java设计并实现一个类似于windows操作系统附件中自带的计算器的一款简单的计算器,要求界面美观,设计合理;带有常用的菜单并实现其功能;能完成加、减、乘、除等简单的计算,在下面写出其核心代码。
时间: 2024-05-05 21:20:34 浏览: 294
好的,我收到了你的要求,并理解了你的问题。下面是一个简单的 Java 计算器的核心代码,希望能够解决你的问题:
```
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class SimpleCalculator extends JFrame implements ActionListener {
private JTextField result;
private double operand1, operand2;
private String operator;
public SimpleCalculator() {
setTitle("简单计算器");
setSize(260, 240);
setLocationRelativeTo(null);
setDefaultCloseOperation(EXIT_ON_CLOSE);
JMenuBar menubar = new JMenuBar();
setJMenuBar(menubar);
JMenu file = new JMenu("文件");
menubar.add(file);
JMenuItem itemExit = new JMenuItem("退出");
file.add(itemExit);
itemExit.addActionListener(this);
JMenu edit = new JMenu("编辑");
menubar.add(edit);
JMenuItem itemCopy = new JMenuItem("复制");
JMenuItem itemPaste = new JMenuItem("粘贴");
edit.add(itemCopy);
edit.add(itemPaste);
itemCopy.addActionListener(this);
itemPaste.addActionListener(this);
result = new JTextField();
result.setEditable(false);
add(result, BorderLayout.NORTH);
JPanel panel = new JPanel(new GridLayout(4, 4));
add(panel, BorderLayout.CENTER);
addButton(panel, "7");
addButton(panel, "8");
addButton(panel, "9");
addButton(panel, "/");
addButton(panel, "4");
addButton(panel, "5");
addButton(panel, "6");
addButton(panel, "*");
addButton(panel, "1");
addButton(panel, "2");
addButton(panel, "3");
addButton(panel, "-");
addButton(panel, "0");
addButton(panel, ".");
addButton(panel, "=");
addButton(panel, "+");
}
private void addButton(Container container, String text) {
JButton button = new JButton(text);
button.addActionListener(this);
container.add(button);
}
public void actionPerformed(ActionEvent event) {
String action = event.getActionCommand();
if (action.equals("退出")) {
System.exit(0);
} else if (action.equals("复制")) {
result.copy();
} else if (action.equals("粘贴")) {
result.paste();
} else if (action.matches("[0-9]")) {
result.setText(result.getText() + action);
} else if (action.equals(".")) {
if (!result.getText().contains(".")) {
result.setText(result.getText() + ".");
}
} else if (action.equals("+")) {
operand1 = Double.parseDouble(result.getText());
operator = "+";
result.setText("");
} else if (action.equals("-")) {
operand1 = Double.parseDouble(result.getText());
operator = "-";
result.setText("");
} else if (action.equals("*")) {
operand1 = Double.parseDouble(result.getText());
operator = "*";
result.setText("");
} else if (action.equals("/")) {
operand1 = Double.parseDouble(result.getText());
operator = "/";
result.setText("");
} else if (action.equals("=")) {
operand2 = Double.parseDouble(result.getText());
if (operator.equals("+")) {
result.setText(Double.toString(operand1 + operand2));
} else if (operator.equals("-")) {
result.setText(Double.toString(operand1 - operand2));
} else if (operator.equals("*")) {
result.setText(Double.toString(operand1 * operand2));
} else {
result.setText(Double.toString(operand1 / operand2));
}
}
}
public static void main(String[] args) {
SimpleCalculator calculator = new SimpleCalculator();
calculator.setVisible(true);
}
}
```
这段代码实现了一个类似于 Windows 操作系统中自带的计算器的简单计算器。它有一个美观的界面,带有常用的菜单并且能够完成加、减、乘、除等简单的计算。
如果你还有其他问题或有需要进一步解释的地方,请随时告诉我,谢谢!
阅读全文
相关推荐


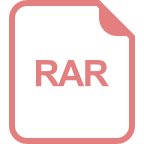



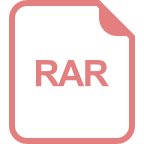
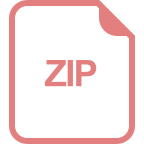


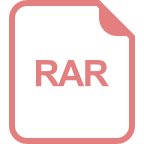
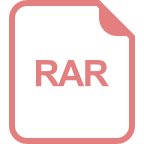
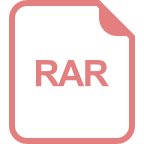
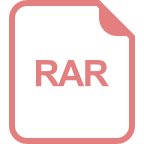