springboot实现文件导入导出
时间: 2023-12-21 07:04:31 浏览: 124
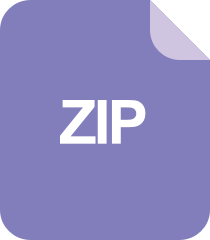
spring-boot导入导出
1. 文件导入
Spring Boot提供了多种方式来实现文件导入,以下是其中一种方式:
1) 定义上传文件的接口:
```
@PostMapping("/upload")
public ResponseEntity<String> upload(@RequestParam("file") MultipartFile file) {
try {
// Save the file to a temporary directory
File tempFile = File.createTempFile("temp", file.getOriginalFilename());
file.transferTo(tempFile);
// Process the file
// ...
return ResponseEntity.ok("File uploaded successfully");
} catch (IOException e) {
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).body(e.getMessage());
}
}
```
2) 在前端页面中添加上传文件的表单:
```
<form method="post" enctype="multipart/form-data" action="/upload">
<input type="file" name="file"/>
<button type="submit">Upload</button>
</form>
```
3) 在应用程序中添加文件上传的配置:
```
@Configuration
public class FileUploadConfig {
@Bean
public MultipartConfigElement multipartConfigElement() {
MultipartConfigFactory factory = new MultipartConfigFactory();
factory.setMaxFileSize(DataSize.ofMegabytes(10));
factory.setMaxRequestSize(DataSize.ofMegabytes(10));
return factory.createMultipartConfig();
}
}
```
2. 文件导出
Spring Boot也提供了多种方式来实现文件导出,以下是其中一种方式:
1) 定义文件导出的接口:
```
@GetMapping("/download")
public ResponseEntity<Resource> download() {
// Generate the file content
String content = "Hello, world!";
ByteArrayResource resource = new ByteArrayResource(content.getBytes());
// Return the file as a downloadable resource
return ResponseEntity.ok()
.header(HttpHeaders.CONTENT_DISPOSITION, "attachment;filename=example.txt")
.contentType(MediaType.TEXT_PLAIN)
.contentLength(content.getBytes().length)
.body(resource);
}
```
2) 在前端页面中添加下载文件的链接:
```
<a href="/download">Download</a>
```
以上就是Spring Boot实现文件导入导出的简单示例。
阅读全文
相关推荐
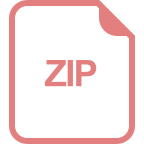
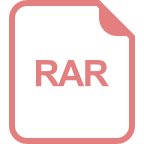
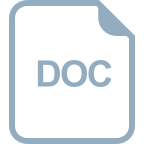

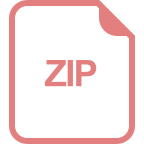
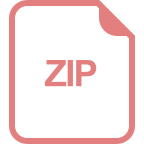











