p ython五子棋游戏代码
时间: 2023-07-09 08:43:16 浏览: 46
下面是一个简单的 Python 五子棋游戏代码,供参考:
```python
import numpy as np
# 定义棋盘大小
ROW = 15
COL = 15
# 定义棋子类型
EMPTY = 0
BLACK = 1
WHITE = 2
# 定义赢法数组
win_array = np.zeros((ROW, COL, 572), dtype=np.bool)
# 初始化赢法数组
count = 0
# 横向赢法
for i in range(ROW):
for j in range(COL-4):
for k in range(5):
win_array[i, j+k, count] = True
count += 1
# 纵向赢法
for i in range(ROW-4):
for j in range(COL):
for k in range(5):
win_array[i+k, j, count] = True
count += 1
# 斜向赢法 (\)
for i in range(ROW-4):
for j in range(COL-4):
for k in range(5):
win_array[i+k, j+k, count] = True
count += 1
# 斜向赢法 (/)
for i in range(4, ROW):
for j in range(COL-4):
for k in range(5):
win_array[i-k, j+k, count] = True
count += 1
# 定义游戏类
class Game:
def __init__(self):
self.board = np.zeros((ROW, COL), dtype=np.int)
self.current_player = BLACK
self.gameover = False
# 判断是否胜利
def is_win(self, player):
for i in range(572):
if np.all(self.board[win_array[:, :, i]] == player):
return True
return False
# 判断是否平局
def is_draw(self):
return np.all(self.board != EMPTY)
# 落子
def move(self, i, j):
if self.gameover or self.board[i, j] != EMPTY:
return False
self.board[i, j] = self.current_player
if self.is_win(self.current_player):
self.gameover = True
print("Player %d wins!" % self.current_player)
elif self.is_draw():
self.gameover = True
print("Draw!")
else:
self.current_player = WHITE if self.current_player == BLACK else BLACK
return True
# 打印棋盘
def print_board(self):
print(" ", end="")
for i in range(COL):
print("%2d" % i, end="")
print()
for i in range(ROW):
print("%2d" % i, end="")
for j in range(COL):
if self.board[i, j] == BLACK:
print(" ○", end="")
elif self.board[i, j] == WHITE:
print(" ●", end="")
else:
print(" +", end="")
print()
# 测试代码
if __name__ == '__main__':
game = Game()
game.print_board()
while not game.gameover:
i, j = map(int, input("Player %d's turn (row, col): " % game.current_player).split())
game.move(i, j)
game.print_board()
```
运行此代码可以开始玩五子棋游戏。
相关推荐
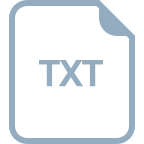
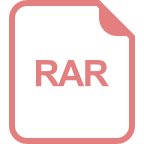












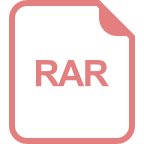