springboot+vue将html格式的数据转为docx文档并将html样式转化为对应的docx样式的代码
时间: 2024-04-20 19:24:10 浏览: 18
你可以使用Apache POI和Thymeleaf来实现将HTML格式的数据转为docx文档,并将HTML样式转化为对应的docx样式。下面是一个示例代码:
1. 在Spring Boot项目的pom.xml文件中添加以下依赖:
```xml
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>4.1.2</version>
</dependency>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>4.1.2</version>
</dependency>
<dependency>
<groupId>org.thymeleaf</groupId>
<artifactId>thymeleaf-spring5</artifactId>
<version>3.0.12.RELEASE</version>
</dependency>
```
2. 创建一个名为`HtmlToDocxConverter`的类,用于将HTML转为docx:
```java
import org.apache.poi.xwpf.usermodel.XWPFDocument;
import org.apache.poi.xwpf.usermodel.XWPFParagraph;
import org.apache.poi.xwpf.usermodel.XWPFRun;
import org.springframework.stereotype.Component;
import org.thymeleaf.TemplateEngine;
import org.thymeleaf.context.Context;
import java.io.FileOutputStream;
import java.io.IOException;
@Component
public class HtmlToDocxConverter {
private final TemplateEngine templateEngine;
public HtmlToDocxConverter(TemplateEngine templateEngine) {
this.templateEngine = templateEngine;
}
public void convert(String html, String outputPath) throws IOException {
Context context = new Context();
context.setVariable("content", html);
String processedHtml = templateEngine.process("template", context);
XWPFDocument document = new XWPFDocument();
XWPFParagraph paragraph = document.createParagraph();
XWPFRun run = paragraph.createRun();
run.setText(processedHtml);
FileOutputStream out = new FileOutputStream(outputPath);
document.write(out);
out.close();
document.close();
}
}
```
3. 创建一个名为`HtmlToDocxController`的控制器,用于接收HTML数据并调用转换方法:
```java
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RestController;
import java.io.IOException;
@RestController
public class HtmlToDocxController {
private final HtmlToDocxConverter converter;
public HtmlToDocxController(HtmlToDocxConverter converter) {
this.converter = converter;
}
@PostMapping("/convert")
public String convertToDocx(@RequestBody String html) {
try {
converter.convert(html, "output.docx");
return "Conversion successful!";
} catch (IOException e) {
return "Conversion failed: " + e.getMessage();
}
}
}
```
4. 创建一个名为`template.html`的Thymeleaf模板文件,用于保持HTML样式:
```html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>HTML to DOCX</title>
<style>
/* 在这里定义对应的样式 */
</style>
</head>
<body>
<div th:utext="${content}"></div>
</body>
</html>
```
通过发送POST请求到`/convert`端点,并在请求体中包含HTML数据,你将会在项目根目录下生成一个名为`output.docx`的docx文件,其中包含转换后的内容和样式。
这只是一个简单的示例,你可以根据你的需求进行修改和扩展。希望对你有帮助!
相关推荐
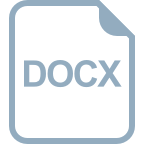














