spring boot实现登录
时间: 2023-05-08 09:58:38 浏览: 66
Spring Boot是一个基于Spring Framework的快速开发框架,并提供了许多开箱即用的功能。其中之一就是安全性,它提供了一种简单但有效的方式实现用户身份认证和授权。
首先,我们需要在pom.xml文件中添加security依赖项。在创建一个基于Spring Boot的Web应用程序时,可以使用Spring Boot的Spring Security Starter来添加所需的依赖关系。这可以通过在pom.xml文件中添加以下依赖项来完成:
```
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
```
一旦添加了这个依赖项,我们就可以在应用程序中启用安全相关功能了。Spring Boot提供了一种嵌入式Web服务器,使得启用安全功能非常方便。只需在应用程序主类的注释中添加@EnableWebSecurity注释即可启用安全功能:
```
@SpringBootApplication
@EnableWebSecurity
public class MyApp {
// ...
}
```
现在我们需要定义一个认证机制,将用户名称和密码与数据库中存储的凭据进行比较。Spring Security接口有一个UserDetailsService接口,我们需要实现它来检索有关用户的详细信息。我们可以将该实现与数据库集成,以检索存储在数据库中的用户详细信息。以下是UserDetailsService接口的示例实现:
```
@Service
public class UserDetailsServiceImpl implements UserDetailsService {
@Autowired
private UserRepository userRepository;
@Override
public UserDetails loadUserByUsername(String username) throws UsernameNotFoundException {
User user = userRepository.findByUsername(username);
if (user == null) {
throw new UsernameNotFoundException("User not found");
}
return new org.springframework.security.core.userdetails.User(user.getUsername(), user.getPassword(),
AuthorityUtils.createAuthorityList("ROLE_USER"));
}
}
```
接下来,我们需要编写一个安全配置,其中包括:
- 将我们的UserDetailsService实现添加到身份验证管理器
- 配置身份验证请求
- 配置基于表单的身份验证
以下是一个示例安全配置的实现:
```
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private UserDetailsService userDetailsService;
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/").permitAll()
.antMatchers("/login").permitAll()
.antMatchers("/admin/**").hasRole("ADMIN")
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login")
.defaultSuccessUrl("/")
.failureUrl("/login?error=true")
.permitAll()
.and()
.logout()
.logoutSuccessUrl("/login")
.permitAll();
}
@Autowired
public void configureGlobal(AuthenticationManagerBuilder auth) throws Exception {
auth.userDetailsService(userDetailsService)
.passwordEncoder(passwordEncoder());
}
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
}
```
最后,我们需要创建一个登录表单。为此,我们将创建一个名为“login.html”的页面,并将其放置在src/main/resources/templates目录下。以下是一个示例页面:
```
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8" />
<title>Login</title>
</head>
<body>
<form id="login" th:action="@{/login}" method="post">
<div>
<label for="username">Username:</label>
<input type="text" id="username" name="username" />
</div>
<div>
<label for="password">Password:</label>
<input type="password" id="password" name="password" />
</div>
<button type="submit">Login</button>
</form>
</body>
</html>
```
完成以上步骤后,您现在应该拥有完整的基于Spring Boot的Web应用程序,该应用程序实现了基本的登录认证机制。用户将能够使用凭据登录系统,并根据其角色获得所需的访问权限。
相关推荐
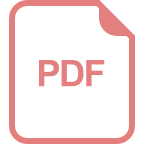















