前端如何用axios连接后端接口,并载入前端的数组中
时间: 2023-12-10 14:39:43 浏览: 75
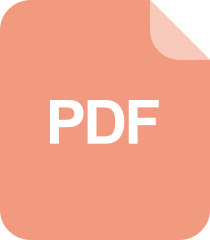
前端与后端连接,Ajax,axios,jquery等练习
首先,你需要在前端项目中安装 `axios` 库,可以使用 `npm` 或 `yarn` 命令进行安装。
然后,在前端代码中使用 `axios` 发送请求到后端接口,并将返回的数据存储在前端数组中,可以按照以下步骤进行操作:
1. 导入 `axios` 库:
```javascript
import axios from 'axios';
```
2. 发送请求到后端接口:
```javascript
axios.get('/api/data') // 发送 GET 请求到 /api/data 接口
.then(response => {
// 处理响应数据并存储到前端数组中
const data = response.data;
const frontendArray = []; // 前端数组
data.forEach(item => {
frontendArray.push(item);
});
})
.catch(error => {
console.error(error);
});
```
在以上代码中,我们使用 `axios.get()` 方法发送 GET 请求到 `/api/data` 接口,并在请求成功后将返回的数据存储到 `frontendArray` 前端数组中。
3. 在前端界面中展示前端数组中的数据。
```javascript
// 在 Vue.js 中的模板中展示前端数组中的数据
<template>
<div>
<ul>
<li v-for="item in frontendArray" :key="item.id">{{ item.name }}</li>
</ul>
</div>
</template>
<script>
import axios from 'axios';
export default {
data() {
return {
frontendArray: []
}
},
mounted() {
axios.get('/api/data')
.then(response => {
const data = response.data;
data.forEach(item => {
this.frontendArray.push(item);
});
})
.catch(error => {
console.error(error);
});
}
}
</script>
```
在以上代码中,我们在 Vue.js 的组件中展示了前端数组中的数据。使用 `v-for` 指令遍历数组中的数据,并将数据展示在页面上。
当然,以上代码只是一个简单的示例,实际开发中需要根据具体的需求进行调整。
阅读全文
相关推荐















