3.编写类模版,实现顺序栈的功能: (1)栈的初始化 (2)判断栈是否为空; (3)判断栈是否为满; (4)入栈; (5)出栈; (6)销毁。 并写出调用此类模板的完整程序。
时间: 2024-02-17 14:03:27 浏览: 76
以下是实现顺序栈的类模板的代码:
```cpp
template <class T>
class SeqStack{
private:
T* data; // 存储栈元素的数组
int top; // 栈顶指针
int maxSize; // 栈的最大容量
public:
SeqStack(int size=100) : maxSize(size), top(-1){
data = new T[maxSize];
}
~SeqStack(){
delete[] data;
}
bool isEmpty(){
return top == -1;
}
bool isFull(){
return top == maxSize - 1;
}
bool push(const T& x){
if(isFull()) return false;
data[++top] = x;
return true;
}
bool pop(T& x){
if(isEmpty()) return false;
x = data[top--];
return true;
}
void clear(){
top = -1;
}
};
```
下面是一个调用此类模板的完整程序示例:
```cpp
#include<iostream>
#include<string>
using namespace std;
int main(){
SeqStack<int> s; // 定义一个存储 int 类型的栈
int x;
for(int i=1; i<=5; i++){
s.push(i);
}
while(!s.isEmpty()){
s.pop(x);
cout << x << " ";
}
cout << endl;
SeqStack<string> s2(10); // 定义一个存储 string 类型的栈,最大容量为 10
string str;
while(cin >> str && str != "quit"){
s2.push(str);
}
while(!s2.isEmpty()){
s2.pop(str);
cout << str << " ";
}
cout << endl;
return 0;
}
```
此程序演示了如何使用 SeqStack 类模板来实现一个存储 int 和 string 类型元素的栈,并进行入栈、出栈、判断栈是否为空等操作。
阅读全文
相关推荐
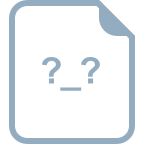
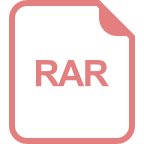
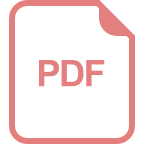
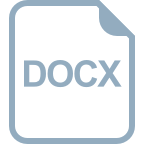
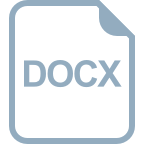
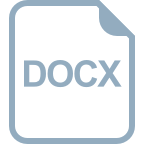
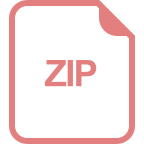