用nodejs实现从题库中提取选择题与填空题,然后组合成一张a3大小的试卷并打印出来
时间: 2023-06-19 12:07:48 浏览: 192
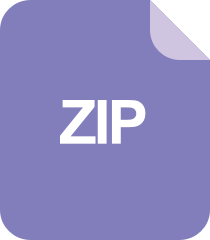
ServiceNowScriptDocumenter:使用NodeJS从ServiceNow中提取脚本,将其写入javascript文件,并基于这些文件创建文档
这个任务比较复杂,需要涉及到多个方面的知识,包括Node.js、Web Scraping、PDF生成、打印等。下面我将简单介绍实现的步骤和需要用到的工具和库。
步骤:
1. 确定选择题和填空题的来源,可以是网站、数据库或文件等。
2. 使用Node.js编写脚本,利用Web Scraping技术从来源中提取出选择题和填空题的内容,并将其保存到一个数据结构中。
3. 设计试卷的格式,包括标题、题号、题目、选项、答案等信息,并使用HTML和CSS编写模板。
4. 使用JavaScript将从数据结构中提取出来的题目插入到模板中,并生成HTML页面。
5. 使用第三方库(例如Puppeteer)将HTML页面转换为PDF文件,并设置A3大小。
6. 将生成的PDF文件打印出来。
需要用到的工具和库:
1. Node.js:一个基于Chrome V8引擎的JavaScript运行环境。
2. Cheerio:一个类似于jQuery的库,用于在Node.js中解析HTML文档并提取数据。
3. Request或Axios:HTTP客户端库,用于从网站中获取数据。
4. Puppeteer:一个基于Chrome DevTools协议的无头浏览器,用于生成PDF文件。
5. PDFKit:一个用于生成PDF文件的Node.js库。
6. Printer:一个用于打印PDF文件的Node.js库。
以下是一个简单的代码示例:
```javascript
const cheerio = require('cheerio');
const request = require('request');
const puppeteer = require('puppeteer');
const PDFDocument = require('pdfkit');
const printer = require('printer');
// 从网站中获取选择题和填空题
request('http://example.com/questions', (err, res, body) => {
if (err) throw err;
const $ = cheerio.load(body);
const questions = [];
// 提取选择题
$('.question-choice').each((i, el) => {
const question = {
type: 'choice',
number: i + 1,
content: $(el).find('.question-content').text(),
options: [],
answer: $(el).find('.question-answer').text(),
};
$(el).find('.question-option').each((j, option) => {
question.options.push($(option).text());
});
questions.push(question);
});
// 提取填空题
$('.question-fill').each((i, el) => {
const question = {
type: 'fill',
number: i + 1,
content: $(el).find('.question-content').text(),
answer: $(el).find('.question-answer').text(),
};
questions.push(question);
});
// 生成HTML页面
const html = `
<html>
<head>
<style>
/* 样式 */
</style>
</head>
<body>
<h1>测试试卷</h1>
${questions.map(question => `
<div class="question">
<div class="question-number">${question.number}</div>
<div class="question-content">${question.content}</div>
${question.type === 'choice' ? `
<ul class="question-options">
${question.options.map(option => `
<li>${option}</li>
`).join('')}
</ul>
<div class="question-answer">答案:${question.answer}</div>
` : `
<div class="question-answer">答案:_______________________</div>
`}
</div>
`).join('')}
</body>
</html>
`;
// 生成PDF文件
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.setContent(html);
const pdf = await page.pdf({
format: 'A3',
printBackground: true,
});
await browser.close();
// 打印PDF文件
const doc = new PDFDocument();
doc.pipe(printer.createWriteStream());
doc.image(pdf);
doc.end();
})();
});
```
需要注意的是,以上代码仅为示例,实际应用中可能存在性能问题、网站结构变化等问题需要处理。同时,还需要考虑试卷的排版、题目的难度、题型的多样性等因素,以生成一份质量较高的试卷。
阅读全文
相关推荐
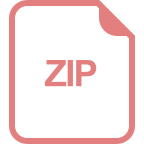
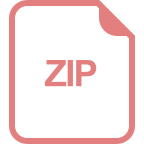













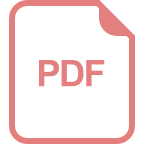
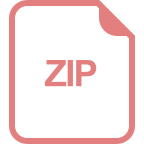
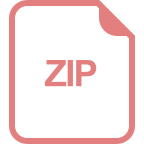