map的entrySet导致stackOverFlow
时间: 2023-08-01 18:09:12 浏览: 48
`entrySet()`方法是Java中Map接口的一个方法,它返回一个`Set`集合,包含了Map中所有的键值对。在Java中,由于`entrySet()`方法返回的是一个`Set`集合,因此遍历`entrySet()`也是使用迭代器或者增强for循环进行遍历的,不会导致stack overflow的问题。
如果你在使用`entrySet()`方法时遇到了stack overflow的问题,可能是由于在遍历时使用了递归的方式,导致了stack overflow的错误。在遍历`entrySet()`时,应该使用迭代器或者增强for循环,而不是递归调用。例如:
```
Map<String, String> map = new HashMap<String, String>();
// 添加键值对到map中
...
// 遍历map中的键值对
for (Map.Entry<String, String> entry : map.entrySet()) {
String key = entry.getKey();
String value = entry.getValue();
// 处理键值对
}
```
在上面的代码中,使用了增强for循环来遍历`entrySet()`方法返回的Set集合,从而避免了递归调用导致的stack overflow问题。
相关问题
map的entrySet导致了stackOverFlow,该怎么解决
如果在使用`entrySet()`方法遍历Map时,出现了stack overflow的问题,可能是由于在遍历Map时使用了递归的方式,导致了stack overflow错误。解决这个问题的方法是改用迭代方法来遍历,或者使用非递归的遍历方法。
以下是使用迭代方法来遍历Map的示例代码:
```
Map<String, String> map = new HashMap<String, String>();
// 添加键值对到map中
...
// 遍历map中的键值对
Iterator<Map.Entry<String, String>> iterator = map.entrySet().iterator();
while (iterator.hasNext()) {
Map.Entry<String, String> entry = iterator.next();
String key = entry.getKey();
String value = entry.getValue();
// 处理键值对
}
```
以上代码使用了迭代器来遍历Map中的键值对,从而避免了递归调用导致的stack overflow问题。
另外,也可以使用Java8中的Lambda表达式来遍历Map中的键值对,示例代码如下:
```
Map<String, String> map = new HashMap<String, String>();
// 添加键值对到map中
...
// 遍历map中的键值对
map.forEach((key, value) -> {
// 处理键值对
});
```
这种方式也不会导致stack overflow的问题。
Collections.synchronizedMap entrySet ConcurrentModificationException
使用`Collections.synchronizedMap`方法创建的同步Map,可以确保多线程环境下的安全访问。但是如果在迭代Map的`entrySet()`时,其他线程对Map进行了修改,就会抛出`ConcurrentModificationException`异常。
这是因为`entrySet()`方法返回的是一个视图,它与原始Map相关联。当原始Map被修改时,视图也会相应地发生变化。如果在迭代过程中,其他线程对原始Map进行了增删操作,就会导致迭代器检测到不一致的修改,从而抛出异常。
为了避免这个问题,可以在迭代过程中使用`synchronized`关键字对Map进行锁定,或者使用`synchronizedMap`方法返回的Map对象进行迭代操作。这样可以确保在迭代期间其他线程无法修改Map,从而避免`ConcurrentModificationException`异常的发生。
相关推荐
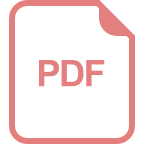












