1. Write a LC-3 subroutine for printing “Hello” for N times. Write a main program to input N from the keyboard, call the subroutine and then output “Hello” for N times.
时间: 2024-02-01 09:02:13 浏览: 25
Here is the LC-3 subroutine for printing "Hello" for N times:
```
; Subroutine to print "Hello" for N times
; Input: R0 = N (number of times to print)
; Output: None
HELLO_SUB:
LD R1, HELLO_STR ; Load address of "Hello" string
AND R0, R0, #0 ; Clear R0
ADD R0, R0, #10 ; Set R0 to 10
LOOP:
BRz END_LOOP ; If R0 is zero, exit loop
ADD R0, R0, #-1 ; Decrement R0
TRAP x22 ; Output "Hello"
ADD R6, R6, #-1 ; Decrement stack pointer
ST R1, R6, #0 ; Save address of "Hello" string on stack
BRnzp LOOP ; Continue looping
END_LOOP:
RET ; Return from subroutine
; String "Hello"
HELLO_STR .STRINGZ "Hello"
```
And here is the main program to input N from the keyboard, call the subroutine, and output "Hello" for N times:
```
; Main program to call HELLO_SUB and output "Hello" for N times
.ORIG x3000
; Prompt user for N
LEA R0, PROMPT_N
PUTS
GETC
OUT
IN
PUTS
; Convert input to decimal
LD R1, ASCII_ZERO
ADD R2, R0, #-10 ; Subtract 10 to convert from ASCII
ADD R2, R2, R1
ADD R0, R2, #0
; Call HELLO_SUB
JSR HELLO_SUB
; Output "Hello" for N times
LOOP:
LD R1, HELLO_STR
TRAP x22
ADD R0, R0, #-1
BRnzp LOOP
; String "Enter N: "
PROMPT_N .STRINGZ "Enter N: "
; ASCII value of zero
ASCII_ZERO .FILL x0030
.END
```
The program prompts the user to enter N, converts the input from ASCII to decimal, calls HELLO_SUB, and then outputs "Hello" for N times.
相关推荐
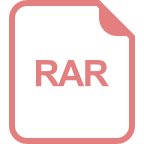

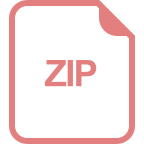
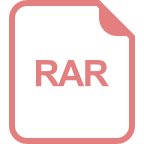
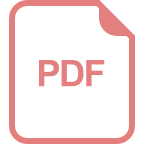
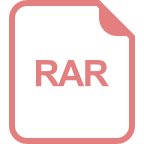
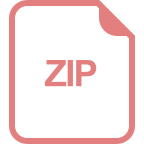
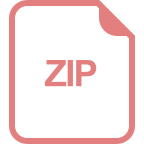