用python把表格的首行填充成浅蓝色
时间: 2023-06-07 07:07:34 浏览: 60
您可以使用 openpyxl 库来操作 Excel 文件,其中包含设定单元格填充颜色的方法。
下面是一个示例代码:
```python
import openpyxl
from openpyxl.styles import PatternFill, Alignment
# 打开 Excel 文件
wb = openpyxl.load_workbook('example.xlsx')
# 获取第一个工作表
ws = wb.active
# 设定样式
fill = PatternFill(start_color='B7E1CD', end_color='B7E1CD', fill_type='solid')
font = ws.cell(1, 1).font
alignment = Alignment(horizontal='center', vertical='center', wrap_text=True)
# 设定单元格样式
for col in ws.iter_cols(min_row=1, max_row=1):
for cell in col:
cell.fill = fill
cell.font = font
cell.alignment = alignment
# 保存文件
wb.save('example.xlsx')
```
这段代码会打开名为 `example.xlsx` 的 Excel 文件,将第一行单元格的背景色设定为浅蓝色,保存后退出。
相关问题
用pandas把表格的首行填充成浅蓝色
您好!感谢您对ChitGPT的信任与使用!对于您的问题,我可以提供如下的代码示例:
```python
import pandas as pd
from openpyxl.styles import PatternFill
from openpyxl.utils.dataframe import dataframe_to_rows
# 创建一个简单的数据表
df = pd.DataFrame({
'姓名': ['张三', '李四', '王五'],
'年龄': [25, 30, 28],
'性别': ['男', '男', '女'],
'工资': [5000, 6000, 5500]
})
# 将数据表转换成 Excel 内存对象
wb = pd.ExcelWriter('test.xlsx', engine='openpyxl')
df.to_excel(wb, sheet_name='数据')
# 获取表格的首行单元格
ws = wb.sheets['数据']
first_row_cells = ws[1]
# 定义一个浅蓝色填充
fill = PatternFill(start_color='CCE5FF', end_color='CCE5FF', fill_type='solid')
# 遍历首行单元格,进行填充操作
for cell in first_row_cells:
cell.fill = fill
# 将 Excel 内存对象保存为文件
wb.save()
```
上述代码将通过 Pandas 将数据表转换为 Excel 内存对象,然后使用 openpyxl 库中的 PatternFill 对象定义浅蓝色填充,并通过遍历首行单元格,进行填充操作。最后,将内存对象保存为文件即可。希望能够解决您的问题,如有不妥之处敬请谅解。
用df.style.set_properties把表格的首行填充成浅蓝色
好的,以下是代码示例:
```python
import pandas as pd
# 假设有一个数据框 df
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6], 'C': [7, 8, 9]})
# 用 df.style.set_properties 将首行填充成浅蓝色
styled_df = df.style.set_properties(**{'background-color': 'lightblue'}, subset=pd.IndexSlice[:, [0]])
# 打印输出已填充颜色的数据框
print(styled_df)
```
希望这个回答可以对你有所帮助!如果你有任何其他问题,可以随时问我。
相关推荐
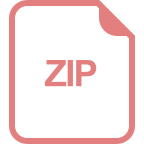
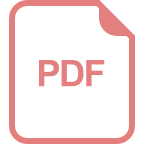
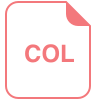
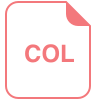
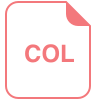
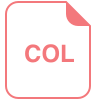
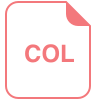







