pyqt5 QLable 如何模拟灯发光动画
时间: 2023-08-11 18:43:49 浏览: 127
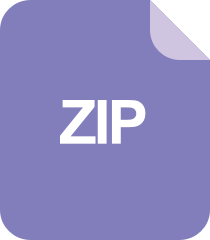
PyQt5:QLable
要实现模拟灯发光的动画效果,可以使用 QTimer 和 QPropertyAnimation 类来控制 QLabel 的亮点动画。
具体步骤如下:
1. 创建一个 QTimer 对象,用于定时触发亮点动画。
```
timer = QTimer()
```
2. 创建一个 QPropertyAnimation 对象,用于控制 QLabel 的亮点动画。
```
animation = QPropertyAnimation(label, b"pos")
```
其中,`label` 表示要进行动画的 QLabel 对象,`b"pos"` 表示对 QLabel 的位置属性进行动画。
3. 设置 QTimer 的定时器间隔和启动动画。
```
timer.setInterval(2000)
timer.timeout.connect(animation.start)
timer.start()
```
其中,`setInterval` 方法设置定时器的间隔时间,`timeout` 信号连接到 `start` 方法,表示定时器超时时启动动画。
4. 设置 QPropertyAnimation 的动画属性和值。
```
animation.setDuration(1000)
animation.setStartValue(QPoint(0, 0))
animation.setEndValue(QPoint(20, 20))
```
其中,`setDuration` 方法设置动画的持续时间,`setStartValue` 方法设置动画的起始位置,`setEndValue` 方法设置动画的结束位置。
5. 在动画结束时,更新 QLabel 的亮点图像。
```
animation.finished.connect(lambda: label.setPixmap(pixmap))
```
其中,`finished` 信号连接到一个 lambda 函数,该函数在动画结束时更新 QLabel 的亮点图像。
最终的代码如下:
```
from PyQt5.QtCore import QPoint, QTimer, QPropertyAnimation
from PyQt5.QtGui import QPixmap
from PyQt5.QtWidgets import QApplication, QLabel
app = QApplication([])
label = QLabel("Hello, World!")
label.setStyleSheet("background-color: #333333; border: 2px solid #cccccc; border-radius: 10px; box-shadow: 0px 0px 10px 5px #ffffff;")
pixmap = QPixmap("light.png").scaled(20, 20)
label.setPixmap(pixmap)
timer = QTimer()
animation = QPropertyAnimation(label, b"pos")
animation.setDuration(1000)
animation.setStartValue(QPoint(0, 0))
animation.setEndValue(QPoint(20, 20))
animation.finished.connect(lambda: label.setPixmap(pixmap))
timer.setInterval(2000)
timer.timeout.connect(animation.start)
timer.start()
label.show()
app.exec_()
```
以上代码会显示一个带有模拟灯发光动画效果的 QLabel 控件,并在控件的中心位置添加一个白色的亮点。每隔 2 秒钟,亮点会从左上角移动到右下角,并在移动结束时更新亮点图像。
阅读全文
相关推荐
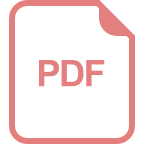
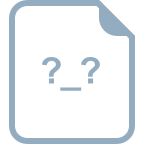
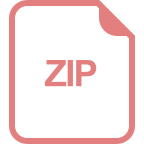
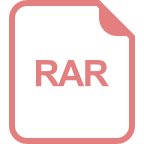
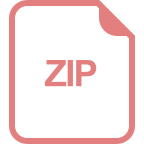
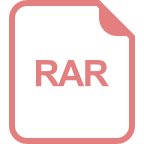
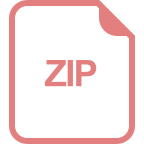
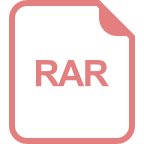
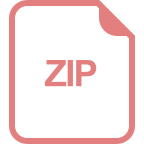
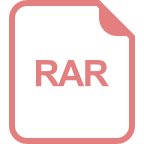
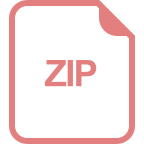