android studio购物车价格计算
时间: 2023-10-04 18:05:17 浏览: 142
要实现购物车价格计算,你需要做以下步骤:
1. 创建商品类:创建一个商品类,包含商品名称、单价、数量等属性。
2. 创建购物车类:创建一个购物车类,包含添加商品、删除商品、清空购物车等方法。
3. 创建界面:创建一个界面,展示商品列表和购物车列表。
4. 实现添加商品:在商品列表中,添加商品到购物车。当添加商品时,将商品信息存储到购物车中,并且更新购物车列表。
5. 实现删除商品:在购物车列表中,删除不需要的商品。当删除商品时,将商品从购物车中移除,并且更新购物车列表。
6. 实现清空购物车:在购物车列表中,清空购物车。当清空购物车时,将购物车中的所有商品移除,并且更新购物车列表。
7. 实现价格计算:在购物车列表中,计算购物车的总价。当计算总价时,遍历购物车中的所有商品,累加单价和数量的乘积。
以上步骤可以通过使用 Java 和 Android Studio 实现。你可以参考以下代码片段:
商品类:
```
public class Product {
private String name;
private double price;
private int quantity;
public Product(String name, double price, int quantity) {
this.name = name;
this.price = price;
this.quantity = quantity;
}
public String getName() {
return name;
}
public double getPrice() {
return price;
}
public int getQuantity() {
return quantity;
}
}
```
购物车类:
```
public class ShoppingCart {
private List<Product> products = new ArrayList<>();
public void addProduct(Product product) {
products.add(product);
}
public void removeProduct(Product product) {
products.remove(product);
}
public void clearCart() {
products.clear();
}
public double getTotalPrice() {
double totalPrice = 0;
for (Product product : products) {
totalPrice += product.getPrice() * product.getQuantity();
}
return totalPrice;
}
public List<Product> getProducts() {
return products;
}
}
```
界面类:
```
public class MainActivity extends AppCompatActivity {
private List<Product> productList = new ArrayList<>();
private ShoppingCart shoppingCart = new ShoppingCart();
private ProductAdapter productAdapter;
private ShoppingCartAdapter shoppingCartAdapter;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// 初始化商品列表
productList.add(new Product("商品1", 10, 0));
productList.add(new Product("商品2", 20, 0));
productList.add(new Product("商品3", 30, 0));
// 初始化商品列表适配器
productAdapter = new ProductAdapter(productList, new ProductAdapter.OnItemClickListener() {
@Override
public void onItemClick(Product product) {
shoppingCart.addProduct(product);
shoppingCartAdapter.notifyDataSetChanged();
}
});
// 绑定商品列表适配器
RecyclerView productRecyclerView = findViewById(R.id.productRecyclerView);
productRecyclerView.setLayoutManager(new LinearLayoutManager(this));
productRecyclerView.setAdapter(productAdapter);
// 初始化购物车适配器
shoppingCartAdapter = new ShoppingCartAdapter(shoppingCart.getProducts(), new ShoppingCartAdapter.OnItemClickListener() {
@Override
public void onItemClick(Product product) {
shoppingCart.removeProduct(product);
shoppingCartAdapter.notifyDataSetChanged();
}
});
// 绑定购物车适配器
RecyclerView shoppingCartRecyclerView = findViewById(R.id.shoppingCartRecyclerView);
shoppingCartRecyclerView.setLayoutManager(new LinearLayoutManager(this));
shoppingCartRecyclerView.setAdapter(shoppingCartAdapter);
// 绑定清空购物车按钮点击事件
Button clearCartButton = findViewById(R.id.clearCartButton);
clearCartButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
shoppingCart.clearCart();
shoppingCartAdapter.notifyDataSetChanged();
}
});
// 绑定计算总价按钮点击事件
Button calculateButton = findViewById(R.id.calculateButton);
calculateButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
double totalPrice = shoppingCart.getTotalPrice();
Toast.makeText(MainActivity.this, "总价:" + totalPrice, Toast.LENGTH_SHORT).show();
}
});
}
// 商品列表适配器
private static class ProductAdapter extends RecyclerView.Adapter<ProductViewHolder> {
private List<Product> productList;
private OnItemClickListener listener;
public ProductAdapter(List<Product> productList, OnItemClickListener listener) {
this.productList = productList;
this.listener = listener;
}
@NonNull
@Override
public ProductViewHolder onCreateViewHolder(@NonNull ViewGroup parent, int viewType) {
View view = LayoutInflater.from(parent.getContext()).inflate(R.layout.item_product, parent, false);
return new ProductViewHolder(view);
}
@Override
public void onBindViewHolder(@NonNull ProductViewHolder holder, int position) {
Product product = productList.get(position);
holder.nameTextView.setText(product.getName());
holder.priceTextView.setText(String.valueOf(product.getPrice()));
holder.quantityTextView.setText(String.valueOf(product.getQuantity()));
holder.itemView.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
listener.onItemClick(product);
}
});
}
@Override
public int getItemCount() {
return productList.size();
}
public interface OnItemClickListener {
void onItemClick(Product product);
}
}
// 商品列表ViewHolder
private static class ProductViewHolder extends RecyclerView.ViewHolder {
private TextView nameTextView;
private TextView priceTextView;
private TextView quantityTextView;
public ProductViewHolder(@NonNull View itemView) {
super(itemView);
