qt中使用C++语言在QCombobox中添加一个Button,点击这个Button实现关闭当前选中的item
时间: 2024-02-23 14:00:17 浏览: 34
在Qt中,可以通过自定义一个QComboBox的ItemDelegate来实现在QComboBox中添加一个Button,并且点击这个Button实现关闭当前选中的item。具体步骤如下:
1. 新建一个类,继承自QStyledItemDelegate,重写createEditor、updateEditorGeometry和paint方法。其中,createEditor方法用于创建一个QWidget对象作为ItemDelegate的编辑器,updateEditorGeometry方法用于更新编辑器的位置和大小,paint方法用于绘制ItemDelegate的内容。
2. 在createEditor方法中创建一个QPushButton对象,并且将其与QComboBox的currentIndexChanged信号连接起来。在这个槽函数中,可以通过QComboBox的currentIndex方法获取当前选中的item的索引,然后通过QComboBox的removeItem方法将其从QComboBox中删除。
3. 将自定义的ItemDelegate对象设置为QComboBox的ItemDelegate,即可在QComboBox中添加一个Button。
下面是示例代码:
```
class ComboBoxDelegate : public QStyledItemDelegate
{
public:
QWidget *createEditor(QWidget *parent, const QStyleOptionViewItem &option, const QModelIndex &index) const override
{
QPushButton *button = new QPushButton("Close", parent);
connect(button, &QPushButton::clicked, this, [=](){
QComboBox *comboBox = qobject_cast<QComboBox*>(parent);
if(comboBox)
{
int currentIndex = comboBox->currentIndex();
comboBox->removeItem(currentIndex);
}
});
return button;
}
void updateEditorGeometry(QWidget *editor, const QStyleOptionViewItem &option, const QModelIndex &index) const override
{
editor->setGeometry(option.rect);
}
void paint(QPainter *painter, const QStyleOptionViewItem &option, const QModelIndex &index) const override
{
painter->drawText(option.rect, index.data().toString());
}
};
//在使用QComboBox的地方设置ItemDelegate
QComboBox *comboBox = new QComboBox(this);
comboBox->setItemDelegate(new ComboBoxDelegate());
```
相关推荐
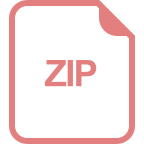
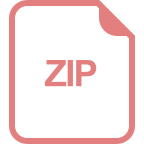















