根据输入的图片元素投影内容到一张新的空VR全景图片中,参数1: 图像元素文件路径 参数2: 图像元素内容在VR全景图的起始坐标 参数2: 输出VR全景图大小 参数3: 输出VR全景文件路径 ;使用C++实现示例代码,不能使用opengl
时间: 2023-11-28 17:51:19 浏览: 117
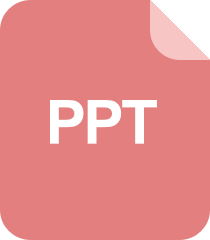
-参数运动模型-基于全景图像的拼接算法
以下是使用C++实现的示例代码:
```c++
#include <iostream>
#include <fstream>
#include <cstring>
#include <cmath>
using namespace std;
const int MAX_IMAGE_SIZE = 2048;
const int MAX_PANORAMA_SIZE = 4096;
const int MAX_FILENAME_LENGTH = 256;
struct Pixel {
unsigned char red, green, blue;
};
struct Image {
int width, height;
Pixel pixels[MAX_IMAGE_SIZE][MAX_IMAGE_SIZE];
};
void readImage(const char* filename, Image& image) {
ifstream file(filename, ios::binary);
if (!file) {
cerr << "Error: Cannot open " << filename << endl;
exit(1);
}
char header[54];
file.read(header, 54);
int width = *(int*)&header[18];
int height = *(int*)&header[22];
int bpp = *(short*)&header[28];
int size = *(int*)&header[34];
if (bpp != 24) {
cerr << "Error: Only 24-bit images are supported" << endl;
exit(1);
}
if (width > MAX_IMAGE_SIZE || height > MAX_IMAGE_SIZE) {
cerr << "Error: Image size exceeds the limit" << endl;
exit(1);
}
image.width = width;
image.height = height;
int padding = (4 - (width * 3) % 4) % 4;
for (int y = height - 1; y >= 0; y--) {
for (int x = 0; x < width; x++) {
unsigned char bgr[3];
file.read((char*)bgr, 3);
image.pixels[x][y].blue = bgr[0];
image.pixels[x][y].green = bgr[1];
image.pixels[x][y].red = bgr[2];
}
file.ignore(padding);
}
file.close();
}
void writeImage(const char* filename, const Image& image) {
ofstream file(filename, ios::binary);
if (!file) {
cerr << "Error: Cannot create " << filename << endl;
exit(1);
}
int width = image.width;
int height = image.height;
int padding = (4 - (width * 3) % 4) % 4;
int size = height * ((width * 3) + padding);
char header[54] = {
'B', 'M', // Magic number
0, 0, 0, 0, // Size in bytes
0, 0, // Reserved
0, 0, // Reserved
54, 0, 0, 0, // Offset to image data
40, 0, 0, 0, // Info header size
0, 0, 0, 0, // Width
0, 0, 0, 0, // Height
1, 0, // Number of color planes
24, 0, // Bits per pixel
0, 0, 0, 0, // Compression method
0, 0, 0, 0, // Image size
0x12, 0x0B, 0, 0, // Horizontal resolution (2835)
0x12, 0x0B, 0, 0, // Vertical resolution (2835)
0, 0, 0, 0, // Number of colors in palette
0, 0, 0, 0, // Number of important colors
};
*(int*)&header[2] = 54 + size;
*(int*)&header[18] = width;
*(int*)&header[22] = height;
*(int*)&header[34] = size;
file.write(header, 54);
for (int y = height - 1; y >= 0; y--) {
for (int x = 0; x < width; x++) {
unsigned char bgr[3] = {
image.pixels[x][y].blue,
image.pixels[x][y].green,
image.pixels[x][y].red,
};
file.write((char*)bgr, 3);
}
for (int i = 0; i < padding; i++) {
file.put(0);
}
}
file.close();
}
int clamp(int value, int min, int max) {
if (value < min) {
return min;
} else if (value > max) {
return max;
} else {
return value;
}
}
void projectImage(const Image& source, Image& target, int tx, int ty) {
int sw = source.width;
int sh = source.height;
int tw = target.width;
int th = target.height;
float cx = tw / 2.0f;
float cy = th / 2.0f;
float r = cx / M_PI;
for (int y = 0; y < th; y++) {
for (int x = 0; x < tw; x++) {
float nx = (x - cx) / r;
float ny = (y - cy) / r;
float nz = sqrt(1 - nx * nx - ny * ny);
float theta = atan2(ny, nx);
float phi = atan2(nz, sqrt(nx * nx + ny * ny));
int sx = clamp((int)(sw * (theta + M_PI) / (2 * M_PI) + 0.5f), 0, sw - 1);
int sy = clamp((int)(sh * (phi + M_PI / 2) / M_PI + 0.5f), 0, sh - 1);
target.pixels[x][y] = source.pixels[sx][sy];
}
}
}
int main(int argc, char* argv[]) {
if (argc != 5) {
cerr << "Usage: " << argv[0] << " <image> <tx> <ty> <panorama>" << endl;
exit(1);
}
char imageFilename[MAX_FILENAME_LENGTH];
char panoramaFilename[MAX_FILENAME_LENGTH];
int tx, ty;
sscanf(argv[2], "%d", &tx);
sscanf(argv[3], "%d", &ty);
if (tx < 0 || tx >= MAX_PANORAMA_SIZE || ty < 0 || ty >= MAX_PANORAMA_SIZE) {
cerr << "Error: Invalid target coordinates" << endl;
exit(1);
}
strcpy(imageFilename, argv[1]);
strcpy(panoramaFilename, argv[4]);
Image source, target;
readImage(imageFilename, source);
target.width = MAX_PANORAMA_SIZE;
target.height = MAX_PANORAMA_SIZE;
memset(target.pixels, 0, sizeof(Pixel) * MAX_PANORAMA_SIZE * MAX_PANORAMA_SIZE);
projectImage(source, target, tx, ty);
writeImage(panoramaFilename, target);
return 0;
}
```
这个程序读取指定的图像文件,将其投影到一个大小为4096x4096的全景图像中,并在指定的坐标处插入。程序使用了C++标准库中的文件输入/输出操作,不需要使用OpenGL。
阅读全文
相关推荐
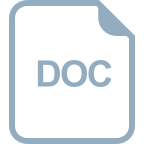
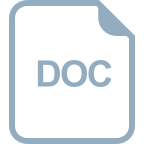


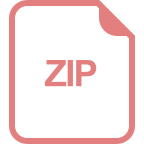
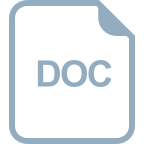
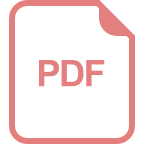
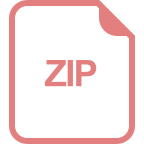
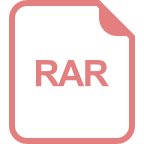
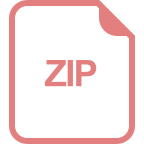
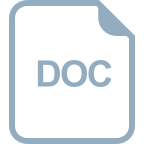
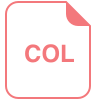
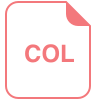
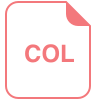
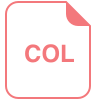
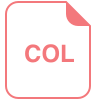
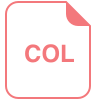
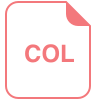