Write comprehensive program in Java which include all the common features of OOP with displaying results of variables using the methods
时间: 2024-04-09 20:28:01 浏览: 94
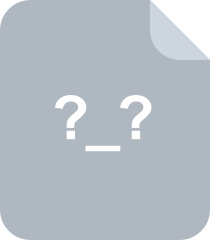
使用Java实现面向对象编程(Java OOP)
Certainly! Here's an example of a comprehensive Java program that includes common features of object-oriented programming (OOP) and displays the results of variables using methods:
```java
// Define a superclass called Shape
class Shape {
// Instance variables
protected String color;
// Constructor
public Shape(String color) {
this.color = color;
}
// Getter and setter methods
public String getColor() {
return color;
}
public void setColor(String color) {
this.color = color;
}
// Method to calculate area (to be overridden by subclasses)
public double calculateArea() {
return 0.0;
}
// Display method
public void displayInfo() {
System.out.println("Color: " + color);
System.out.println("Area: " + calculateArea());
}
}
// Define a subclass called Circle
class Circle extends Shape {
// Instance variable
private double radius;
// Constructor
public Circle(String color, double radius) {
super(color);
this.radius = radius;
}
// Getter and setter methods
public double getRadius() {
return radius;
}
public void setRadius(double radius) {
this.radius = radius;
}
// Override calculateArea() method from Shape class
@Override
public double calculateArea() {
return Math.PI * radius * radius;
}
}
// Define a subclass called Rectangle
class Rectangle extends Shape {
// Instance variables
private double length;
private double width;
// Constructor
public Rectangle(String color, double length, double width) {
super(color);
this.length = length;
this.width = width;
}
// Getter and setter methods
public double getLength() {
return length;
}
public void setLength(double length) {
this.length = length;
}
public double getWidth() {
return width;
}
public void setWidth(double width) {
this.width = width;
}
// Override calculateArea() method from Shape class
@Override
public double calculateArea() {
return length * width;
}
}
// Main class
public class Main {
public static void main(String[] args) {
// Create instances of Circle and Rectangle
Circle circle = new Circle("Red", 5.0);
Rectangle rectangle = new Rectangle("Blue", 4.0, 6.0);
// Display shape information
System.out.println("Circle:");
circle.displayInfo();
System.out.println("\nRectangle:");
rectangle.displayInfo();
}
}
```
In this example, we have a superclass called `Shape` with an instance variable `color` and methods for getting and setting the color, calculating the area (to be overridden by subclasses), and displaying the shape information.
We also have two subclasses, `Circle` and `Rectangle`, that inherit from the `Shape` superclass. These subclasses have their own unique instance variables (`radius` for `Circle` and `length` and `width` for `Rectangle`), as well as methods for getting and setting these variables. The subclasses override the `calculateArea()` method to provide their specific area calculation formulas.
In the `Main` class, we create instances of `Circle` and `Rectangle`, passing in the color and dimensions as arguments. We then call the `displayInfo()` method on each instance to display the shape's color and calculated area.
This program demonstrates inheritance, polymorphism (through method overriding), encapsulation, and the concept of objects and classes in OOP.
阅读全文
相关推荐
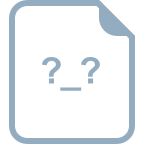
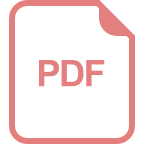
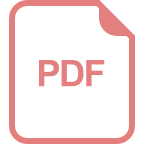
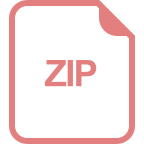
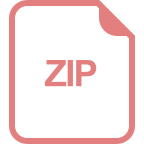
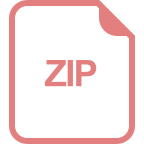
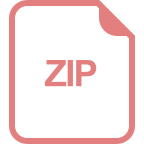
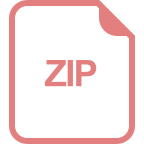
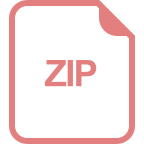
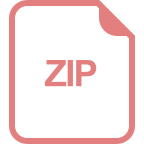
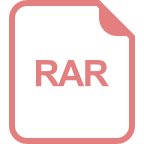
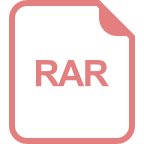
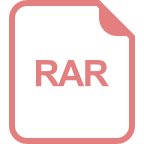
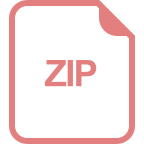
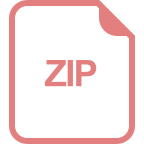
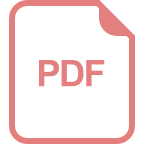