nameTextView = itemView.findViewById(R.id.nameTextView);
priceTextView = itemView.findViewById(R.id.priceTextView);
quantityTextView = itemView.findViewById(R.id.quantityTextView);
}
}
// 购物车列表适配器
private static class ShoppingCartAdapter extends RecyclerView.Adapter<ShoppingCartViewHolder> {
private List<Product> productList;
private OnItemClickListener listener;
public ShoppingCartAdapter(List<Product> productList, OnItemClickListener listener) {
this.productList = productList;
this.listener = listener;
}
@NonNull
@Override
public ShoppingCartViewHolder onCreateViewHolder(@NonNull ViewGroup parent, int viewType) {
View view = LayoutInflater.from(parent.getContext()).inflate(R.layout.item_shopping_cart, parent, false);
return new ShoppingCartViewHolder(view);
}
@Override
public void onBindViewHolder(@NonNull ShoppingCartViewHolder holder, int position) {
Product product = productList.get(position);
holder.nameTextView.setText(product.getName());
holder.priceTextView.setText(String.valueOf(product.getPrice()));
holder.quantityTextView.setText(String.valueOf(product.getQuantity()));
holder.itemView.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
listener.onItemClick(product);
}
});
}
@Override
public int getItemCount() {
return productList.size();
}
public interface OnItemClickListener {
void onItemClick(Product product);
}
}
// 购物车列表ViewHolder
private static class ShoppingCartViewHolder extends RecyclerView.ViewHolder {
private TextView nameTextView;
private TextView priceTextView;
private TextView quantityTextView;
public ShoppingCartViewHolder(@NonNull View itemView) {
super(itemView);
nameTextView = itemView.findViewById(R.id.nameTextView);
priceTextView = itemView.findViewById(R.id.priceTextView);
quantityTextView = itemView.findViewById(R.id.quantityTextView);
}
}
}
```
对应的布局文件:
activity_main.xml
```
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="horizontal"
android:layout_width="match_parent"
android:layout_height="match_parent">
<LinearLayout
android:orientation="vertical"
android:layout_width="0dp"
android:layout_weight="1"
android:layout_height="match_parent">
<TextView
android:text="商品列表"
android:textSize="20sp"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
<androidx.recyclerview.widget.RecyclerView
android:id="@+id/productRecyclerView"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1"/>
</LinearLayout>
<LinearLayout
android:orientation="vertical"
android:layout_width="0dp"
android:layout_weight="1"
android:layout_height="match_parent">
<TextView
android:text="购物车"
android:textSize="20sp"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
<androidx.recyclerview.widget.RecyclerView
android:id="@+id/shoppingCartRecyclerView"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1"/>
<LinearLayout
android:orientation="horizontal"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<Button
android:id="@+id/clearCartButton"
android:text="清空购物车"
android:layout_width="0dp"
android:layout_weight="1"
android:layout_height="wrap_content"/>
<Button
android:id="@+id/calculateButton"
android:text="计算总价"
android:layout_width="0dp"
android:layout_weight="1"
android:layout_height="wrap_content"/>
</LinearLayout>
</LinearLayout>
</LinearLayout>
```
item_product.xml
```
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="horizontal"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:padding="16dp">
<TextView
android:id="@+id/nameTextView"
android:textSize="20sp"
android:layout_width="0dp"
android:layout_weight="1"
android:layout_height="wrap_content"/>
<TextView
android:id="@+id/priceTextView"
android:textSize="20sp"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
<TextView
android:id="@+id/quantityTextView"
android:textSize="20sp"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
</LinearLayout>
```
item_shopping_cart.xml
```
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="horizontal"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:padding="16dp">
<TextView
android:id="@+id/nameTextView"
android:textSize="20sp"
android:layout_width="0dp"
android:layout_weight="1"
android:layout_height="wrap_content"/>
<TextView
android:id="@+id/priceTextView"
android:textSize="20sp"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
<TextView
android:id="@+id/quantityTextView"
android:textSize="20sp"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
</LinearLayout>
```
上述代码是一个简单的购物车价格计算实现,你可以根据自己的需求进行修改和扩展。
阅读全文
相关推荐
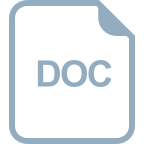
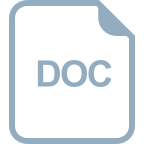
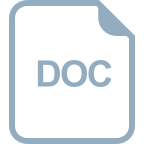
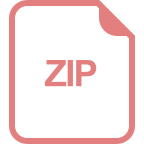














