Java中如何使用Snowflake算法来实现weuyiid
时间: 2024-05-08 17:18:40 浏览: 8
Snowflake算法是一种分布式ID生成算法,可以用来生成唯一ID。在Java中使用Snowflake算法,您需要实现一个Snowflake算法的生成器。下面是一个示例代码:
```
public class SnowflakeIdGenerator {
private static final long twepoch = 1288834974657L;
private static final long workerIdBits = 5L;
private static final long datacenterIdBits = 5L;
private static final long sequenceBits = 12L;
private static final long maxWorkerId = -1L ^ (-1L << workerIdBits);
private static final long maxDatacenterId = -1L ^ (-1L << datacenterIdBits);
private static final long sequenceMask = -1L ^ (-1L << sequenceBits);
private static final long workerIdShift = sequenceBits;
private static final long datacenterIdShift = sequenceBits + workerIdBits;
private static final long timestampLeftShift = sequenceBits + workerIdBits + datacenterIdBits;
private long workerId;
private long datacenterId;
private long sequence = 0L;
private long lastTimestamp = -1L;
public SnowflakeIdGenerator(long workerId, long datacenterId) {
if (workerId > maxWorkerId || workerId < 0) {
throw new IllegalArgumentException("worker Id can't be greater than " + maxWorkerId + " or less than 0");
}
if (datacenterId > maxDatacenterId || datacenterId < 0) {
throw new IllegalArgumentException("datacenter Id can't be greater than " + maxDatacenterId + " or less than 0");
}
this.workerId = workerId;
this.datacenterId = datacenterId;
}
public synchronized long nextId() {
long timestamp = timeGen();
if (timestamp < lastTimestamp) {
throw new RuntimeException("Clock moved backwards. Refusing to generate id");
}
if (lastTimestamp == timestamp) {
sequence = (sequence + 1) & sequenceMask;
if (sequence == 0) {
timestamp = tilNextMillis(lastTimestamp);
}
} else {
sequence = 0L;
}
lastTimestamp = timestamp;
return ((timestamp - twepoch) << timestampLeftShift) |
(datacenterId << datacenterIdShift) |
(workerId << workerIdShift) |
sequence;
}
protected long tilNextMillis(long lastTimestamp) {
long timestamp = timeGen();
while (timestamp <= lastTimestamp) {
timestamp = timeGen();
}
return timestamp;
}
protected long timeGen() {
return System.currentTimeMillis();
}
}
```
在上面的代码中,SnowflakeIdGenerator类实现了一个Snowflake算法的生成器。通过实例化该类,您可以生成唯一的ID。您需要传递workerId和datacenterId参数,这两个参数将用于生成ID。调用nextId()方法即可生成ID。
下面是一个示例代码,演示如何使用Snowflake算法实现weuyiid:
```
SnowflakeIdGenerator idGenerator = new SnowflakeIdGenerator(1, 1);
long weuyiid = idGenerator.nextId();
System.out.println(weuyiid);
```
在上面的代码中,我们实例化了SnowflakeIdGenerator类,并传递了workerId和datacenterId参数。使用nextId()方法即可生成唯一的ID,并将其赋值给weuyiid变量。最后,我们将weuyiid值打印出来。
相关推荐
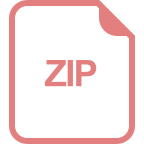
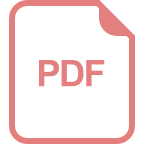
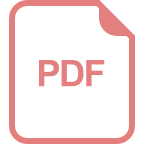














