#include<iostream> #include<cstring> using namespace std; void strmcpy(char* s,char* t,int m); int main(){ int repeat,m; char t[100],s[100]; cin>>repeat; for(int i=0;i<repeat;i++){ cin.getline(t,100); cin>>m; strmcpy(s,t,m); } } void strmcpy(char* s,char* t,int m){ int len=strlen(t); cout<<len<<endl; if(m>len||m<1){ cout<<"error input"<<endl; } else{ strcpy(s,&t[m-1]); cout<<s<<endl; } }这串代码那里出了问题致使不能输出上述问题正确结果
时间: 2024-03-23 18:39:45 浏览: 12
这段代码中存在两个问题:
1. 在输入字符串之前,需要先将之前输入的换行符读取掉,否则会影响输入字符串的正确性。可以在读取重复次数之后添加一行 `cin.get();` 来解决。
2. 在 `strmcpy` 函数中,使用 `strcpy` 函数将字符串复制到指定位置可能会导致越界访问,因为 `s` 数组并没有足够的空间来存放复制后的字符串。可以使用指针运算来实现复制操作,例如可以使用 `s = &t[m-1];` 来直接让 `s` 指向 `t` 数组中指定位置的字符。
修改后的代码如下:
```c++
#include<iostream>
#include<cstring>
using namespace std;
void strmcpy(char* s,char* t,int m);
int main(){
int repeat,m;
char t[100],s[100];
cin>>repeat;
cin.get(); // 读取换行符
for(int i=0;i<repeat;i++){
cin.getline(t,100);
cin>>m;
strmcpy(s,t,m);
}
}
void strmcpy(char* s,char* t,int m){
int len=strlen(t);
cout<<len<<endl;
if(m>len||m<1){
cout<<"error input"<<endl;
}
else{
s = &t[m-1];
cout<<s<<endl;
}
}
```
相关推荐
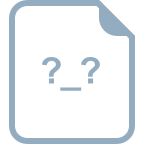
















