vue3 ref 数组
时间: 2023-11-07 09:00:09 浏览: 150
在 Vue3 中,可以使用 `ref` 创建响应式数据。但是 `ref` 只能创建单一的响应式数据,而不能直接创建响应式数组。如果要创建响应式数组,可以使用 `reactive` 或 `ref` 结合 `shallowRef`。
1. 使用 `reactive` 创建响应式数组
```javascript
import { reactive } from 'vue'
const state = reactive({
arr: [1, 2, 3]
})
console.log(state.arr) // [1, 2, 3]
state.arr.push(4)
console.log(state.arr) // [1, 2, 3, 4]
```
2. 使用 `ref` 和 `shallowRef` 创建响应式数组
```javascript
import { ref, shallowRef } from 'vue'
const arr = ref([1, 2, 3])
console.log(arr.value) // [1, 2, 3]
arr.value.push(4)
console.log(arr.value) // [1, 2, 3, 4]
// 使用 shallowRef 创建响应式数组
const arr2 = shallowRef([1, 2, 3])
console.log(arr2.value) // [1, 2, 3]
arr2.value.push(4)
console.log(arr2.value) // [1, 2, 3, 4]
```
使用 `ref` 和 `shallowRef` 创建响应式数组时,需要注意以下几点:
- `ref` 创建的响应式数组需要通过 `arr.value` 访问。
- `ref` 创建的响应式数组不支持数组方法的响应式更新,如 `arr.value.push(1)` 不会触发视图更新,需要手动调用 `arr.value = [...arr.value, 1]` 或 `arr.value.splice(index, 0, item)`。
- `shallowRef` 创建的响应式数组只会对数组本身进行响应式处理,而不会对数组里的对象进行响应式处理。如果数组里的对象需要进行响应式处理,需要使用 `reactive`。
阅读全文
相关推荐
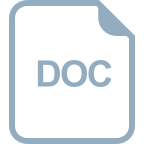
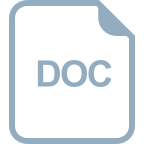
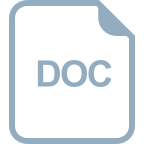















