解释每条语句的作用import json import csv import os def process_json_file(filepath): #解码json文件 # f = open(filepath,mode='r',encoding='utf-8') # city_list = json.load(f) # return city_list with open(filepath,mode='r',encoding='utf-8') as f: city_list = json.load(f) print(city_list) def process_csv_file(filepath): #处理csv文件 with open(filepath, mode='r', encoding='utf-8', newline='') as f: reader = csv.reader(f) for row in reader: print(', '.join(row)) # 将列表里每一行的函数用‘, ’连接起来 def main(): #主函数 filepath = input('请输入文件名称:') filename, file_ext = os.path.splitext(filepath) if file_ext == '.json': # json文件 process_json_file(filepath) elif file_ext == '.csv': # csv文件 process_csv_file(filepath) else: print('不支持的文件格式!') if __name__ == '__main__': main()
时间: 2023-06-20 12:03:44 浏览: 67
这段代码的作用是读取一个文件,根据文件的扩展名来判断文件类型,如果是json文件则解码json文件,如果是csv文件则处理csv文件,最后输出文件内容。
具体解释如下:
- import json import csv import os
导入需要用到的模块:json、csv、os。
- def process_json_file(filepath): #解码json文件
定义一个函数,用于解码json文件。
- with open(filepath,mode='r',encoding='utf-8') as f: city_list = json.load(f) print(city_list)
打开文件,使用json模块的load()方法将文件内容解码为Python对象,并将结果存储在变量city_list中,最后输出city_list的内容。
- def process_csv_file(filepath): #处理csv文件
定义一个函数,用于处理csv文件。
- with open(filepath, mode='r', encoding='utf-8', newline='') as f: reader = csv.reader(f) for row in reader: print(', '.join(row))
打开文件,使用csv模块的reader()方法将文件内容读取为一个迭代器对象reader,然后遍历reader中的每一行数据,并将每一行中的数据用逗号分隔连接起来,并输出结果。
- def main(): #主函数 filepath = input('请输入文件名称:') filename, file_ext = os.path.splitext(filepath) if file_ext == '.json': # json文件 process_json_file(filepath) elif file_ext == '.csv': # csv文件 process_csv_file(filepath) else: print('不支持的文件格式!')
定义一个主函数,首先获取用户输入的文件名,然后使用os模块的splitext()方法来获取文件的扩展名,根据扩展名来判断文件类型,如果是json文件则调用process_json_file()函数处理,如果是csv文件则调用process_csv_file()函数处理,否则输出不支持的文件格式。
- if __name__ == '__main__': main()
判断代码是否在主程序中运行,如果是则调用main()函数。
相关推荐
















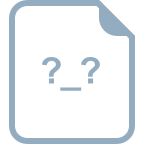
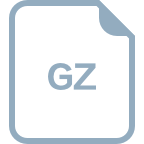