springboot将数据库数据导入word
时间: 2023-12-16 21:06:26 浏览: 33
要实现将数据库数据导入 Word,可以使用 Apache POI 库,它是 Java 操作 Office 文档的一个 API。以下是一个简单的示例代码:
1. 首先,需要在 pom.xml 文件中添加 Apache POI 的依赖:
```xml
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>4.1.2</version>
</dependency>
```
2. 然后,创建一个 WordUtils 类,用于导出 Word 文档:
```java
public class WordUtils {
public static void exportDataToWord(List<User> userList, String filePath) throws IOException {
// 创建 Word 文档
XWPFDocument document = new XWPFDocument();
// 创建段落
XWPFParagraph paragraph = document.createParagraph();
// 创建表格
XWPFTable table = document.createTable();
// 设置表格列数
int columnCount = 2;
table.getCTTbl().addNewTblGrid().addNewGridCol().setW(BigInteger.valueOf(5000));
table.getCTTbl().getTblGrid().addNewGridCol().setW(BigInteger.valueOf(5000));
// 添加表头行
XWPFTableRow headerRow = table.getRow(0);
headerRow.getCell(0).setText("ID");
headerRow.getCell(1).setText("Name");
// 添加数据行
for (User user : userList) {
XWPFTableRow dataRow = table.createRow();
dataRow.getCell(0).setText(String.valueOf(user.getId()));
dataRow.getCell(1).setText(user.getName());
}
// 输出 Word 文档
FileOutputStream outputStream = new FileOutputStream(filePath);
document.write(outputStream);
outputStream.close();
}
}
```
3. 最后,在 Controller 中调用 WordUtils 类的 exportDataToWord() 方法即可:
```java
@RestController
public class UserController {
@Autowired
private UserService userService;
@GetMapping("/export")
public void exportToWord(HttpServletResponse response) throws IOException {
List<User> userList = userService.getAllUsers();
String fileName = "user_list.docx";
String filePath = "D:\\" + fileName;
WordUtils.exportDataToWord(userList, filePath);
response.setContentType("application/vnd.openxmlformats-officedocument.wordprocessingml.document");
response.setHeader("Content-Disposition", "attachment; filename=" + fileName);
File file = new File(filePath);
InputStream inputStream = new FileInputStream(file);
IOUtils.copy(inputStream, response.getOutputStream());
response.flushBuffer();
}
}
```
注意,上述代码中的 User 类是数据库表对应的实体类,getAllUsers() 方法是用于获取所有用户数据的方法,可以根据实际情况进行调整。同时,为了让用户能够下载生成的 Word 文档,需要在响应头中设置 Content-Disposition 和 Content-Type。
相关推荐
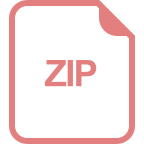
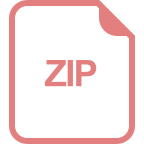














