用C语言 A数组中存储500个2000以内的随机整数,完成以下操作: (1)对A数组中的元素进行直接插入排序,显示排序所用时间; (2)对A数组中的元素进行希尔排序,显示排序所用时间; (3)对A数组中的元素进行起泡排序,显示排序所用时间; (4)对A数组中的元素进行快速排序,显示排序所用时间。
时间: 2023-06-23 17:09:41 浏览: 82
以下是用C语言实现对A数组进行排序的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define MAX 500
#define LIMIT 2000
void swap(int *a, int *b){
int temp = *a;
*a = *b;
*b = temp;
}
void printArray(int arr[], int size){
for(int i=0; i<size; i++){
printf("%d ", arr[i]);
}
printf("\n");
}
void insertSort(int arr[], int size){
int i, j, temp;
clock_t start, end;
start = clock();
for(i=1; i<size; i++){
temp = arr[i];
j = i - 1;
while(j>=0 && arr[j]>temp){
arr[j+1] = arr[j];
j--;
}
arr[j+1] = temp;
}
end = clock();
printf("Insertion Sort: %f seconds\n", (double)(end-start)/CLOCKS_PER_SEC);
}
void shellSort(int arr[], int size){
int i, j, gap, temp;
clock_t start, end;
start = clock();
for(gap=size/2; gap>0; gap/=2){
for(i=gap; i<size; i++){
temp = arr[i];
for(j=i; j>=gap && arr[j-gap]>temp; j-=gap){
arr[j] = arr[j-gap];
}
arr[j] = temp;
}
}
end = clock();
printf("Shell Sort: %f seconds\n", (double)(end-start)/CLOCKS_PER_SEC);
}
void bubbleSort(int arr[], int size){
int i, j, flag=1;
clock_t start, end;
start = clock();
for(i=0; i<size-1 && flag==1; i++){
flag = 0;
for(j=0; j<size-i-1; j++){
if(arr[j]>arr[j+1]){
swap(&arr[j], &arr[j+1]);
flag = 1;
}
}
}
end = clock();
printf("Bubble Sort: %f seconds\n", (double)(end-start)/CLOCKS_PER_SEC);
}
int partition(int arr[], int low, int high){
int pivot = arr[high];
int i = low - 1;
for(int j=low; j<=high-1; j++){
if(arr[j]<=pivot){
i++;
swap(&arr[i], &arr[j]);
}
}
swap(&arr[i+1], &arr[high]);
return i+1;
}
void quickSort(int arr[], int low, int high){
if(low<high){
int pi = partition(arr, low, high);
quickSort(arr, low, pi-1);
quickSort(arr, pi+1, high);
}
}
void quickSortWrapper(int arr[], int size){
clock_t start, end;
start = clock();
quickSort(arr, 0, size-1);
end = clock();
printf("Quick Sort: %f seconds\n", (double)(end-start)/CLOCKS_PER_SEC);
}
int main(){
srand(time(NULL)); // 初始化随机数种子
int A[MAX];
for(int i=0; i<MAX; i++){
A[i] = rand() % LIMIT + 1;
}
printf("Original Array: ");
printArray(A, MAX);
insertSort(A, MAX);
printf("Insertion Sorted Array: ");
printArray(A, MAX);
shellSort(A, MAX);
printf("Shell Sorted Array: ");
printArray(A, MAX);
bubbleSort(A, MAX);
printf("Bubble Sorted Array: ");
printArray(A, MAX);
quickSortWrapper(A, MAX);
printf("Quick Sorted Array: ");
printArray(A, MAX);
return 0;
}
```
在此代码中,我们首先定义了数组的最大大小和随机数的范围。然后,我们使用 `srand()` 函数初始化随机数种子,并且使用 `rand()` 函数生成在指定范围内的随机整数来填充数组。接下来,我们分别实现了插入排序、希尔排序、冒泡排序和快速排序,并且在每个函数中使用 `clock()` 函数来计算排序所用的时间。最后,我们在 `main()` 函数中调用这些排序函数,并且在每个排序函数完成后输出排序所用的时间和排序后的数组。
注意,快速排序由于其递归的实现方式,我们需要将其封装在一个辅助函数中,以便于计算排序所用的时间。
阅读全文
相关推荐
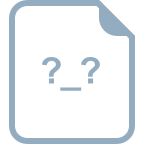
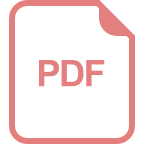
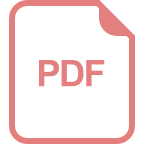
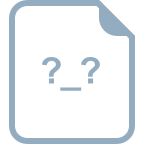
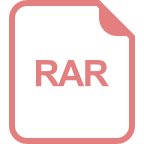
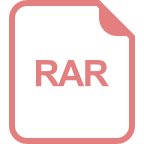
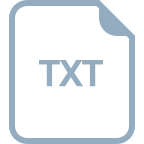
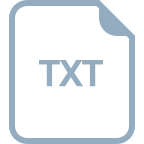
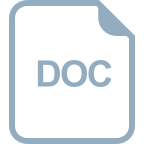
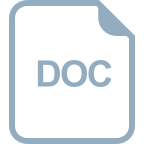


