用jsp在网页中插入图片
时间: 2023-06-01 17:02:41 浏览: 321
在jsp中插入图片可以使用html标签<img>,如下示例:
```html
<img src="图片路径" alt="图片描述">
```
其中,src属性指定图片的路径,alt属性是图片的描述,可以在图片无法显示时显示该描述。在jsp中,可以使用相对路径或绝对路径指定图片路径。
示例代码:
```jsp
<html>
<head>
<title>插入图片</title>
</head>
<body>
<h1>图片展示</h1>
<img src="images/pic.jpg" alt="美丽的风景">
</body>
</html>
```
在上述示例中,图片路径为相对路径,图片文件名为pic.jpg,存放在images文件夹中。如果图片路径为绝对路径,则直接指定完整的URL即可。
注:需要确保图片文件存在,路径正确。
相关问题
mysql数据库插入图片后使用java语言将blob数据转换为图片并在网页上显示出来
在 Java 中,可以使用 JDBC API 将从 MySQL 数据库中检索到的 BLOB 数据转换为图片。然后,可以使用 Servlet 或 JSP 将图片嵌入到 HTML 中,以在 Web 页面上显示图片。下面是具体的步骤:
1. 使用 JDBC API 连接到 MySQL 数据库,并执行 SELECT 语句以检索包含图片的 BLOB 列。
```java
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "username", "password");
PreparedStatement stmt = conn.prepareStatement("SELECT data FROM images WHERE id = ?");
stmt.setInt(1, 1);
ResultSet rs = stmt.executeQuery();
```
其中,1 是图片所在行的 ID。
2. 从 ResultSet 对象中提取 BLOB 列中的二进制数据。
```java
if (rs.next()) {
Blob blob = rs.getBlob("data");
InputStream inputStream = blob.getBinaryStream();
}
```
3. 将二进制数据转换为图片,并将其写入 Servlet 或 JSP 的输出流中。
```java
response.setContentType("image/jpeg"); // 设置响应内容类型为图片类型
OutputStream outputStream = response.getOutputStream();
byte[] buffer = new byte[4096]; // 缓冲区大小
int bytesRead = -1;
while ((bytesRead = inputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, bytesRead); // 将图片数据写入输出流
}
inputStream.close();
outputStream.close();
```
其中,response.setContentType() 方法用于设置响应内容类型为图片类型,getOutputStream() 方法用于获取输出流,write() 方法用于将图片数据写入输出流。
4. 在 HTML 中嵌入图片。
```html
<img src="/imageServlet?id=1" alt="example">
```
其中,src 属性指向 Servlet 或 JSP 的 URL,id 参数指定图片所在行的 ID。
完整的代码示例如下:
ImageServlet.java:
```java
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.sql.Blob;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class ImageServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
try {
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "username", "password");
PreparedStatement stmt = conn.prepareStatement("SELECT data FROM images WHERE id = ?");
stmt.setInt(1, Integer.parseInt(request.getParameter("id")));
ResultSet rs = stmt.executeQuery();
if (rs.next()) {
Blob blob = rs.getBlob("data");
InputStream inputStream = blob.getBinaryStream();
response.setContentType("image/jpeg");
OutputStream outputStream = response.getOutputStream();
byte[] buffer = new byte[4096];
int bytesRead = -1;
while ((bytesRead = inputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, bytesRead);
}
inputStream.close();
outputStream.close();
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
index.jsp:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Image Example</title>
</head>
<body>
<img src="/imageServlet?id=1" alt="example">
</body>
</html>
```
其中,/imageServlet?id=1 是 Servlet 的 URL,id 参数指定图片所在行的 ID。将 ImageServlet.java 和 index.jsp 部署到 Web 服务器中,访问 index.jsp 即可在 Web 页面上显示图片。
为网页制作一个精美的logo,公司地址的信息和时间,对应的页面为logo.jsp、info.jsp、和info2.jsp,分别用JSP指令和动作将这三个文件插入zy.jsp页面,书写代码
在JSP页面中,你可以使用`<jsp:include>`标签或`<jsp:forward>`动作来嵌入其他JSP文件,并传递数据。假设你有一个名为`logo.jsp`, `info.jsp` 和 `info2.jsp` 的文件,下面是将它们包含到`zy.jsp`页面的示例代码:
```jsp
<!-- logo.jsp -->
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<!DOCTYPE html>
<html>
<head>
<title>Logo</title>
<img src="path_to_your_logo.jpg" alt="Company Logo">
</head>
<body>
<!-- Your logo content here -->
</body>
</html>
<!-- info.jsp -->
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<h1>公司地址:</h1>
<p>${company_address}</p>
<%-- 或者使用EL表达式或者其他合适的方式传递值 --%>
<!-- info2.jsp -->
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<h2>当前时间:</h2>
<p><%= new java.util.Date().toLocaleString() %></p>
<%-- 类似地,这里可以显示系统时间 --%>
<!-- zy.jsp -->
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<!DOCTYPE html>
<html>
<body>
<!-- 使用jsp:include包含logo -->
<jsp:include file="logo.jsp" />
<!-- 使用jsp:forward跳转并传递数据 -->
<%@ include file="info.jsp" %>
<!-- 如果需要在新的页面展示info2的内容,可以用forward -->
<%@ include file="info2.jsp" %>
</body>
</html>
```
在上述代码中,`<jsp:include>`用于静态内容的嵌套,如logo;`<%@ include file="..." %>`用于动态内容的引入,比如`info.jsp`中的公司地址信息。如果你想要在`info.jsp`之后展示新的页面而不刷新整个页面,可以使用`<jsp:forward>`。
请注意,你需要将`path_to_your_logo.jpg`替换为实际的logo图片路径,同时确保`company_address`变量在包含它的页面上已经设置过。如果需要从数据库或其他源获取这些信息,记得使用JSP的Bean或者EL表达式等机制进行数据绑定。
阅读全文
相关推荐
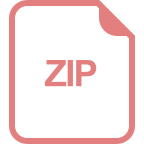
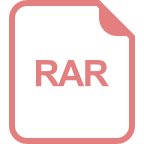
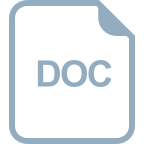
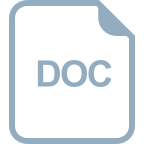
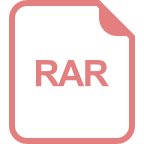
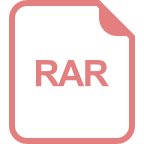
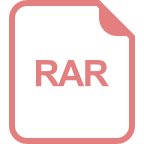
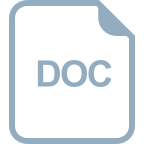
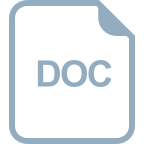
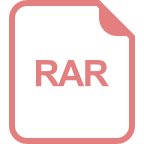
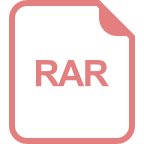
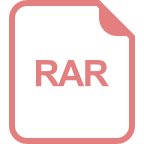
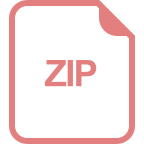