眼科疾病分类python代码
时间: 2023-09-11 20:07:23 浏览: 151
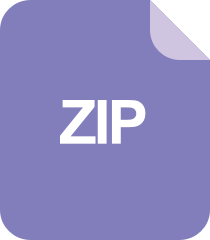
层次分析matlab代码-eyepy:用于根据人类眼动模式对人类进行分类的Python工具箱
以下是一个简单的眼科疾病分类的Python代码示例,使用了Keras深度学习框架和眼科疾病图像数据集:
```python
import os
import numpy as np
import tensorflow as tf
from tensorflow import keras
from tensorflow.keras import layers
# 设置GPU内存增长
physical_devices = tf.config.list_physical_devices('GPU')
tf.config.experimental.set_memory_growth(physical_devices[0], True)
# 数据路径和参数
data_path = 'path/to/dataset/'
img_height = 256
img_width = 256
batch_size = 32
# 数据预处理
train_ds = keras.preprocessing.image_dataset_from_directory(
data_path,
validation_split=0.2,
subset="training",
seed=123,
image_size=(img_height, img_width),
batch_size=batch_size)
val_ds = keras.preprocessing.image_dataset_from_directory(
data_path,
validation_split=0.2,
subset="validation",
seed=123,
image_size=(img_height, img_width),
batch_size=batch_size)
class_names = train_ds.class_names
num_classes = len(class_names)
# 数据增强
data_augmentation = keras.Sequential(
[
layers.experimental.preprocessing.RandomFlip("horizontal",
input_shape=(img_height,
img_width,
3)),
layers.experimental.preprocessing.RandomRotation(0.1),
layers.experimental.preprocessing.RandomZoom(0.1),
]
)
# 构建模型
model = keras.Sequential([
data_augmentation,
layers.experimental.preprocessing.Rescaling(1./255),
layers.Conv2D(32, 3, padding='same', activation='relu'),
layers.MaxPooling2D(),
layers.Conv2D(64, 3, padding='same', activation='relu'),
layers.MaxPooling2D(),
layers.Conv2D(128, 3, padding='same', activation='relu'),
layers.MaxPooling2D(),
layers.Flatten(),
layers.Dense(128, activation='relu'),
layers.Dense(num_classes)
])
# 编译模型
model.compile(optimizer='adam',
loss=tf.keras.losses.SparseCategoricalCrossentropy(from_logits=True),
metrics=['accuracy'])
# 训练模型
epochs = 10
model.fit(
train_ds,
validation_data=val_ds,
epochs=epochs
)
# 评估模型
test_ds = keras.preprocessing.image_dataset_from_directory(
data_path,
seed=123,
image_size=(img_height, img_width),
batch_size=batch_size)
loss, accuracy = model.evaluate(test_ds)
print("Accuracy", accuracy)
```
这个示例代码使用了卷积神经网络(CNN)来训练眼科疾病分类模型。数据集使用了眼科图像数据集,预处理包括数据增强和归一化。在训练过程中,使用了验证集进行模型性能评估,并在测试集上进行最终评估。
阅读全文
相关推荐
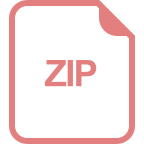
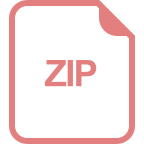
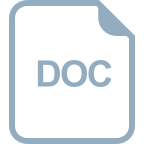
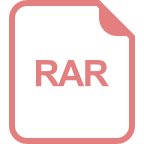
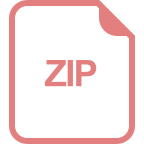
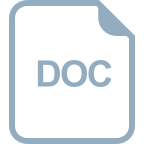
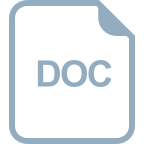
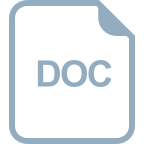
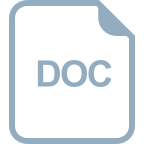
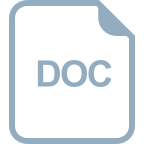
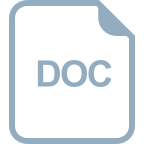
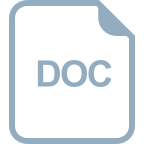
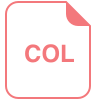
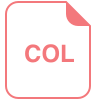
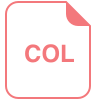
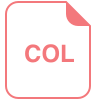
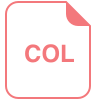
