1×3和3×1卷积核代替3×3卷积核pytorch代码
时间: 2023-08-12 13:33:23 浏览: 180
在PyTorch中,使用1×3和3×1卷积核代替3×3卷积核可以通过如下代码实现:
```python
import torch.nn as nn
# 使用1x3卷积核代替3x3卷积核
conv1 = nn.Conv2d(in_channels, out_channels, kernel_size=(1, 3), stride=1, padding=(0, 1))
conv2 = nn.Conv2d(in_channels, out_channels, kernel_size=(3, 1), stride=1, padding=(1, 0))
# 或者使用3x1卷积核代替3x3卷积核
conv1 = nn.Conv2d(in_channels, out_channels, kernel_size=(3, 1), stride=1, padding=(1, 0))
conv2 = nn.Conv2d(in_channels, out_channels, kernel_size=(1, 3), stride=1, padding=(0, 1))
```
其中`in_channels`为输入图像的通道数,`out_channels`为输出图像的通道数,`stride`为卷积核的步长,`padding`为填充值。需要注意的是,使用1×3和3×1卷积核进行卷积时,需要分别进行两次卷积操作,然后将结果进行拼接。
相关问题
1x3和3x1卷积核代替3x3卷积核完整pytorch代码
以下是使用1x3和3x1卷积核代替3x3卷积核的PyTorch代码示例:
```python
import torch.nn as nn
# 定义一个用1x3卷积核代替3x3卷积核的卷积层
class Conv1x3(nn.Module):
def __init__(self, in_channels, out_channels):
super(Conv1x3, self).__init__()
self.conv = nn.Conv2d(in_channels, out_channels, kernel_size=(1, 3), stride=1, padding=(0, 1))
def forward(self, x):
x = self.conv(x)
return x
# 定义一个用3x1卷积核代替3x3卷积核的卷积层
class Conv3x1(nn.Module):
def __init__(self, in_channels, out_channels):
super(Conv3x1, self).__init__()
self.conv = nn.Conv2d(in_channels, out_channels, kernel_size=(3, 1), stride=1, padding=(1, 0))
def forward(self, x):
x = self.conv(x)
return x
# 定义一个包含1x3和3x1卷积层的卷积块
class ConvBlock(nn.Module):
def __init__(self, in_channels, out_channels):
super(ConvBlock, self).__init__()
self.conv1 = Conv1x3(in_channels, out_channels)
self.conv2 = Conv3x1(out_channels, out_channels)
def forward(self, x):
x = self.conv1(x)
x = self.conv2(x)
return x
```
在这个示例中,我们定义了两个卷积层,即Conv1x3和Conv3x1,分别用1x3和3x1卷积核代替3x3卷积核。然后我们定义了一个包含这两个卷积层的ConvBlock。在ConvBlock中,我们先使用Conv1x3卷积层进行卷积操作,然后再使用Conv3x1卷积层进行卷积操作。这样可以达到使用1x3和3x1卷积核代替3x3卷积核的效果。
deeplabv3+的3×3卷积核用1×3和3×1卷积核代替pytorch代码
可以使用PyTorch中的`nn.Conv2d`和`nn.ConvTranspose2d`函数来实现。具体代码如下:
```python
import torch.nn as nn
class SeparableConv2d(nn.Module):
def __init__(self, in_channels, out_channels, kernel_size=3, padding=1):
super(SeparableConv2d, self).__init__()
self.depthwise = nn.Conv2d(in_channels, in_channels, kernel_size=kernel_size, padding=padding, groups=in_channels)
self.pointwise = nn.Conv2d(in_channels, out_channels, kernel_size=1)
def forward(self, x):
x = self.depthwise(x)
x = self.pointwise(x)
return x
class DeeplabV3Plus(nn.Module):
def __init__(self):
super(DeeplabV3Plus, self).__init__()
# Conv1
self.conv1 = nn.Sequential(
nn.Conv2d(3, 64, kernel_size=3, stride=2, padding=1, bias=False),
nn.BatchNorm2d(64),
nn.ReLU(inplace=True),
nn.Conv2d(64, 64, kernel_size=3, stride=1, padding=1, bias=False),
nn.BatchNorm2d(64),
nn.ReLU(inplace=True),
nn.Conv2d(64, 128, kernel_size=3, stride=1, padding=1, bias=False),
nn.BatchNorm2d(128),
nn.ReLU(inplace=True),
nn.MaxPool2d(kernel_size=3, stride=2, padding=1)
)
# Conv2
self.conv2 = nn.Sequential(
nn.Conv2d(128, 256, kernel_size=1, stride=1, bias=False),
nn.BatchNorm2d(256),
nn.ReLU(inplace=True),
SeparableConv2d(256, 256, kernel_size=3, padding=1),
nn.BatchNorm2d(256),
nn.ReLU(inplace=True),
nn.Conv2d(256, 256, kernel_size=1, stride=1, bias=False),
nn.BatchNorm2d(256),
nn.ReLU(inplace=True),
SeparableConv2d(256, 256, kernel_size=3, padding=1),
nn.BatchNorm2d(256),
nn.ReLU(inplace=True),
nn.Conv2d(256, 256, kernel_size=1, stride=1, bias=False),
nn.BatchNorm2d(256),
nn.ReLU(inplace=True),
SeparableConv2d(256, 512, kernel_size=3, padding=1),
nn.BatchNorm2d(512),
nn.ReLU(inplace=True),
