SIZE;
cout << car.license << " arrives at waiting queue, position: " << rear << endl;
}
Car pop() {
if (is_empty()) {
cerr << "The waiting queue is empty!" << endl;
return {};
}
Car car = cars[front];
front = (front + 1) % MAX_SIZE;
return car;
}
void show_cars() {
cout << "Cars in waiting queue: " << endl;
for (int i = front; i != rear; i = (i + 1) % MAX_SIZE) {
cout << "Position " << (i + 1) % MAX_SIZE << ": " << cars[i].license << endl;
}
}
private:
Car cars[MAX_SIZE];
int front, rear;
};
int main() {
srand(time(NULL)); // 初始化随机数种子
Stack parking_lot; // 停车场
Queue waiting_queue; // 便道
while (true) {
cout << "Please enter action type (0 for arrive, 1 for depart): ";
int action_type;
cin >> action_type;
if (action_type != ARRIVE && action_type != DEPART) {
cerr << "Invalid action type!" << endl;
continue;
}
cout << "Please enter car license: ";
char license[8];
cin >> license;
cout << "Please enter time (0 ~ 23): ";
int time;
cin >> time;
if (time < 0 || time > MAX_TIME) {
cerr << "Invalid time!" << endl;
continue;
}
if (action_type == ARRIVE) {
Car car;
strcpy(car.license, license);
car.arrive_time = time;
car.park_time = -1; // 表示未停留
car.fee = -1; // 表示未产生费用
if (!parking_lot.is_full()) { // 停车场未满,直接停车
parking_lot.push(car);
} else { // 停车场已满,需停放到便道
waiting_queue.push(car);
}
} else { // 汽车离去
int pos = parking_lot.find_car(license);
if (pos != -1) { // 汽车在停车场内
Car car = parking_lot.get_car(pos);
car.park_time = time - car.arrive_time;
car.fee = car.park_time * 10; // 停车费用为每小时10元
cout << car.license << " departs from parking lot, park time: " << car.park_time << " hours, fee: " << car.fee << " yuan" << endl;
// 删除停车场内的车辆
for (int i = pos + 1; i <= parking_lot.top; i++) {
parking_lot.cars[i - 1] = parking_lot.cars[i];
}
parking_lot.pop();
// 将便道上的车辆移动到停车场
if (!waiting_queue.is_empty()) {
Car car = waiting_queue.pop();
parking_lot.push(car);
}
} else { // 汽车不在停车场内
pos = waiting_queue.find_car(license);
if (pos != -1) { // 汽车在便道上
cout << license << " departs from waiting queue" << endl;
// 删除便道上的车辆
int i = pos;
while ((i + 1) % MAX_SIZE != waiting_queue.rear) {
waiting_queue.cars[i] = waiting_queue.cars[(i + 1) % MAX_SIZE];
i = (i + 1) % MAX_SIZE;
}
waiting_queue.rear = (waiting_queue.rear - 1 + MAX_SIZE) % MAX_SIZE;
} else { // 汽车不存在
cerr << "Car not found!" << endl;
}
}
}
// 显示停车场和便道上的车辆
parking_lot.show_cars();
waiting_queue.show_cars();
}
return 0;
}
```
在这个实现中,使用了两个类来模拟停车场和便道。当有车辆到达时,如果停车场未满,则直接停车,否则停放到便道。当有车辆离去时,先在停车场中查找,如果找到则计算停留时间和停车费用,并从停车场中删除该车辆。如果未找到,则在便道上查找,如果找到则删除该车辆。在车辆到达或离去后,显示停车场和便道上的车辆。
需要注意的是,由于输入数据中的时间是整数,表示小时数,因此本程序假设一天最大时间为24小时。停车费用为每小时10元,可以根据需求进行修改。
相关推荐
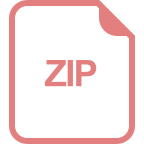
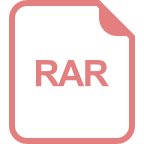
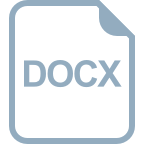








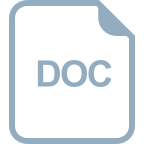
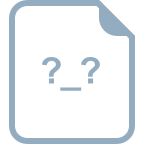
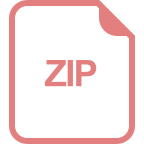
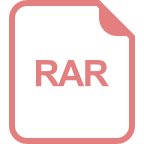
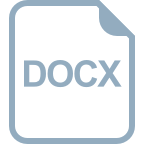
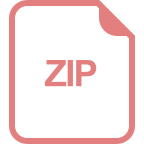
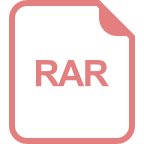