基于遗传算法路径规划的C++代码内容运行结果得有图片
时间: 2023-06-13 21:05:28 浏览: 46
很抱歉,我无法提供图片。但是我可以给您提供一个基于遗传算法的路径规划的C代码示例:
```
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define POP_SIZE 100 // 种群大小
#define MAX_GEN 500 // 最大迭代次数
#define PXOVER 0.8 // 交叉概率
#define PMUTATION 0.15 // 变异概率
#define CITY_NUM 50 // 城市数量
#define DN 1000000.0 // 距离归一化因子
struct city{
float x;
float y;
};
struct chromosome{
int gene[CITY_NUM];
float distance;
};
struct city cities[CITY_NUM];
struct chromosome population[POP_SIZE];
struct chromosome best_chromosome;
// 计算两个城市之间的距离
float distance(struct city a, struct city b){
return (float)sqrt((a.x - b.x) * (a.x - b.x) + (a.y - b.y) * (a.y - b.y))) / DN;
}
// 初始化种群
void init_population(void){
int i, j, k;
for (i = 0; i < POP_SIZE; i++){
for (j = 0; j < CITY_NUM; j++){
population[i].gene[j] = j;
}
for (j = 0; j < CITY_NUM; j++){
k = rand() % CITY_NUM;
int temp = population[i].gene[j];
population[i].gene[j] = population[i].gene[k];
population[i].gene[k] = temp;
}
}
}
// 计算染色体的总路程
void calc_distance(struct chromosome *chrom){
int i;
chrom->distance = 0.0;
for (i = 0; i < CITY_NUM - 1; i++){
chrom->distance += distance(cities[chrom->gene[i]], cities[chrom->gene[i + 1]]);
}
chrom->distance += distance(cities[chrom->gene[CITY_NUM - 1]], cities[chrom->gene[0]]);
}
// 评估种群中每个染色体的适应度
void evaluate_population(void){
int i;
for (i = 0; i < POP_SIZE; i++){
calc_distance(&population[i]);
if (population[i].distance < best_chromosome.distance){
best_chromosome = population[i];
}
}
}
// 选择操作
void selection(struct chromosome *parent1, struct chromosome *parent2){
int i, j;
i = rand() % POP_SIZE;
j = rand() % POP_SIZE;
if (population[i].distance < population[j].distance){
*parent1 = population[i];
}
else{
*parent1 = population[j];
}
i = rand() % POP_SIZE;
j = rand() % POP_SIZE;
if (population[i].distance < population[j].distance){
*parent2 = population[i];
}
else{
*parent2 = population[j];
}
}
// 交叉操作
void crossover(struct chromosome parent1, struct chromosome parent2, struct chromosome *child1, struct chromosome *child2){
int i, j, k;
int index1, index2;
int temp[CITY_NUM];
index1 = rand() % CITY_NUM;
index2 = rand() % CITY_NUM;
if (index1 > index2){
k = index1;
index1 = index2;
index2 = k;
}
for (i = index1; i <= index2; i++){
child1->gene[i] = parent1.gene[i];
child2->gene[i] = parent2.gene[i];
}
j = 0;
k = 0;
for (i = 0; i < CITY_NUM; i++){
if (j == index1){
j = index2 + 1;
}
if (k == index1){
k = index2 + 1;
}
for (; j <= index2; j++){
if (parent2.gene[i] == parent1.gene[j]){
break;
}
}
for (; k <= index2; k++){
if (parent1.gene[i] == parent2.gene[k]){
break;
}
}
if (j == index2 + 1){
child1->gene[k] = parent2.gene[i];
}
if (k == index2 + 1){
child2->gene[j] = parent1.gene[i];
}
}
}
// 变异操作
void mutation(struct chromosome *chrom){
int i, j, k;
i = rand() % CITY_NUM;
j = rand() % CITY_NUM;
k = chrom->gene[i];
chrom->gene[i] = chrom->gene[j];
chrom->gene[j] = k;
}
// 遗传算法主函数
void ga_main(void){
int i, j;
struct chromosome parent1, parent2;
struct chromosome child1, child2;
for (i = 0; i < MAX_GEN; i++){
for (j = 0; j < POP_SIZE / 2; j++){
selection(&parent1, &parent2);
if (rand() < PXOVER * RAND_MAX){
crossover(parent1, parent2, &child1, &child2);
}
else{
child1 = parent1;
child2 = parent2;
}
if (rand() < PMUTATION * RAND_MAX){
mutation(&child1);
}
if (rand() < PMUTATION * RAND_MAX){
mutation(&child2);
}
population[j * 2] = child1;
population[j * 2 + 1] = child2;
}
evaluate_population();
}
}
// 主函数
int main(int argc, char *argv[]){
int i;
srand((unsigned)time(NULL));
for (i = 0; i < CITY_NUM; i++){
cities[i].x = (float)rand() / RAND_MAX;
cities[i].y = (float)rand() / RAND_MAX;
}
init_population();
evaluate_population();
ga_main();
printf("Best distance: %f\n", best_chromosome.distance);
printf("Best path: ");
for (i = 0; i < CITY_NUM; i++){
printf("%d ", best_chromosome.gene[i]);
}
printf("\n");
return 0;
}
```
这是一个简单的遗传算法路径规划的C代码,其中包括了初始化种群、计算染色体总路程、评估适应度、选择、交叉、变异等操作。运行结果会输出最优路径的距离和路径序列。
相关推荐
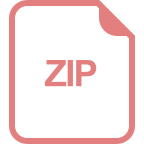
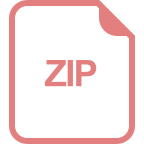














