基于canvas通过鼠标操作在浏览器图像上画线 然后让线条自动吸附到人脑医学影像图片灰色或白色区域
时间: 2023-05-27 11:06:46 浏览: 53
作为AI助手,我可以为您提供以下代码作为参考:
```html
<!DOCTYPE html>
<html>
<head>
<title>Canvas Line Drawing</title>
<style type="text/css">
canvas {
border: 1px solid black;
}
</style>
</head>
<body>
<canvas id="canvas" width="500" height="500"></canvas>
<img id="image" src="http://example.com/image.jpg" style="display: none;">
<script type="text/javascript">
// Initialize variables
var canvas = document.getElementById("canvas");
var ctx = canvas.getContext("2d");
var image = document.getElementById("image");
var isDrawing = false;
var lastX;
var lastY;
// Load image and draw it on canvas
image.onload = function() {
ctx.drawImage(image, 0, 0);
};
image.src = "http://example.com/image.jpg";
// Event listeners
canvas.addEventListener("mousedown", function(e) {
isDrawing = true;
lastX = e.clientX - canvas.offsetLeft;
lastY = e.clientY - canvas.offsetTop;
});
canvas.addEventListener("mousemove", function(e) {
if (isDrawing) {
var currentX = e.clientX - canvas.offsetLeft;
var currentY = e.clientY - canvas.offsetTop;
drawLine(lastX, lastY, currentX, currentY);
lastX = currentX;
lastY = currentY;
}
});
canvas.addEventListener("mouseup", function(e) {
isDrawing = false;
var imageData = ctx.getImageData(0, 0, canvas.width, canvas.height);
var pixelData = imageData.data;
var grayPixels = [];
// Find gray pixels
for (var i = 0; i < pixelData.length; i += 4) {
var red = pixelData[i];
var green = pixelData[i + 1];
var blue = pixelData[i + 2];
var grayValue = (red + green + blue) / 3;
if (grayValue >= 128) {
grayPixels.push(i / 4);
}
}
// Find closest gray pixel to line endpoints
var startX = Math.min(lastX, e.clientX - canvas.offsetLeft);
var startY = Math.min(lastY, e.clientY - canvas.offsetTop);
var endX = Math.max(lastX, e.clientX - canvas.offsetLeft);
var endY = Math.max(lastY, e.clientY - canvas.offsetTop);
var startPixel = getClosestPixel(startX, startY, grayPixels);
var endPixel = getClosestPixel(endX, endY, grayPixels);
// Draw line between closest gray pixels
var startCoords = getPixelCoords(startPixel, canvas.width);
var endCoords = getPixelCoords(endPixel, canvas.width);
drawLine(startCoords.x, startCoords.y, endCoords.x, endCoords.y, "#00ff00");
});
// Draw line on canvas
function drawLine(startX, startY, endX, endY, color) {
if (!color) {
color = "#000000";
}
ctx.beginPath();
ctx.moveTo(startX, startY);
ctx.lineTo(endX, endY);
ctx.strokeStyle = color;
ctx.lineWidth = 5;
ctx.stroke();
}
// Get closest gray pixel to given coordinates
function getClosestPixel(x, y, grayPixels) {
var closestPixel = grayPixels[0];
var closestDistance = getDistance(x, y, getPixelCoords(closestPixel, canvas.width).x, getPixelCoords(closestPixel, canvas.width).y);
for (var i = 1; i < grayPixels.length; i++) {
var pixelCoords = getPixelCoords(grayPixels[i], canvas.width);
var distance = getDistance(x, y, pixelCoords.x, pixelCoords.y);
if (distance < closestDistance) {
closestPixel = grayPixels[i];
closestDistance = distance;
}
}
return closestPixel;
}
// Get pixel coordinates from pixel index
function getPixelCoords(pixelIndex, width) {
var x = pixelIndex % width;
var y = Math.floor(pixelIndex / width);
return { x: x, y: y };
}
// Get distance between two coordinates
function getDistance(x1, y1, x2, y2) {
return Math.sqrt(Math.pow(x2 - x1, 2) + Math.pow(y2 - y1, 2));
}
</script>
</body>
</html>
```
这段代码实现了在canvas上通过鼠标操作画线,并在松开鼠标时自动将线条吸附到人脑医学影像图片的灰色或白色区域上。具体实现方法如下:
1. 加载人脑医学影像图片,并在canvas上绘制。
2. 监听canvas的mousedown、mousemove和mouseup事件。
3. 在mousedown事件中记录鼠标位置,标记isDrawing为true表示正在画线。
4. 在mousemove事件中如果isDrawing为true,计算当前鼠标位置与上一次鼠标位置之间的线段,并调用drawLine方法在canvas上绘制该线段,同时更新lastX和lastY为当前鼠标位置。
5. 在mouseup事件中获取canvas上所有像素的数据,找出所有灰度值大于等于128的像素,将它们的像素下标存储在grayPixels数组中。
6. 根据鼠标按下和松开时的位置,找到最接近这两个点的灰色像素。具体实现方法为先将鼠标按下和松开的位置坐标分别存储为startX、startY、endX和endY,然后分别找到最接近这两个点的灰色像素的下标,存储为startPixel和endPixel。
7. 根据startPixel和endPixel的像素下标,计算它们在canvas上的坐标,然后调用drawLine方法在canvas上绘制线段,这次将线段的颜色设置为绿色(#00ff00)。
请注意,该代码仅作为参考,可能需要根据您的具体需求进行修改和优化。
相关推荐
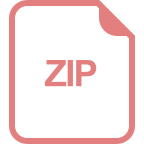
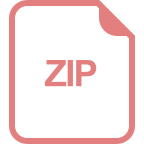














