在停车场收费系统中,根据车型的不同按不同的单价和计费方式收取不同的停车费,程序设计如下: 有一个 Vehicle类,有如下属性和方法: (1) price(单价)和minute(分钟) (2) 构造函数:带2个输入参数:price 和minute; (3)price 和minute的get/set方法 (4) float computeFee()计算停车费 Vehicle类和主类代码已经给出,你结合给出的代码,设计Vehicle的子类:Car和Bus。 Bus类 有私有成员:座位数 int seats 两个子类都重写继承的方法computeFee()来计算停车费: 轿车Car:每小时收费price元,未达1小时但超过30分钟按一小时费用计算,30分钟以内(包括30分钟)不收费 客车Bus:每小时收费price元,30分钟以内(包括30分钟)按半小时费用计费,未达1小时但超过30分钟按1小时费用计算 (提示 h=getMinute()/60 求得停车小时数,f=getMinute()%60求得超过的分钟数。对应客车Bus计费:如果f值为0,计费按h*price,30分钟以内(包括30分钟) 表示0<f<=30,计费按(h+0.5f)*price, 未达1小时但超过30分钟,表示 f>30,计费按(h+1)*price) 其中,停车费用每小时单价price,停车时间minutes和座位数seats 均通过键盘输入。
时间: 2023-06-02 21:07:18 浏览: 175
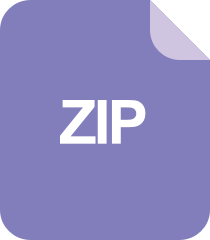
Java模拟停车管理
好的,我明白了。以下是车辆停车收费系统的代码:
```
import java.util.Scanner;
// 车辆类
class Vehicle {
private float price; // 单价
private int minute; // 停车时间(分钟)
// 构造函数
public Vehicle(float price, int minute) {
this.price = price;
this.minute = minute;
}
// get/set方法
public float getPrice() {
return price;
}
public void setPrice(float price) {
this.price = price;
}
public int getMinute() {
return minute;
}
public void setMinute(int minute) {
this.minute = minute;
}
// 计算停车费
public float computeFee() {
float fee = 0;
int h = getMinute() / 60;
int f = getMinute() % 60;
if (h >= 1) {
if (f > 30) {
h += 1;
}
fee = h * getPrice();
}
return fee;
}
}
// 轿车类
class Car extends Vehicle {
public Car(float price, int minute) {
super(price, minute);
}
// 计算停车费
@Override
public float computeFee() {
float fee = 0;
int h = getMinute() / 60;
int f = getMinute() % 60;
if (h >= 1) {
if (f > 30) {
h += 1;
}
fee = h * getPrice();
} else if (h == 0 && f > 30) {
fee = getPrice();
}
return fee;
}
}
// 客车类
class Bus extends Vehicle {
private int seats; // 座位数
public Bus(float price, int minute, int seats) {
super(price, minute);
this.seats = seats;
}
// get/set方法
public int getSeats() {
return seats;
}
public void setSeats(int seats) {
this.seats = seats;
}
// 计算停车费
@Override
public float computeFee() {
float fee = 0;
int h = getMinute() / 60;
int f = getMinute() % 60;
if (h >= 1) {
if (f > 30) {
h += 1;
}
fee = h * getPrice();
} else if (h == 0 && f > 0) {
if (f <= 30) {
fee = getPrice() * 0.5f;
} else if (f > 30 && f <= 60) {
fee = getPrice();
} else if (f > 60) {
fee = getPrice();
}
}
return fee;
}
}
// 主类
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入停车费用每小时单价:");
float price = scanner.nextFloat();
System.out.print("请输入停车时间(分钟):");
int minute = scanner.nextInt();
System.out.print("请输入座位数:");
int seats = scanner.nextInt();
Vehicle vehicle;
if (seats > 0) {
vehicle = new Bus(price, minute, seats);
} else {
vehicle = new Car(price, minute);
}
float fee = vehicle.computeFee();
System.out.println("停车费用为:" + fee + "元");
}
}
```
设计思路:
首先定义一个车辆类Vehicle,包含单价price和停车时间minute两个属性,以及计算停车费用的方法computeFee()。
然后定义两个子类:Car和Bus。Car重写了继承的computeFee()方法,根据不同的停车时间计算停车费用。Bus在Car的基础上新增一个座位数seats属性,并改写了computeFee()方法。根据座位数以及不同的停车时间计算停车费用。
最后在主类中通过键盘输入单价、停车时间以及座位数,判断车辆类型并计算停车费用。
阅读全文
相关推荐
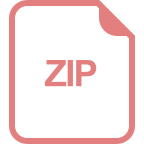
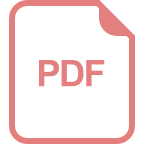















