如何用Python实现一个请销假管理系统
时间: 2023-05-27 17:07:24 浏览: 206
请销假管理系统可以包含以下功能:
1. 登录系统:用户可以输入用户名和密码进行登录,登录成功后可以进入系统。
2. 请假申请:用户可以填写请假申请表,包括请假类型、开始时间、结束时间、请假原因等信息,提交后系统自动发送邮件给审批人。
3. 审批请假:审批人可以查看待审批的请假申请,可以通过或驳回申请,同时可以填写审批意见。
4. 查看请假记录:用户和管理员可以查看已经提交的请假记录,包括请假类型、开始时间、结束时间、请假原因、审批状态等信息。
5. 统计请假情况:管理员可以查看请假统计报表,包括请假类型、请假天数、请假人数等信息。
以下是一个简单的Python代码实现示例:
```python
import smtplib
from email.mime.text import MIMEText
class LeaveApplication:
def __init__(self, applicant, leave_type, start_date, end_date, reason):
self.applicant = applicant
self.leave_type = leave_type
self.start_date = start_date
self.end_date = end_date
self.reason = reason
def submit(self, approver):
# send email to approver
subject = f"Leave application from {self.applicant}"
content = f"Leave type: {self.leave_type}\n" \
f"Start date: {self.start_date}\n" \
f"End date: {self.end_date}\n" \
f"Reason: {self.reason}\n" \
f"Please approve or reject this application."
msg = MIMEText(content)
msg['Subject'] = subject
msg['From'] = 'your email address'
msg['To'] = approver
s = smtplib.SMTP('your email server address', 25)
s.login('your email username', 'your email password')
s.sendmail('your email address', [approver], msg.as_string())
s.quit()
class LeaveApproval:
def __init__(self, approver):
self.approver = approver
self.applications = []
def approve(self, application):
application.status = 'approved'
application.approver = self.approver
self.applications.append(application)
# send email to applicant
subject = f"Leave application approved by {self.approver}"
content = f"Leave type: {application.leave_type}\n" \
f"Start date: {application.start_date}\n" \
f"End date: {application.end_date}\n" \
f"Reason: {application.reason}\n" \
f"Approved by: {self.approver}"
msg = MIMEText(content)
msg['Subject'] = subject
msg['From'] = 'your email address'
msg['To'] = application.applicant
s = smtplib.SMTP('your email server address', 25)
s.login('your email username', 'your email password')
s.sendmail('your email address', [application.applicant], msg.as_string())
s.quit()
def reject(self, application):
application.status = 'rejected'
application.approver = self.approver
self.applications.append(application)
# send email to applicant
subject = f"Leave application rejected by {self.approver}"
content = f"Leave type: {application.leave_type}\n" \
f"Start date: {application.start_date}\n" \
f"End date: {application.end_date}\n" \
f"Reason: {application.reason}\n" \
f"Rejected by: {self.approver}"
msg = MIMEText(content)
msg['Subject'] = subject
msg['From'] = 'your email address'
msg['To'] = application.applicant
s = smtplib.SMTP('your email server address', 25)
s.login('your email username', 'your email password')
s.sendmail('your email address', [application.applicant], msg.as_string())
s.quit()
class LeaveRecord:
def __init__(self):
self.records = []
def add_record(self, application):
self.records.append(application)
def get_records(self):
return self.records
class LeaveReport:
def __init__(self, record):
self.record = record
def get_report(self):
report = {}
for application in self.record.get_records():
if application.status == 'approved':
leave_type = application.leave_type
days = (application.end_date - application.start_date).days + 1
if leave_type in report:
report[leave_type] += days
else:
report[leave_type] = days
return report
```
这是一个基于面向对象编程的实现示例,其中LeaveApplication类表示请假申请,LeaveApproval类表示请假审批,LeaveRecord类表示请假记录,LeaveReport类表示请假统计报表。
在实际应用中,可以根据需求进行修改和扩展。同时,需要注意安全性和合法性问题,例如用户登录验证、数据校验等。
阅读全文
相关推荐
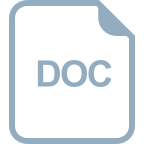
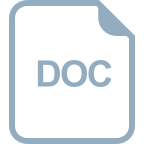
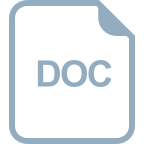


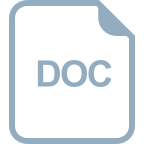
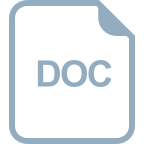
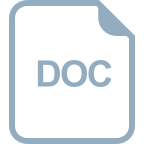

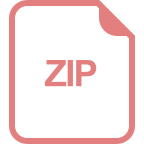
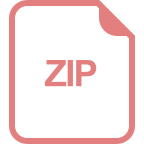
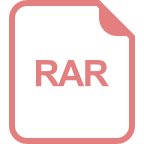
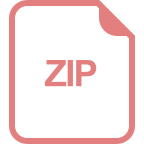
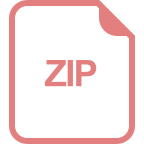
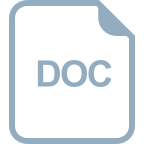
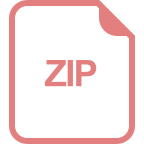
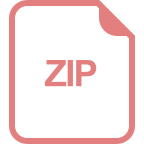
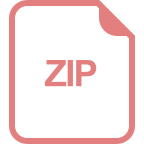
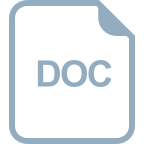