tkinter 文件选择器 点击触发程序
时间: 2023-11-17 16:07:07 浏览: 101
好的,您可以通过以下代码实现tkinter文件选择器并点击触发程序:
```python
import tkinter as tk
from tkinter import filedialog
# 创建tkinter窗口
root = tk.Tk()
root.withdraw()
# 弹出文件选择框
file_path = filedialog.askopenfilename()
# 如果用户选择了文件,则执行相应的程序
if file_path:
# 执行程序
print("您选择的文件是:", file_path)
else:
print("您未选择任何文件!")
```
上述代码中,我们首先创建了一个tkinter窗口并隐藏它(`root.withdraw()`),然后使用`filedialog.askopenfilename()`弹出文件选择对话框。如果用户选择了文件,则可以在`if file_path:`语句块中执行相应的程序。否则,可以在`else:`语句块中给出相应的提示。
相关问题
tkinter文件选择对话框
### Tkinter 文件选择对话框 示例代码
为了创建一个简单的文件选择对话框,可以使用 `tkinter` 和其子模块 `filedialog` 来实现这一功能。下面是一段完整的 Python 代码示例,用于展示如何通过 Tkinter 实现基本的文件选择对话框:
```python
import tkinter as tk
from tkinter import filedialog
root = tk.Tk()
root.title("文件选择对话框实例")
def select_file():
filepath = filedialog.askopenfilename(
title="请选择文件",
initialdir=r"D:\Temp", # 设置初始目录
filetypes=[("Image files", "*.jpg *.png")] # 可选参数:限定可选文件类型
)
if filepath:
print(f"所选文件路径为: {filepath}")
button = tk.Button(root, text="点击选择文件", command=select_file)
button.pack(pady=20)
root.mainloop()
```
此段代码定义了一个带有按钮的小型图形界面应用程序[^1]。当用户单击该按钮时,会触发 `askopenfilename()` 方法显示文件选择器窗口,在其中可以选择特定类型的图像文件(如 `.jpg`,`.png`),并且打印出选定文件的具体位置。
对于更复杂的需求,还可以考虑允许用户一次选取多个文件或仅限于某类扩展名的文档等特性。这可以通过调整传递给 `askopenfilename()` 函数的不同选项来完成[^3]。
tkinter 文件管理器
Tkinter是Python的一个标准库,用于创建图形用户界面(GUI)。它本身并不内置文件管理器功能,但你可以使用Tkinter构建一个简单的文件浏览器或选择器来帮助用户浏览和选择文件。以下是一个基本的例子,展示如何使用Tkinter的`filedialog`模块来实现文件选择:
```python
import tkinter as tk
from tkinter import filedialog
def browse_files():
# 弹出文件选择对话框
file_path = filedialog.askopenfilename()
if file_path: # 如果用户选择了文件
print(f"用户选择了文件: {file_path}")
# 创建主窗口
root = tk.Tk()
root.title("File Manager")
# 创建一个按钮,点击后触发文件选择
browse_button = tk.Button(root, text="浏览并选择文件", command=browse_files)
browse_button.pack()
# 运行主循环
root.mainloop()
```
在这个例子中,当你点击“浏览并选择文件”按钮时,会弹出一个对话框,用户可以在其中选择一个文件。这个对话框可以用来打开、保存文件或者执行其他与文件操作相关的任务。
阅读全文
相关推荐





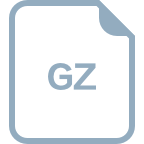









