丁莹渐晕标定代码c++
时间: 2023-10-16 16:31:11 浏览: 40
丁莹渐晕标定是一种基于图像的相机标定方法,可以校准相机的内部参数和外部参数。以下是一个简单的 C++ 实现:
```c++
#include <iostream>
#include <vector>
#include <opencv2/opencv.hpp>
using namespace std;
using namespace cv;
// 丁莹渐晕标定函数
void calibration(vector<vector<Point2f>> imagePoints,
vector<vector<Point3f>> objectPoints,
Size imageSize, Mat &cameraMatrix, Mat &distCoeffs) {
// 初始化相机参数估计器
vector<Mat> rvecs, tvecs;
cameraMatrix = Mat::eye(3, 3, CV_64F);
distCoeffs = Mat::zeros(8, 1, CV_64F);
// 进行标定
double rms = calibrateCamera(objectPoints, imagePoints, imageSize,
cameraMatrix, distCoeffs, rvecs, tvecs);
// 输出标定结果
cout << "RMS error: " << rms << endl;
cout << "Camera matrix: " << endl << cameraMatrix << endl;
cout << "Distortion coefficients: " << endl << distCoeffs << endl;
}
int main() {
// 读取标定图像
vector<vector<Point2f>> imagePoints;
vector<vector<Point3f>> objectPoints;
Size boardSize(6, 9);
float squareSize = 1.f;
vector<String> filenames;
glob("calibration_images/*.jpg", filenames);
for (int i = 0; i < filenames.size(); ++i) {
Mat image = imread(filenames[i]);
if (image.empty()) continue;
vector<Point2f> corners;
bool found = findChessboardCorners(image, boardSize, corners);
if (found) {
Mat gray;
cvtColor(image, gray, COLOR_BGR2GRAY);
cornerSubPix(gray, corners, Size(11, 11), Size(-1, -1),
TermCriteria(TermCriteria::EPS + TermCriteria::MAX_ITER, 30, 0.1));
drawChessboardCorners(image, boardSize, corners, found);
imshow("image", image);
waitKey(0);
imagePoints.push_back(corners);
vector<Point3f> obj;
for (int j = 0; j < boardSize.height; ++j)
for (int k = 0; k < boardSize.width; ++k)
obj.push_back(Point3f(k * squareSize, j * squareSize, 0));
objectPoints.push_back(obj);
}
}
// 进行标定
Mat cameraMatrix, distCoeffs;
calibration(imagePoints, objectPoints, Size(1920, 1080), cameraMatrix, distCoeffs);
return 0;
}
```
在这个实现中,我们首先使用 `glob` 函数读取标定图像,然后使用 OpenCV 的 `findChessboardCorners` 函数检测棋盘格角点,在检测到角点的情况下,使用 `cornerSubPix` 函数对角点进行亚像素精度的优化,并将优化后的角点和对应的物理坐标保存到 `imagePoints` 和 `objectPoints` 中。最后,调用 `calibration` 函数进行相机标定,并输出标定结果。
相关推荐
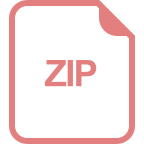
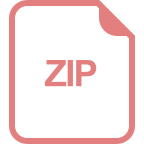
















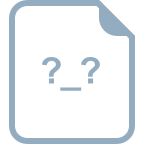