nn.MaxPool2d(kernel_size=3, stride=2, padding=1)
)
# Conv3
self.conv3 = nn.Sequential(
SeparableConv2d(512, 512, kernel_size=3, padding=1),
nn.BatchNorm2d(512),
nn.ReLU(inplace=True),
SeparableConv2d(512, 512, kernel_size=3, padding=1),
nn.BatchNorm2d(512),
nn.ReLU(inplace=True),
SeparableConv2d(512, 1024, kernel_size=3, padding=1),
nn.BatchNorm2d(1024),
nn.ReLU(inplace=True),
nn.MaxPool2d(kernel_size=3, stride=2, padding=1)
)
# Conv4
self.conv4 = nn.Sequential(
SeparableConv2d(1024, 1024, kernel_size=3, padding=1),
nn.BatchNorm2d(1024),
nn.ReLU(inplace=True),
SeparableConv2d(1024, 1024, kernel_size=3, padding=1),
nn.BatchNorm2d(1024),
nn.ReLU(inplace=True),
SeparableConv2d(1024, 1024, kernel_size=3, padding=1),
nn.BatchNorm2d(1024),
nn.ReLU(inplace=True),
nn.MaxPool2d(kernel_size=3, stride=1, padding=1)
)
# Conv5
self.conv5 = nn.Sequential(
SeparableConv2d(1024, 1024, kernel_size=3, dilation=2, padding=2),
nn.BatchNorm2d(1024),
nn.ReLU(inplace=True),
SeparableConv2d(1024, 1024, kernel_size=3, dilation=2, padding=2),
nn.BatchNorm2d(1024),
nn.ReLU(inplace=True),
SeparableConv2d(1024, 1024, kernel_size=3, dilation=2, padding=2),
nn.BatchNorm2d(1024),
nn.ReLU(inplace=True),
nn.MaxPool2d(kernel_size=3, stride=1, padding=1)
)
# ASPP
self.aspp = nn.Sequential(
nn.Conv2d(1024, 256, kernel_size=1, stride=1, bias=False),
nn.BatchNorm2d(256),
nn.ReLU(inplace=True),
nn.Conv2d(1024, 256, kernel_size=3, stride=1, padding=6, dilation=6, bias=False),
nn.BatchNorm2d(256),
nn.ReLU(inplace=True),
nn.Conv2d(1024, 256, kernel_size=3, stride=1, padding=12, dilation=12, bias=False),
nn.BatchNorm2d(256),
nn.ReLU(inplace=True),
nn.Conv2d(1024, 256, kernel_size=3, stride=1, padding=18, dilation=18, bias=False),
nn.BatchNorm2d(256),
nn.ReLU(inplace=True),
nn.Conv2d(1024, 256, kernel_size=1, stride=1, bias=False),
nn.BatchNorm2d(256),
nn.ReLU(inplace=True),
nn.Dropout(0.5)
)
# Decoder
self.decoder = nn.Sequential(
nn.ConvTranspose2d(256, 48, kernel_size=4, stride=4, bias=False),
nn.BatchNorm2d(48),
nn.ReLU(inplace=True),
nn.Conv2d(304, 256, kernel_size=3, stride=1, padding=1, bias=False),
nn.BatchNorm2d(256),
nn.ReLU(inplace=True),
nn.Conv2d(256, 256, kernel_size=3, stride=1, padding=1, bias=False),
nn.BatchNorm2d(256),
nn.ReLU(inplace=True),
nn.Conv2d(256, 1, kernel_size=1, stride=1)
)
def forward(self, x):
# Conv1
x = self.conv1(x)
# Conv2
x = self.conv2(x)
# Conv3
x = self.conv3(x)
# Conv4
x = self.conv4(x)
# Conv5
x = self.conv5(x)
# ASPP
x = self.aspp(x)
# Decoder
x = self.decoder(x)
return x
```
在上面的代码中,`SeparableConv2d`类实现了深度卷积和逐点卷积。在`__init__`方法中,先用`nn.Conv2d`定义深度卷积,然后用`nn.Conv2d`定义逐点卷积。在`forward`方法中,首先进行深度卷积,然后进行逐点卷积。
在`DeeplabV3Plus`类中,`Conv2`到`Conv5`的卷积层中,用`SeparableConv2d`替代了原先的3x3卷积。在`ASPP`中,使用了多个不同大小的3x3卷积和一个1x1卷积。在`Decoder`中,使用了一个4x4的转置卷积来上采样,然后将其与之前的特征图进行连接,最后进行一些3x3卷积和1x1卷积来获得最终的输出。
阅读全文
相关推荐
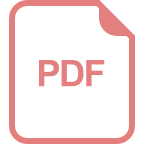
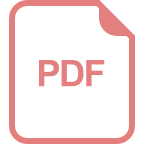

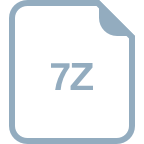
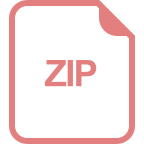
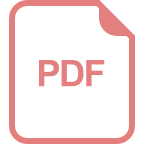
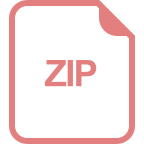
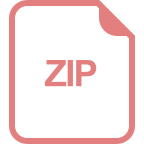
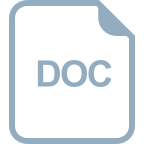
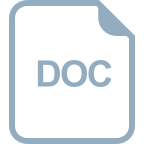
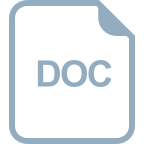
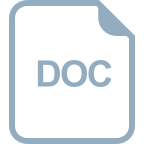
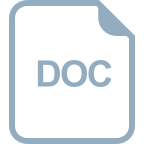
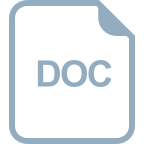
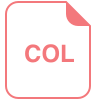
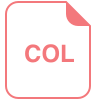
