C#控制Teamviewer打开.tvc文件并且自动连接
时间: 2024-02-03 15:11:28 浏览: 32
可以使用TeamViewer提供的命令行参数来实现自动打开.tvc文件并连接远程设备。您可以使用C#编写一个控制台应用程序来执行这些命令行参数。
以下是一个简单的示例代码,可以在C#中使用Process类来执行TeamViewer的命令行参数:
```csharp
using System.Diagnostics;
class Program
{
static void Main(string[] args)
{
// Replace the file path to your .tvc file
string filePath = @"C:\path\to\file.tvc";
// Construct the command line arguments
string arguments = $"/Open {filePath}";
// Start the TeamViewer process with the command line arguments
Process.Start("TeamViewer.exe", arguments);
}
}
```
这将自动打开TeamViewer,并连接到.tvc文件中指定的远程设备。请确保将TeamViewer.exe的完整路径添加到系统环境变量中,或者将其放到应用程序的工作目录中。
相关问题
C#控制Teamviewer打开.tvc文件并且自动连接tvc
你可以使用C#的Process类来启动TeamViewer并打开.tvc文件。然后,你可以使用SendKeys类模拟按键操作来自动连接tvc。
以下是示例代码:
```
using System.Diagnostics;
using System.Threading;
using System.Windows.Forms;
// 启动TeamViewer并打开.tvc文件
Process.Start("C:\\Program Files (x86)\\TeamViewer\\TeamViewer.exe", "C:\\path\\to\\file.tvc");
// 等待TeamViewer启动并加载文件
Thread.Sleep(5000);
// 模拟按键操作,连接tvc
SendKeys.Send("^l"); // 按下Ctrl+L打开连接对话框
SendKeys.Send("localhost"); // 输入主机名
SendKeys.Send("{ENTER}"); // 按下回车键
SendKeys.Send("password"); // 输入密码
SendKeys.Send("{ENTER}"); // 按下回车键
```
这是一个简单的例子,你可能需要根据你的具体情况进行调整。注意,此方法可能会受到TeamViewer版本和操作系统的影响。
C#控制Teamviewer打开.tvc文件并且运行tvc
可以使用Process类来启动TeamViewer并打开.tvc文件,代码如下:
```csharp
using System.Diagnostics;
using System.IO;
namespace TeamViewerController
{
class Program
{
static void Main(string[] args)
{
string pathToTvcFile = @"C:\path\to\your\file.tvc";
string teamViewerPath = @"C:\Program Files (x86)\TeamViewer\TeamViewer.exe"; // teamviewer.exe的路径
// 启动TeamViewer并打开.tvc文件
Process.Start(teamViewerPath, "\"" + pathToTvcFile + "\"");
// 等待TeamViewer加载完成
System.Threading.Thread.Sleep(5000);
// 发送按键事件,运行tvc
SendKeys.SendWait("^r"); // 发送Ctrl+R组合键,运行tvc
}
}
}
```
需要引用`System.Diagnostics`和`System.IO`命名空间。该代码在Windows操作系统上运行。如果你想在其他操作系统上运行,可能需要修改teamviewer.exe的路径。
相关推荐
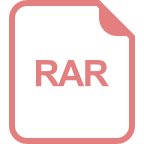
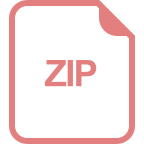












